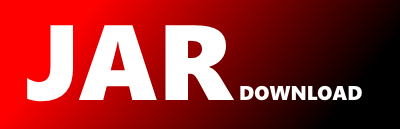
com.aoindustries.aoserv.client.SSLConnector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2012, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.io.AOPool;
import com.aoindustries.lang.ObjectUtils;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Logger;
import javax.net.ssl.SSLSocketFactory;
/**
* A SSLConnector
provides the connection between
* the client and server over secured SSL sockets.
*
* @see AOServConnector
*
* @author AO Industries, Inc.
*/
public class SSLConnector extends TCPConnector {
/**
* The trust store used for this connector.
*/
private static String trustStorePath;
/**
* The password for the trust store.
*/
private static String trustStorePassword;
/**
* The protocol for this connector.
*/
public static final String PROTOCOL="ssl";
/**
* Instances of connectors are created once and then reused.
*/
private static final List connectors=new ArrayList<>();
protected SSLConnector(
String hostname,
String local_ip,
int port,
String connectAs,
String authenticateAs,
String password,
String daemonServer,
int poolSize,
long maxConnectionAge,
String trustStorePath,
String trustStorePassword,
Logger logger
) throws IOException {
super(hostname, local_ip, port, connectAs, authenticateAs, password, daemonServer, poolSize, maxConnectionAge, logger);
if(
(
SSLConnector.trustStorePath!=null
&& !SSLConnector.trustStorePath.equals(trustStorePath)
) || (
SSLConnector.trustStorePassword!=null
&& !SSLConnector.trustStorePassword.equals(trustStorePassword)
)
) throw new IllegalArgumentException(
"Trust store path and password may only be set once, currently '"
+ SSLConnector.trustStorePath
+ "', trying to set to '"
+ trustStorePath
+ "'"
);
if(SSLConnector.trustStorePath==null) {
SSLConnector.trustStorePath=trustStorePath;
SSLConnector.trustStorePassword=trustStorePassword;
}
}
@Override
public String getProtocol() {
return PROTOCOL;
}
@Override
Socket getSocket() throws IOException {
if(trustStorePath!=null && trustStorePath.length()>0) {
System.setProperty("javax.net.ssl.trustStore", trustStorePath);
}
if(trustStorePassword!=null && trustStorePassword.length()>0) {
System.setProperty("javax.net.ssl.trustStorePassword", trustStorePassword);
}
SSLSocketFactory sslFact=(SSLSocketFactory)SSLSocketFactory.getDefault();
Socket regSocket = new Socket();
if(local_ip!=null) regSocket.bind(new InetSocketAddress(local_ip, 0));
regSocket.connect(new InetSocketAddress(hostname, port), AOPool.DEFAULT_CONNECT_TIMEOUT);
regSocket.setKeepAlive(true);
regSocket.setSoLinger(true, AOPool.DEFAULT_SOCKET_SO_LINGER);
//regSocket.setTcpNoDelay(true);
return sslFact.createSocket(regSocket, hostname, port, true);
}
public static synchronized SSLConnector getSSLConnector(
String hostname,
String local_ip,
int port,
String connectAs,
String authenticateAs,
String password,
String daemonServer,
int poolSize,
long maxConnectionAge,
String trustStorePath,
String trustStorePassword,
Logger logger
) throws IOException {
if(connectAs==null) throw new NullPointerException("connectAs is null");
if(authenticateAs==null) throw new NullPointerException("authenticateAs is null");
if(password==null) throw new NullPointerException("password is null");
int size=connectors.size();
for(int c=0;c
© 2015 - 2024 Weber Informatics LLC | Privacy Policy