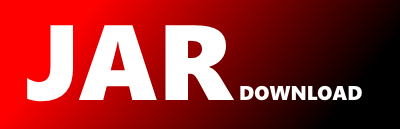
com.aoindustries.aoserv.client.PostgresDatabase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2000-2013, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.io.CompressedDataInputStream;
import com.aoindustries.io.CompressedDataOutputStream;
import com.aoindustries.util.BufferManager;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.Writer;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* A PostgresDatabase
corresponds to a unique PostgreSQL table
* space on one server. The database name must be unique per server
* and, to aid in account portability, will typically be unique
* across the entire system.
*
* @see PostgresEncoding
* @see PostgresServerUser
*
* @author AO Industries, Inc.
*/
final public class PostgresDatabase extends CachedObjectIntegerKey implements Dumpable, Removable, JdbcProvider {
static final int
COLUMN_PKEY=0,
COLUMN_POSTGRES_SERVER=2,
COLUMN_DATDBA=3
;
static final String COLUMN_NAME_name = "name";
static final String COLUMN_POSTGRES_SERVER_name = "postgres_server";
/**
* The classname of the JDBC driver used for the PostgresDatabase
.
*/
public static final String JDBC_DRIVER="org.postgresql.Driver";
/**
* Special databases.
*/
public static final String
AOINDUSTRIES="aoindustries",
AOSERV="aoserv",
AOWEB="aoweb",
TEMPLATE0="template0",
TEMPLATE1="template1"
;
/**
* The name of a database is limited by the internal data type of
* the pg_database
table. The type is name
* which has a maximum length of 31 characters.
*/
public static final int MAX_DATABASE_NAME_LENGTH=31;
String name;
int postgres_server;
int datdba;
private int encoding;
private boolean is_template;
private boolean allow_conn;
private boolean enable_postgis;
public boolean allowsConnections() {
return allow_conn;
}
@Override
public void dump(PrintWriter out) throws IOException, SQLException {
dump((Writer)out);
}
public void dump(final Writer out) throws IOException, SQLException {
table.connector.requestUpdate(
false,
new AOServConnector.UpdateRequest() {
@Override
public void writeRequest(CompressedDataOutputStream masterOut) throws IOException {
masterOut.writeCompressedInt(AOServProtocol.CommandID.DUMP_POSTGRES_DATABASE.ordinal());
masterOut.writeCompressedInt(pkey);
}
@Override
public void readResponse(CompressedDataInputStream masterIn) throws IOException, SQLException {
int code;
byte[] buff=BufferManager.getBytes();
try {
char[] chars=BufferManager.getChars();
try {
while((code=masterIn.readByte())==AOServProtocol.NEXT) {
int len=masterIn.readShort();
masterIn.readFully(buff, 0, len);
for(int c=0;c getCannotRemoveReasons() throws SQLException, IOException {
List reasons=new ArrayList<>();
PostgresServer ps=getPostgresServer();
if(!allow_conn) reasons.add(new CannotRemoveReason<>("Not allowed to drop a PostgreSQL database that does not allow connections: "+name+" on "+ps.getName()+" on "+ps.getAOServer().getHostname(), this));
if(is_template) reasons.add(new CannotRemoveReason<>("Not allowed to drop a template PostgreSQL database: "+name+" on "+ps.getName()+" on "+ps.getAOServer().getHostname(), this));
if(
name.equals(AOINDUSTRIES)
|| name.equals(AOSERV)
|| name.equals(AOWEB)
) reasons.add(new CannotRemoveReason<>("Not allowed to drop a special PostgreSQL database: "+name+" on "+ps.getName()+" on "+ps.getAOServer().getHostname(), this));
return reasons;
}
@Override
public void remove() throws IOException, SQLException {
table.connector.requestUpdateIL(
true,
AOServProtocol.CommandID.REMOVE,
SchemaTable.TableID.POSTGRES_DATABASES,
pkey
);
}
@Override
String toStringImpl() {
return name;
}
@Override
public void write(CompressedDataOutputStream out, AOServProtocol.Version protocolVersion) throws IOException {
out.writeCompressedInt(pkey);
out.writeUTF(name);
out.writeCompressedInt(postgres_server);
out.writeCompressedInt(datdba);
out.writeCompressedInt(encoding);
out.writeBoolean(is_template);
out.writeBoolean(allow_conn);
if(protocolVersion.compareTo(AOServProtocol.Version.VERSION_1_30)<=0) {
out.writeShort(0);
out.writeShort(7);
}
if(protocolVersion.compareTo(AOServProtocol.Version.VERSION_1_27)>=0) out.writeBoolean(enable_postgis);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy