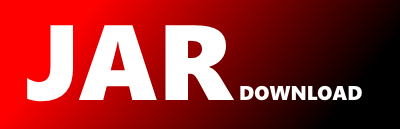
com.aoindustries.aoserv.client.web.tomcat.Site Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ Platform.
* Copyright (C) 2001-2013, 2016, 2017, 2018, 2019 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client.web.tomcat;
import com.aoindustries.aoserv.client.AOServConnector;
import com.aoindustries.aoserv.client.CachedObjectIntegerKey;
import com.aoindustries.aoserv.client.linux.PosixPath;
import com.aoindustries.aoserv.client.schema.AoservProtocol;
import com.aoindustries.aoserv.client.schema.Table;
import com.aoindustries.io.stream.StreamableInput;
import com.aoindustries.io.stream.StreamableOutput;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
/**
* An HttpdTomcatSite
indicates that an HttpdSite
* uses the Jakarta Tomcat project as its servlet engine. The servlet
* engine may be configured in several ways, only what is common to
* every type of Tomcat installation is stored in HttpdTomcatSite
.
*
* @see Site
* @see PrivateTomcatSite
*
* @author AO Industries, Inc.
*/
final public class Site extends CachedObjectIntegerKey {
static final int COLUMN_HTTPD_SITE=0;
public static final String COLUMN_HTTPD_SITE_name = "httpd_site";
private int version;
private boolean blockWebinf;
private boolean use_apache; // Only used for protocol compatibility on the server side
/**
* The minimum amount of time in milliseconds between Java VM starts.
*/
public static final int MINIMUM_START_JVM_DELAY=30000;
/**
* The minimum amount of time in milliseconds between Java VM start and stop.
*/
public static final int MINIMUM_STOP_JVM_DELAY=15000;
public int addHttpdTomcatContext(
String className,
boolean cookies,
boolean crossContext,
PosixPath docBase,
boolean override,
String path,
boolean privileged,
boolean reloadable,
boolean useNaming,
String wrapperClass,
int debug,
PosixPath workDir,
boolean serverXmlConfigured
) throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getContext().addHttpdTomcatContext(
this,
className,
cookies,
crossContext,
docBase,
override,
path,
privileged,
reloadable,
useNaming,
wrapperClass,
debug,
workDir,
serverXmlConfigured
);
}
public int addJkMount(
String path,
boolean mount
) throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getJkMount().addHttpdTomcatSiteJkMount(
this,
path,
mount
);
}
/**
* Determines if the API user is allowed to stop the Java virtual machine associated
* with this site.
*/
public boolean canStop() throws SQLException, IOException {
if(getHttpdSite().isDisabled()) return false;
SharedTomcatSite shr=getHttpdTomcatSharedSite();
if(shr!=null) return shr.canStop();
return true;
}
/**
* Determines if the API user is allowed to start the Java virtual machine associated
* with this site.
*/
public boolean canStart() throws SQLException, IOException {
if(getHttpdSite().isDisabled()) return false;
SharedTomcatSite shr=getHttpdTomcatSharedSite();
if(shr!=null) return shr.canStart();
return true;
}
@Override
protected Object getColumnImpl(int i) {
if(i == COLUMN_HTTPD_SITE) return pkey;
if(i == 1) return version;
if(i == 2) return blockWebinf;
throw new IllegalArgumentException("Invalid index: " + i);
}
public com.aoindustries.aoserv.client.web.jboss.Site getHttpdJBossSite() throws SQLException, IOException {
return table.getConnector().getWeb_jboss().getSite().get(pkey);
}
public com.aoindustries.aoserv.client.web.Site getHttpdSite() throws SQLException, IOException {
com.aoindustries.aoserv.client.web.Site obj=table.getConnector().getWeb().getSite().get(pkey);
if(obj==null) throw new SQLException("Unable to find HttpdSite: "+pkey);
return obj;
}
public Context getHttpdTomcatContext(String path) throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getContext().getHttpdTomcatContext(this, path);
}
public List getHttpdTomcatContexts() throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getContext().getHttpdTomcatContexts(this);
}
public SharedTomcatSite getHttpdTomcatSharedSite() throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getSharedTomcatSite().get(pkey);
}
public PrivateTomcatSite getHttpdTomcatStdSite() throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getPrivateTomcatSite().get(pkey);
}
public Version getHttpdTomcatVersion() throws SQLException, IOException {
Version obj=table.getConnector().getWeb_tomcat().getVersion().get(version);
if(obj==null) throw new SQLException("Unable to find HttpdTomcatVersion: "+version);
if(
obj.getTechnologyVersion(table.getConnector()).getOperatingSystemVersion(table.getConnector()).getPkey()
!= getHttpdSite().getLinuxServer().getHost().getOperatingSystemVersion_id()
) {
throw new SQLException("resource/operating system version mismatch on HttpdTomcatSite: #"+pkey);
}
// Make sure version shared JVM if is a shared site
SharedTomcatSite sharedSite = getHttpdTomcatSharedSite();
if(sharedSite!=null) {
if(
obj.getPkey()
!= sharedSite.getHttpdSharedTomcat().getHttpdTomcatVersion().getPkey()
) {
throw new SQLException("HttpdTomcatSite/HttpdSharedTomcat version mismatch on HttpdTomcatSite: #"+pkey);
}
}
return obj;
}
public List getHttpdWorkers() throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getWorker().getHttpdWorkers(this);
}
public List getJkMounts() throws IOException, SQLException {
return table.getConnector().getWeb_tomcat().getJkMount().getHttpdTomcatSiteJkMounts(this);
}
@Override
public Table.TableID getTableID() {
return Table.TableID.HTTPD_TOMCAT_SITES;
}
@Override
public void init(ResultSet result) throws SQLException {
pkey = result.getInt(1);
version = result.getInt(2);
blockWebinf = result.getBoolean(3);
use_apache = result.getBoolean(4);
}
@Override
public void read(StreamableInput in, AoservProtocol.Version protocolVersion) throws IOException {
pkey = in.readCompressedInt();
version = in.readCompressedInt();
blockWebinf = in.readBoolean();
}
public String startJVM() throws IOException, SQLException {
return table.getConnector().requestResult(
false,
AoservProtocol.CommandID.START_JVM,
new AOServConnector.ResultRequest() {
String result;
@Override
public void writeRequest(StreamableOutput out) throws IOException {
out.writeCompressedInt(pkey);
}
@Override
public void readResponse(StreamableInput in) throws IOException, SQLException {
int code=in.readByte();
if(code==AoservProtocol.DONE) {
result = in.readNullUTF();
return;
}
AoservProtocol.checkResult(code, in);
throw new IOException("Unexpected response code: "+code);
}
@Override
public String afterRelease() {
return result;
}
}
);
}
public String stopJVM() throws IOException, SQLException {
return table.getConnector().requestResult(
false,
AoservProtocol.CommandID.STOP_JVM,
new AOServConnector.ResultRequest() {
String result;
@Override
public void writeRequest(StreamableOutput out) throws IOException {
out.writeCompressedInt(pkey);
}
@Override
public void readResponse(StreamableInput in) throws IOException, SQLException {
int code=in.readByte();
if(code==AoservProtocol.DONE) {
result = in.readNullUTF();
return;
}
AoservProtocol.checkResult(code, in);
throw new IOException("Unexpected response code: "+code);
}
@Override
public String afterRelease() {
return result;
}
}
);
}
@Override
public String toStringImpl() throws SQLException, IOException {
return getHttpdSite().toStringImpl();
}
/**
* Blocks access to /META-INF
* and /WEB-INF
at the Apache level. When
* Apache serves content directly, instead of passing all
* requests to Tomcat, this helps ensure proper protection
* of these paths.
*/
public boolean getBlockWebinf() {
return blockWebinf;
}
public void setBlockWebinf(boolean blockWebinf) throws IOException, SQLException {
table.getConnector().requestUpdateIL(true, AoservProtocol.CommandID.SET_HTTPD_TOMCAT_SITE_BLOCK_WEBINF, pkey, blockWebinf);
}
@Override
public void write(StreamableOutput out, AoservProtocol.Version protocolVersion) throws IOException {
out.writeCompressedInt(pkey);
out.writeCompressedInt(version);
if(protocolVersion.compareTo(AoservProtocol.Version.VERSION_1_81_6) >= 0) {
out.writeBoolean(blockWebinf);
} else {
out.writeBoolean(use_apache);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy