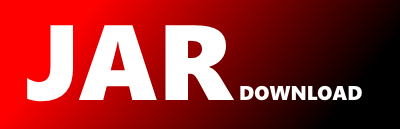
com.aoindustries.aoserv.client.MasterProcess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2013, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.aoserv.client.validator.InetAddress;
import com.aoindustries.aoserv.client.validator.ValidationException;
import com.aoindustries.io.CompressedDataInputStream;
import com.aoindustries.io.CompressedDataOutputStream;
import com.aoindustries.util.InternUtils;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Timestamp;
/**
* Each Thread
on the master reports its activities so that
* a query on this table shows a snapshot of the currently running system.
*
* @author AO Industries, Inc.
*/
final public class MasterProcess extends AOServObject implements SingleTableObject {
private static boolean logCommands=false;
/**
* Turns on/off command logging.
*/
public static void setLogCommands(boolean state) {
logCommands=state;
}
public static boolean getLogCommands() {
return logCommands;
}
/**
* The different states a process may be in.
*/
public static final String
LOGIN="login",
RUN="run",
SLEEP="sleep"
;
long process_id;
private long connector_id=-1;
private String authenticated_user;
private String effective_user;
private int daemon_server;
private InetAddress host;
private String protocol;
private String aoserv_protocol;
private boolean is_secure;
private long connect_time;
private long use_count;
private long total_time;
private int priority;
private String state;
private Object[] command;
private long state_start_time;
private AOServTable table;
public MasterProcess() {
}
public MasterProcess(
long process_id,
InetAddress host,
String protocol,
boolean is_secure,
long connect_time
) {
this.process_id=process_id;
this.host=host;
this.protocol=protocol;
this.is_secure=is_secure;
this.connect_time=connect_time;
this.priority=Thread.NORM_PRIORITY;
this.state=LOGIN;
this.state_start_time=connect_time;
}
synchronized public void commandCompleted() {
long time=System.currentTimeMillis();
total_time+=time-state_start_time;
state=SLEEP;
command=null;
state_start_time=time;
}
synchronized public void commandRunning() {
use_count++;
state=RUN;
state_start_time=System.currentTimeMillis();
}
synchronized public void commandSleeping() {
if(!state.equals(SLEEP)) {
long time=System.currentTimeMillis();
state=SLEEP;
total_time+=time-state_start_time;
state_start_time=time;
}
}
@Override
Object getColumnImpl(int i) {
switch(i) {
case 0: return process_id;
case 1: return connector_id == -1 ? null : connector_id;
case 2: return authenticated_user;
case 3: return effective_user;
case 4: return daemon_server == -1 ? null : daemon_server;
case 5: return host;
case 6: return protocol;
case 7: return aoserv_protocol;
case 8: return is_secure;
case 9: return getConnectTime();
case 10: return use_count;
case 11: return total_time;
case 12: return priority;
case 13: return state;
case 14: return getCommand();
case 15: return getStateStartTime();
default: throw new IllegalArgumentException("Invalid index: "+i);
}
}
public int getDaemonServer() {
return daemon_server;
}
public int getPriority() {
return priority;
}
public long getProcessID() {
return process_id;
}
public long getConnectorID() {
return connector_id;
}
public String getAuthenticatedUser() {
return authenticated_user;
}
public BusinessAdministrator getAuthenticatedBusinessAdministrator() throws IOException, SQLException {
// Null OK when filtered
return table.connector.getBusinessAdministrators().get(authenticated_user);
}
public String getEffectiveUser() {
return effective_user;
}
public BusinessAdministrator getEffectiveBusinessAdministrator() throws SQLException, IOException {
BusinessAdministrator ba=table.connector.getBusinessAdministrators().get(effective_user);
if(ba==null) throw new SQLException("Unable to find BusinessAdministrator: "+effective_user);
return ba;
}
public InetAddress getHost() {
return host;
}
public String getProtocol() {
return protocol;
}
public String getAOServProtocol() {
return aoserv_protocol;
}
public void setAOServProtocol(String aoserv_protocol) {
this.aoserv_protocol=aoserv_protocol;
}
public boolean isSecure() {
return is_secure;
}
public Timestamp getConnectTime() {
return new Timestamp(connect_time);
}
public long getUseCount() {
return use_count;
}
public long getTotalTime() {
return total_time;
}
public String getState() {
return state;
}
public Timestamp getStateStartTime() {
return new Timestamp(state_start_time);
}
@Override
public Long getKey() {
return process_id;
}
/**
* Gets the AOServTable
that contains this AOServObject
.
*
* @return the AOServTable
.
*/
@Override
final public AOServTable getTable() {
return table;
}
@Override
public SchemaTable.TableID getTableID() {
return SchemaTable.TableID.MASTER_PROCESSES;
}
@Override
public void init(ResultSet result) throws SQLException {
throw new SQLException("Should not be read from the database, should be generated.");
}
@Override
public void read(CompressedDataInputStream in) throws IOException {
try {
process_id=in.readLong();
connector_id=in.readLong();
authenticated_user=InternUtils.intern(in.readNullUTF());
effective_user=InternUtils.intern(in.readNullUTF());
daemon_server=in.readCompressedInt();
host=InetAddress.valueOf(in.readUTF()).intern();
protocol=in.readUTF().intern();
aoserv_protocol=InternUtils.intern(in.readNullUTF());
is_secure=in.readBoolean();
connect_time=in.readLong();
use_count=in.readLong();
total_time=in.readLong();
priority=in.readCompressedInt();
state=in.readUTF().intern();
if(in.readBoolean()) {
command=new Object[] {in.readUTF()};
} else {
command=null;
}
state_start_time=in.readLong();
} catch(ValidationException e) {
throw new IOException(e);
}
}
synchronized public void setCommand(Object ... command) {
if(command==null) this.command=null;
else {
this.command=command;
}
}
synchronized public String getCommand() {
if(command==null) return null;
StringBuilder SB=new StringBuilder();
int len=command.length;
for(int c=0;c0) SB.append(' ');
Object com=command[c];
if(com==null) SB.append("''");
else if(com instanceof Object[]) {
Object[] oa=(Object[])com;
int oaLen=oa.length;
for(int d=0;d0) SB.append(' ');
Object com2=oa[d];
if(com2==null) SB.append("''");
else SB.append(com2);
}
} else SB.append(com);
}
return SB.toString();
}
public void setAuthenticatedUser(String username) {
authenticated_user=username;
}
public void setConnectorID(long id) {
connector_id=id;
}
public void setDeamonServer(int server) {
daemon_server=server;
}
public void setEffectiveUser(String username) {
effective_user=username;
}
public void setPriority(int priority) {
this.priority=priority;
}
@Override
public void setTable(AOServTable table) {
if(this.table!=null) throw new IllegalStateException("table already set");
this.table=table;
}
@Override
String toStringImpl() {
return getCommand();
}
@Override
public void write(CompressedDataOutputStream out, AOServProtocol.Version version) throws IOException {
out.writeLong(process_id);
out.writeLong(connector_id);
out.writeNullUTF(authenticated_user);
out.writeNullUTF(effective_user);
out.writeCompressedInt(daemon_server);
out.writeUTF(host.toString());
out.writeUTF(protocol);
if(version.compareTo(AOServProtocol.Version.VERSION_1_0_A_101)>=0) out.writeNullUTF(aoserv_protocol);
out.writeBoolean(is_secure);
out.writeLong(connect_time);
out.writeLong(use_count);
out.writeLong(total_time);
out.writeCompressedInt(priority);
out.writeUTF(state);
String myCommand=getCommand();
out.writeBoolean(myCommand!=null);
if(myCommand!=null) out.writeUTF(myCommand);
out.writeLong(state_start_time);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy