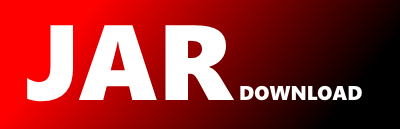
com.aoindustries.aoserv.client.email.ListTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ Platform.
* Copyright (C) 2001-2013, 2016, 2017, 2018, 2019 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client.email;
import com.aoindustries.aoserv.client.AOServConnector;
import com.aoindustries.aoserv.client.CachedTableIntegerKey;
import com.aoindustries.aoserv.client.account.Account;
import com.aoindustries.aoserv.client.aosh.AOSH;
import com.aoindustries.aoserv.client.aosh.Command;
import com.aoindustries.aoserv.client.billing.Package;
import com.aoindustries.aoserv.client.linux.GroupServer;
import com.aoindustries.aoserv.client.linux.PosixPath;
import com.aoindustries.aoserv.client.linux.Server;
import com.aoindustries.aoserv.client.linux.UserServer;
import com.aoindustries.aoserv.client.schema.AoservProtocol;
import com.aoindustries.aoserv.client.schema.Table;
import com.aoindustries.io.TerminalWriter;
import java.io.IOException;
import java.io.Reader;
import java.sql.SQLException;
import java.util.ArrayList;
/**
* @see List
*
* @author AO Industries, Inc.
*/
final public class ListTable extends CachedTableIntegerKey {
ListTable(AOServConnector connector) {
super(connector, List.class);
}
private static final OrderBy[] defaultOrderBy = {
new OrderBy(List.COLUMN_PATH_name, ASCENDING),
new OrderBy(List.COLUMN_LINUX_SERVER_ACCOUNT_name+'.'+UserServer.COLUMN_AO_SERVER_name+'.'+Server.COLUMN_HOSTNAME_name, ASCENDING)
};
@Override
protected OrderBy[] getDefaultOrderBy() {
return defaultOrderBy;
}
public int addEmailList(
PosixPath path,
UserServer lsa,
GroupServer lsg
) throws IllegalArgumentException, IOException, SQLException {
Server lsaAO = lsa.getServer();
Server lsgAO = lsg.getServer();
if(!lsaAO.equals(lsgAO)) throw new IllegalArgumentException("Mismatched servers: " + lsaAO + " and " + lsgAO);
if(
!List.isValidRegularPath(
path,
lsaAO.getHost().getOperatingSystemVersion_id()
)
) throw new IllegalArgumentException("Invalid list path: " + path);
return connector.requestIntQueryIL(
true,
AoservProtocol.CommandID.ADD,
Table.TableID.EMAIL_LISTS,
path,
lsa.getPkey(),
lsg.getPkey()
);
}
@Override
public List get(int pkey) throws IOException, SQLException {
return getUniqueRow(List.COLUMN_PKEY, pkey);
}
public java.util.List getEmailLists(Account business) throws IOException, SQLException {
Account.Name accounting=business.getName();
java.util.List cached = getRows();
int len = cached.size();
java.util.List matches=new ArrayList<>(len);
for (int c = 0; c < len; c++) {
List list = cached.get(c);
if (
list
.getLinuxServerGroup()
.getLinuxGroup()
.getPackage()
.getAccount_name()
.equals(accounting)
) matches.add(list);
}
return matches;
}
public java.util.List getEmailLists(Package pack) throws IOException, SQLException {
Account.Name packName=pack.getName();
java.util.List cached=getRows();
int size=cached.size();
java.util.List matches=new ArrayList<>(size);
for(int c=0;c getEmailLists(UserServer lsa) throws IOException, SQLException {
return getIndexedRows(List.COLUMN_LINUX_SERVER_ACCOUNT, lsa.getPkey());
}
public List getEmailList(Server ao, PosixPath path) throws IOException, SQLException {
int aoPKey = ao.getServer_pkey();
java.util.List cached=getRows();
int size=cached.size();
for(int c=0;c
© 2015 - 2025 Weber Informatics LLC | Privacy Policy