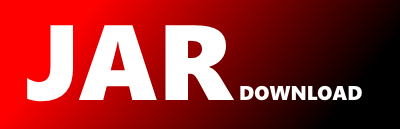
com.aoindustries.aoserv.client.VirtualDisk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2008, 2009, 2014, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.io.CompressedDataInputStream;
import com.aoindustries.io.CompressedDataOutputStream;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Calendar;
/**
* A VirtualDisk
is a block device for a VirtualServer
.
*
* @author AO Industries, Inc.
*/
final public class VirtualDisk extends CachedObjectIntegerKey {
static final int COLUMN_PKEY = 0;
static final int COLUMN_VIRTUAL_SERVER = 1;
static final int COLUMN_DEVICE = 2;
static final String COLUMN_VIRTUAL_SERVER_name = "virtual_server";
static final String COLUMN_DEVICE_name = "device";
private int virtualServer;
private String device;
private int minimumDiskSpeed;
private int minimumDiskSpeedTarget;
private int extents;
private short weight;
private short weightTarget;
private int verifyDayOfWeek;
private int verifyHourOfDay;
@Override
Object getColumnImpl(int i) {
switch(i) {
case COLUMN_PKEY: return pkey;
case COLUMN_VIRTUAL_SERVER : return virtualServer;
case COLUMN_DEVICE : return device;
case 3 : return minimumDiskSpeed==-1 ? null : minimumDiskSpeed;
case 4 : return minimumDiskSpeedTarget==-1 ? null : minimumDiskSpeedTarget;
case 5 : return extents;
case 6 : return weight;
case 7 : return weightTarget;
case 8 : return verifyDayOfWeek;
case 9 : return verifyHourOfDay;
default: throw new IllegalArgumentException("Invalid index: "+i);
}
}
public VirtualServer getVirtualServer() throws SQLException, IOException {
VirtualServer vs=table.connector.getVirtualServers().get(virtualServer);
if(vs==null) throw new SQLException("Unable to find VirtualServer: "+virtualServer);
return vs;
}
/**
* Gets the per-VirtualServer unique device (without the /dev/ prefix), such
* as xvda
or xvdb
.
*/
public String getDevice() {
return device;
}
/**
* Gets the minimum disk speed or -1
if doesn't matter.
*/
public int getMinimumDiskSpeed() {
return minimumDiskSpeed;
}
/**
* Gets the minimum disk speed target or -1
if doesn't matter.
*/
public int getMinimumDiskSpeedTarget() {
return minimumDiskSpeedTarget;
}
/**
* Gets the total extents required by this device.
*/
public int getExtents() {
return extents;
}
/**
* Gets the disk weight.
*/
public short getWeight() {
return weight;
}
/**
* Gets the disk weight target.
*/
public short getWeightTarget() {
return weightTarget;
}
/**
* Gets the day of the week verification will begin
* interpreted by the virtual server's time zone setting
* and Calendar
.
*
* @see Calendar
*/
public int getVerifyDayOfWeek() {
return verifyDayOfWeek;
}
/**
* Gets the hour of day verification will begin
* interpreted by the virtual server's time zone setting
* and Calendar
.
*
* @see Calendar
*/
public int getVerifyHourOfDay() {
return verifyHourOfDay;
}
@Override
public SchemaTable.TableID getTableID() {
return SchemaTable.TableID.VIRTUAL_DISKS;
}
@Override
public void init(ResultSet result) throws SQLException {
int pos = 1;
pkey = result.getInt(pos++);
virtualServer = result.getInt(pos++);
device = result.getString(pos++);
minimumDiskSpeed = result.getInt(pos++);
if(result.wasNull()) minimumDiskSpeed = -1;
minimumDiskSpeedTarget = result.getInt(pos++);
if(result.wasNull()) minimumDiskSpeedTarget = -1;
extents = result.getInt(pos++);
weight = result.getShort(pos++);
weightTarget = result.getShort(pos++);
verifyDayOfWeek = result.getInt(pos++);
verifyHourOfDay = result.getInt(pos++);
}
@Override
public void read(CompressedDataInputStream in) throws IOException {
pkey = in.readCompressedInt();
virtualServer = in.readCompressedInt();
device = in.readUTF().intern();
minimumDiskSpeed = in.readCompressedInt();
minimumDiskSpeedTarget = in.readCompressedInt();
extents = in.readCompressedInt();
weight = in.readShort();
weightTarget = in.readShort();
verifyDayOfWeek = in.readCompressedInt();
verifyHourOfDay = in.readCompressedInt();
}
@Override
String toStringImpl() throws SQLException, IOException {
return getVirtualServer().toStringImpl()+":/dev/"+device;
}
@Override
public void write(CompressedDataOutputStream out, AOServProtocol.Version version) throws IOException {
out.writeCompressedInt(pkey);
out.writeCompressedInt(virtualServer);
out.writeUTF(device);
if(version.compareTo(AOServProtocol.Version.VERSION_1_41)<=0) out.writeNullUTF(null); // primaryMinimumRaidType
if(version.compareTo(AOServProtocol.Version.VERSION_1_40)<=0) out.writeNullUTF(null); // secondaryMinimumRaidType
if(version.compareTo(AOServProtocol.Version.VERSION_1_41)<=0) out.writeNullUTF(null); // primaryMinimumDiskType
if(version.compareTo(AOServProtocol.Version.VERSION_1_40)<=0) out.writeNullUTF(null); // secondaryMinimumDiskType
out.writeCompressedInt(minimumDiskSpeed);
if(version.compareTo(AOServProtocol.Version.VERSION_1_43)>=0) out.writeCompressedInt(minimumDiskSpeedTarget);
if(version.compareTo(AOServProtocol.Version.VERSION_1_40)<=0) out.writeCompressedInt(minimumDiskSpeed);
out.writeCompressedInt(extents);
out.writeShort(weight);
if(version.compareTo(AOServProtocol.Version.VERSION_1_43)>=0) out.writeShort(weightTarget);
if(version.compareTo(AOServProtocol.Version.VERSION_1_40)<=0) out.writeShort(weight);
if(version.compareTo(AOServProtocol.Version.VERSION_1_42)<=0) {
out.writeBoolean(false); // primaryPhysicalVolumesLocked
out.writeBoolean(false); // secondaryPhysicalVolumesLocked
}
if(version.compareTo(AOServProtocol.Version.VERSION_1_72)>=0) {
out.writeCompressedInt(verifyDayOfWeek);
out.writeCompressedInt(verifyHourOfDay);
}
}
/**
* Begins a verification of the redundancy of the virtual disk.
*
* User must have control_virtual_server permissions on this server.
*
* @see BusinessServer#canControlVirtualServer()
*
* @return The time the verification began, which may be in the past if a verification was already in progress
*/
public long verify() throws SQLException, IOException {
return table.connector.requestLongQuery(true, AOServProtocol.CommandID.VERIFY_VIRTUAL_DISK, this.pkey);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy