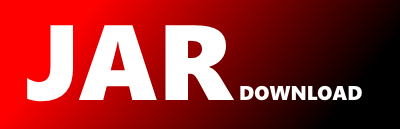
com.aoindustries.aoserv.client.email.Domain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ Platform.
* Copyright (C) 2000-2013, 2016, 2017, 2018, 2019, 2020 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client.email;
import com.aoindustries.aoserv.client.CachedObjectIntegerKey;
import com.aoindustries.aoserv.client.CannotRemoveReason;
import com.aoindustries.aoserv.client.Removable;
import com.aoindustries.aoserv.client.account.Account;
import com.aoindustries.aoserv.client.billing.Package;
import com.aoindustries.aoserv.client.dns.Record;
import com.aoindustries.aoserv.client.dns.RecordType;
import com.aoindustries.aoserv.client.linux.GroupServer;
import com.aoindustries.aoserv.client.linux.Server;
import com.aoindustries.aoserv.client.linux.UserServer;
import com.aoindustries.aoserv.client.schema.AoservProtocol;
import com.aoindustries.aoserv.client.schema.Table;
import com.aoindustries.io.stream.StreamableInput;
import com.aoindustries.io.stream.StreamableOutput;
import com.aoindustries.net.DomainName;
import com.aoindustries.validation.ValidationException;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* A EmailDomain
is one hostname/domain of email
* addresses hosted on a Server
. Multiple, unique
* email addresses may be hosted within the EmailDomain
.
* In order for mail to be routed to the Server
, a
* DNSRecord
entry of type MX
must
* point to the Server
.
*
* @see Address
* @see Record
* @see RecordType#MX
* @see Server
*
* @author AO Industries, Inc.
*/
public final class Domain extends CachedObjectIntegerKey implements Removable {
static final int
COLUMN_PKEY=0,
COLUMN_AO_SERVER=2,
COLUMN_PACKAGE=3
;
static final String COLUMN_AO_SERVER_name = "ao_server";
static final String COLUMN_DOMAIN_name = "domain";
private DomainName domain;
int ao_server;
Account.Name packageName;
public int addEmailAddress(String address) throws SQLException, IOException {
return table.getConnector().getEmail().getAddress().addEmailAddress(address, this);
}
public void addMajordomoServer(
UserServer linuxServerAccount,
GroupServer linuxServerGroup,
MajordomoVersion majordomoVersion
) throws IOException, SQLException {
table.getConnector().getEmail().getMajordomoServer().addMajordomoServer(
this,
linuxServerAccount,
linuxServerGroup,
majordomoVersion
);
}
@Override
protected Object getColumnImpl(int i) {
switch(i) {
case COLUMN_PKEY: return pkey;
case 1: return domain;
case COLUMN_AO_SERVER: return ao_server;
case COLUMN_PACKAGE: return packageName;
default: throw new IllegalArgumentException("Invalid index: " + i);
}
}
public DomainName getDomain() {
return domain;
}
public Address getEmailAddress(String address) throws IOException, SQLException {
return table.getConnector().getEmail().getAddress().getEmailAddress(address, this);
}
public List getEmailAddresses() throws IOException, SQLException {
return table.getConnector().getEmail().getAddress().getEmailAddresses(this);
}
public MajordomoServer getMajordomoServer() throws IOException, SQLException {
return table.getConnector().getEmail().getMajordomoServer().get(pkey);
}
public Package getPackage() throws SQLException, IOException {
Package packageObject = table.getConnector().getBilling().getPackage().get(packageName);
if (packageObject == null) throw new SQLException("Unable to find Package: " + packageName);
return packageObject;
}
public Server getLinuxServer() throws SQLException, IOException {
Server ao=table.getConnector().getLinux().getServer().get(ao_server);
if(ao==null) throw new SQLException("Unable to find linux.Server: "+ao_server);
return ao;
}
@Override
public Table.TableID getTableID() {
return Table.TableID.EMAIL_DOMAINS;
}
@Override
public void init(ResultSet result) throws SQLException {
try {
pkey=result.getInt(1);
domain=DomainName.valueOf(result.getString(2));
ao_server=result.getInt(3);
packageName = Account.Name.valueOf(result.getString(4));
} catch(ValidationException e) {
throw new SQLException(e);
}
}
@Override
public void read(StreamableInput in, AoservProtocol.Version protocolVersion) throws IOException {
try {
pkey = in.readCompressedInt();
domain = DomainName.valueOf(in.readUTF());
ao_server = in.readCompressedInt();
packageName = Account.Name.valueOf(in.readUTF()).intern();
} catch(ValidationException e) {
throw new IOException(e);
}
}
@Override
public List> getCannotRemoveReasons() throws SQLException, IOException {
List> reasons=new ArrayList<>();
MajordomoServer ms=getMajordomoServer();
if(ms!=null) {
Domain ed=ms.getDomain();
reasons.add(new CannotRemoveReason<>("Used by Majordomo server "+ed.getDomain()+" on "+ed.getLinuxServer().getHostname(), ms));
}
for(Address ea : getEmailAddresses()) reasons.addAll(ea.getCannotRemoveReasons());
return reasons;
}
@Override
public void remove() throws IOException, SQLException {
table.getConnector().requestUpdateIL(
true,
AoservProtocol.CommandID.REMOVE,
Table.TableID.EMAIL_DOMAINS,
pkey
);
}
@Override
public void write(StreamableOutput out, AoservProtocol.Version protocolVersion) throws IOException {
out.writeCompressedInt(pkey);
out.writeUTF(domain.toString());
out.writeCompressedInt(ao_server);
out.writeUTF(packageName.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy