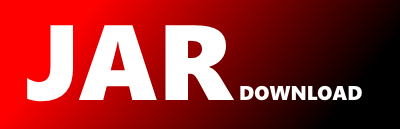
com.aoindustries.aoserv.client.TCPConnector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2012, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import static com.aoindustries.aoserv.client.ApplicationResources.accessor;
import com.aoindustries.io.AOPool;
import com.aoindustries.io.CompressedDataInputStream;
import com.aoindustries.io.CompressedDataOutputStream;
import com.aoindustries.lang.ObjectUtils;
import com.aoindustries.util.IntArrayList;
import com.aoindustries.util.IntList;
import java.io.EOFException;
import java.io.IOException;
import java.io.InterruptedIOException;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.SwingUtilities;
/**
* A TCPConnector
provides the connection between
* the object layer and the data over a pool of un-secured sockets.
*
* @see SocketConnection
*
* @author AO Industries, Inc.
*/
public class TCPConnector extends AOServConnector {
/**
* Close cache monitor after 90 minutes of inactivity.
*/
private static final long MAX_IDLE_LISTEN_CACHES = 90L*60*1000;
/** Avoid repeated copies using static final int. */
private static final int numTables = SchemaTable.TableID.values().length;
class CacheMonitor extends Thread {
CacheMonitor() {
super("TCPConnector - CacheMonitor");
setDaemon(true);
start();
}
@Override
public void run() {
try {
//System.err.println("DEBUG: TCPConnector("+connectAs+"-"+getConnectorID()+").CacheMonitor: run: Starting");
boolean runMore=true;
while(runMore) {
try {
AOServConnection conn=getConnection(1);
try {
try {
//System.err.println("DEBUG: TCPConnector("+connectAs+"-"+getConnectorID()+").CacheMonitor: run: conn.identityHashCode="+System.identityHashCode(conn));
CompressedDataOutputStream out = conn.getOutputStream();
out.writeCompressedInt(AOServProtocol.CommandID.LISTEN_CACHES.ordinal());
out.flush();
CompressedDataInputStream in=conn.getInputStream();
IntList tableList=new IntArrayList();
while(runMore) {
synchronized(cacheMonitorLock) {
long currentTime=System.currentTimeMillis();
long timeSince=currentTime-connectionLastUsed;
if(timeSince<0) connectionLastUsed=currentTime;
else if(timeSince>=MAX_IDLE_LISTEN_CACHES) {
// Must also not have any invalidate listeners
boolean foundListener=false;
for(int c=0;c connectors=new ArrayList<>();
/**
* The maximum size of the connection pool.
*/
final int poolSize;
final long maxConnectionAge;
private static class CacheMonitorLock {}
final private CacheMonitorLock cacheMonitorLock=new CacheMonitorLock();
private long connectionLastUsed;
private CacheMonitor cacheMonitor;
protected TCPConnector(
String hostname,
String local_ip,
int port,
String connectAs,
String authenticateAs,
String password,
String daemonServer,
int poolSize,
long maxConnectionAge,
Logger logger
) throws IOException {
super(hostname, local_ip, port, connectAs, authenticateAs, password, daemonServer, logger);
this.poolSize=poolSize;
this.maxConnectionAge=maxConnectionAge;
this.pool=new SocketConnectionPool(this, logger);
}
private void startCacheMonitor() {
synchronized(cacheMonitorLock) {
connectionLastUsed=System.currentTimeMillis();
if(cacheMonitor==null) cacheMonitor=new CacheMonitor();
}
}
@Override
protected final AOServConnection getConnection(int maxConnections) throws InterruptedIOException, IOException {
if(SwingUtilities.isEventDispatchThread()) {
logger.log(Level.WARNING, null, new RuntimeException(accessor.getMessage("TCPConnector.getConnection.isEventDispatchThread")));
}
startCacheMonitor();
SocketConnection conn = pool.getConnection(maxConnections);
//System.err.println("DEBUG: TCPConnector("+connectAs+"-"+getConnectorID()+"): getConnection("+maxConnections+"): conn.identityHashCode="+System.identityHashCode(conn));
return conn;
}
@Override
public String getProtocol() {
return PROTOCOL;
}
Socket getSocket() throws InterruptedIOException, IOException {
if(Thread.interrupted()) throw new InterruptedIOException();
Socket socket=new Socket();
socket.setKeepAlive(true);
socket.setSoLinger(true, AOPool.DEFAULT_SOCKET_SO_LINGER);
//socket.setTcpNoDelay(true);
if(local_ip!=null) socket.bind(new InetSocketAddress(local_ip, 0));
socket.connect(new InetSocketAddress(hostname, port), AOPool.DEFAULT_CONNECT_TIMEOUT);
return socket;
}
public static synchronized TCPConnector getTCPConnector(
String hostname,
String local_ip,
int port,
String connectAs,
String authenticateAs,
String password,
String daemonServer,
int poolSize,
long maxConnectionAge,
Logger logger
) throws IOException {
if(connectAs==null) throw new NullPointerException("connectAs is null");
if(authenticateAs==null) throw new NullPointerException("authenticateAs is null");
if(password==null) throw new NullPointerException("password is null");
int size=connectors.size();
for(int c=0;c© 2015 - 2024 Weber Informatics LLC | Privacy Policy