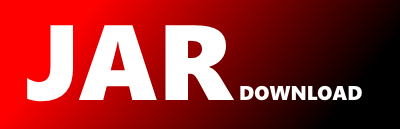
com.aoindustries.aoserv.client.SocketConnectionPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2009, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.io.AOPool;
import com.aoindustries.util.EncodingUtils;
import java.io.IOException;
import java.io.InterruptedIOException;
import java.sql.SQLException;
import java.util.logging.Logger;
/**
* Connections made by TCPConnector
or any
* of its derivatives are pooled and reused.
*
* @see TCPConnector
*
* @author AO Industries, Inc.
*/
final class SocketConnectionPool extends AOPool {
public static final int DELAY_TIME=3*60*1000;
public static final int MAX_IDLE_TIME=15*60*1000;
private final TCPConnector connector;
SocketConnectionPool(TCPConnector connector, Logger logger) {
super(DELAY_TIME, MAX_IDLE_TIME, SocketConnectionPool.class.getName()+"?hostname=" + connector.hostname+"&port="+connector.port+"&connectAs="+connector.connectAs+"&authenticateAs="+connector.authenticateAs, connector.poolSize, connector.maxConnectionAge, logger);
this.connector=connector;
}
@Override
protected void close(SocketConnection conn) {
conn.close();
}
@Override
protected SocketConnection getConnectionObject() throws InterruptedIOException, IOException {
return new SocketConnection(connector);
}
@Override
protected boolean isClosed(SocketConnection conn) {
return conn.isClosed();
}
/**
* Avoid repeated copies.
*/
private static final int numTables = SchemaTable.TableID.values().length;
@Override
protected void printConnectionStats(Appendable out) throws IOException {
try {
// Create statistics on the caches
int totalLoaded=0;
int totalCaches=0;
int totalActive=0;
int totalHashed=0;
int totalIndexed=0;
int totalRows=0;
for(AOServTable table : connector.tables) {
totalLoaded++;
if(table instanceof CachedTable) {
totalCaches++;
int columnCount=table.getTableSchema().getSchemaColumns(connector).size();
CachedTable cached=(CachedTable)table;
if(cached.isLoaded()) {
totalActive++;
for(int d=0;dAOServ Tables \n"
+ " Total Tables: ").append(Integer.toString(numTables)).append(" \n"
+ " Loaded: ").append(Integer.toString(totalLoaded)).append(" \n"
+ " Caches: ").append(Integer.toString(totalCaches)).append(" \n"
+ " Active: ").append(Integer.toString(totalActive)).append(" \n"
+ " Hashed: ").append(Integer.toString(totalHashed)).append(" \n"
+ " Indexes: ").append(Integer.toString(totalIndexed)).append(" \n"
+ " Total Rows: ").append(Integer.toString(totalRows)).append(" \n"
+ "\n"
+ "
\n"
+ "\n"
+ " TCP Connection Pool \n"
+ " Host: ");
EncodingUtils.encodeHtml(connector.hostname, out);
out.append(" \n"
+ " Port: ").append(Integer.toString(connector.port)).append(" \n"
+ " Connected As: ");
EncodingUtils.encodeHtml(connector.connectAs, out);
out.append(" \n"
+ " Authenticated As: ");
EncodingUtils.encodeHtml(connector.authenticateAs, out);
out.append(" \n"
+ " Password: ");
String password=connector.password;
int len=password.length();
for(int c=0;c \n");
} catch(SQLException err) {
throw new IOException(err);
}
}
@Override
protected void resetConnection(SocketConnection conn) {
}
@Override
protected IOException newException(String message, Throwable cause) {
IOException err=new IOException(message);
if(cause!=null) err.initCause(cause);
return err;
}
@Override
protected InterruptedIOException newInterruptedException(String message, Throwable cause) {
InterruptedIOException err = new InterruptedIOException(message);
if(cause!=null) err.initCause(cause);
return err;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy