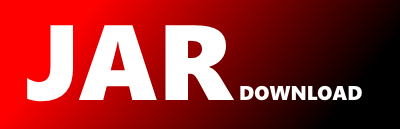
com.aoindustries.aoserv.master.ObjectFactories Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-master Show documentation
Show all versions of aoserv-master Show documentation
Master server for the AOServ Platform.
/*
* aoserv-master - Master server for the AOServ Platform.
* Copyright (C) 2013, 2015, 2017, 2018, 2019, 2020 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-master.
*
* aoserv-master is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-master is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-master. If not, see .
*/
package com.aoindustries.aoserv.master;
import com.aoindustries.aoserv.client.account.Account;
import com.aoindustries.aoserv.client.linux.Group;
import com.aoindustries.aoserv.client.linux.PosixPath;
import com.aoindustries.aoserv.client.net.FirewallZone;
import com.aoindustries.dbc.ObjectFactory;
import com.aoindustries.net.DomainName;
import com.aoindustries.net.Email;
import com.aoindustries.net.HostAddress;
import com.aoindustries.net.InetAddress;
import com.aoindustries.net.Port;
import com.aoindustries.net.Protocol;
import com.aoindustries.util.i18n.Money;
import com.aoindustries.validation.ValidationException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Currency;
import java.util.Locale;
/**
* A set of object factories for various types.
*
* @author AO Industries, Inc.
*/
final public class ObjectFactories {
/**
* Make no instances.
*/
private ObjectFactories() {
}
public static final ObjectFactory accountNameFactory = (ResultSet result) -> {
try {
return Account.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory domainNameFactory = (ResultSet result) -> {
try {
return DomainName.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory emailFactory = (ResultSet result) -> {
try {
return Email.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory groupNameFactory = (ResultSet result) -> {
try {
return Group.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory firewallZoneNameFactory = (ResultSet result) -> {
try {
return FirewallZone.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory hostAddressFactory = (ResultSet result) -> {
try {
return HostAddress.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory inetAddressFactory = (ResultSet result) -> {
try {
return InetAddress.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory linuxUserNameFactory = (ResultSet result) -> {
try {
return com.aoindustries.aoserv.client.linux.User.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory moneyFactory = (ResultSet result) -> {
try {
return new Money(Currency.getInstance(result.getString(1)), result.getBigDecimal(2));
} catch(NumberFormatException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory mysqlDatabaseNameFactory = (ResultSet result) -> {
try {
return com.aoindustries.aoserv.client.mysql.Database.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory mysqlUserNameFactory = (ResultSet result) -> {
try {
return com.aoindustries.aoserv.client.mysql.User.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory postgresqlDatabaseNameFactory = (ResultSet result) -> {
try {
return com.aoindustries.aoserv.client.postgresql.Database.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory postgresqlUserNameFactory = (ResultSet result) -> {
try {
return com.aoindustries.aoserv.client.postgresql.User.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory portFactory = (ResultSet result) -> {
try {
return Port.valueOf(
result.getInt(1),
Protocol.valueOf(result.getString(2).toUpperCase(Locale.ROOT))
);
} catch(IllegalArgumentException | ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory userNameFactory = (ResultSet result) -> {
try {
return com.aoindustries.aoserv.client.account.User.Name.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
public static final ObjectFactory posixPathFactory = (ResultSet result) -> {
try {
return PosixPath.valueOf(result.getString(1));
} catch(ValidationException e) {
throw new SQLException(e.getLocalizedMessage(), e);
}
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy