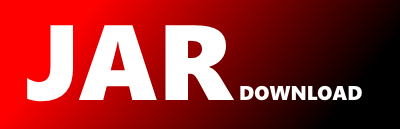
com.aoindustries.aoserv.client.linux.GroupUserTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ Platform.
* Copyright (C) 2001-2012, 2016, 2017, 2018, 2019, 2020 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client.linux;
import com.aoindustries.aoserv.client.AOServConnector;
import com.aoindustries.aoserv.client.CachedTableIntegerKey;
import com.aoindustries.aoserv.client.aosh.AOSH;
import com.aoindustries.aoserv.client.aosh.Command;
import com.aoindustries.aoserv.client.schema.AoservProtocol;
import com.aoindustries.aoserv.client.schema.Table;
import com.aoindustries.collections.AoCollections;
import com.aoindustries.io.TerminalWriter;
import com.aoindustries.lang.NullArgumentException;
import com.aoindustries.util.Tuple2;
import java.io.IOException;
import java.io.Reader;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @see GroupUser
*
* @author AO Industries, Inc.
*/
final public class GroupUserTable extends CachedTableIntegerKey {
private boolean hashBuilt = false;
private final Map,List> hash = new HashMap<>();
/**
* The group name of the primary group is hashed on first use for fast
* lookups.
*/
private boolean primaryHashBuilt = false;
private final Map primaryHash = new HashMap<>();
GroupUserTable(AOServConnector connector) {
super(connector, GroupUser.class);
}
private static final OrderBy[] defaultOrderBy = {
new OrderBy(GroupUser.COLUMN_GROUP_name, ASCENDING),
new OrderBy(GroupUser.COLUMN_USER_name, ASCENDING)
};
@Override
protected OrderBy[] getDefaultOrderBy() {
return defaultOrderBy;
}
int addLinuxGroupAccount(
Group groupObject,
User userObject
) throws IOException, SQLException {
int pkey=connector.requestIntQueryIL(
true,
AoservProtocol.CommandID.ADD,
Table.TableID.LINUX_GROUP_ACCOUNTS,
groupObject.getName(),
userObject.getUsername()
);
return pkey;
}
@Override
public GroupUser get(int id) throws IOException, SQLException {
return getUniqueRow(GroupUser.COLUMN_ID, id);
}
public List getLinuxGroupAccounts(
Group.Name group,
User.Name user
) throws IOException, SQLException {
synchronized(hash) {
if(!hashBuilt) {
hash.clear();
for(GroupUser lga : getRows()) {
Tuple2 key = new Tuple2<>(lga.getGroup_name(), lga.getUser_username());
List list = hash.get(key);
if(list == null) hash.put(key, list = new ArrayList<>());
list.add(lga);
}
// Make entries unmodifiable
for(Map.Entry,List> entry : hash.entrySet()) {
entry.setValue(
AoCollections.optimalUnmodifiableList(entry.getValue())
);
}
hashBuilt = true;
}
List lgas = hash.get(new Tuple2<>(group, user));
if(lgas == null) return Collections.emptyList();
return lgas;
}
}
List getLinuxGroups(User linuxAccount) throws IOException, SQLException {
User.Name username = linuxAccount.getUsername_id();
List rows = getRows();
int len = rows.size();
List matches = new ArrayList<>(GroupUser.MAX_GROUPS);
for(int c = 0; c < len; c++) {
GroupUser lga = rows.get(c);
if(lga.getUser_username().equals(username)) {
Group lg = lga.getGroup();
// Avoid duplicates that are now possible due to operating_system_version
if(!matches.contains(lg)) matches.add(lg);
}
}
return matches;
}
Group getPrimaryGroup(User account) throws IOException, SQLException {
NullArgumentException.checkNotNull(account, "account");
synchronized(primaryHash) {
// Rebuild the hash if needed
if(!primaryHashBuilt) {
List cache = getRows();
primaryHash.clear();
int len = cache.size();
for(int c = 0; c < len; c++) {
GroupUser lga = cache.get(c);
if(lga.isPrimary()) {
User.Name username = lga.getUser_username();
Group.Name groupName = lga.getGroup_name();
Group.Name existing = primaryHash.put(username, groupName);
if(
existing != null
&& !existing.equals(groupName)
) {
throw new SQLException(
"Conflicting primary groups for "
+ username
+ ": "
+ existing
+ " and "
+ groupName
);
}
}
}
primaryHashBuilt = true;
}
Group.Name groupName = primaryHash.get(account.getUsername_id());
if(groupName == null) return null;
// May be filtered
return connector.getLinux().getGroup().get(groupName);
}
}
@Override
public Table.TableID getTableID() {
return Table.TableID.LINUX_GROUP_ACCOUNTS;
}
@Override
public boolean handleCommand(String[] args, Reader in, TerminalWriter out, TerminalWriter err, boolean isInteractive) throws IllegalArgumentException, IOException, SQLException {
String command=args[0];
if(command.equalsIgnoreCase(Command.ADD_LINUX_GROUP_ACCOUNT)) {
if(AOSH.checkParamCount(Command.ADD_LINUX_GROUP_ACCOUNT, args, 2, err)) {
out.println(
connector.getSimpleAOClient().addLinuxGroupAccount(
AOSH.parseGroupName(args[1], "group"),
AOSH.parseLinuxUserName(args[2], "username")
)
);
out.flush();
}
return true;
} else if(command.equalsIgnoreCase(Command.REMOVE_LINUX_GROUP_ACCOUNT)) {
if(AOSH.checkParamCount(Command.REMOVE_LINUX_GROUP_ACCOUNT, args, 2, err)) {
connector.getSimpleAOClient().removeLinuxGroupAccount(
AOSH.parseGroupName(args[1], "group"),
AOSH.parseLinuxUserName(args[2], "username")
);
}
return true;
} else if(command.equalsIgnoreCase(Command.SET_PRIMARY_LINUX_GROUP_ACCOUNT)) {
if(AOSH.checkParamCount(Command.SET_PRIMARY_LINUX_GROUP_ACCOUNT, args, 2, err)) {
connector.getSimpleAOClient().setPrimaryLinuxGroupAccount(
AOSH.parseGroupName(args[1], "group"),
AOSH.parseLinuxUserName(args[2], "username")
);
}
return true;
}
return false;
}
@Override
public void clearCache() {
super.clearCache();
synchronized(hash) {
hashBuilt = false;
}
synchronized(primaryHash) {
primaryHashBuilt = false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy