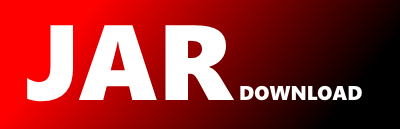
com.aoindustries.aoserv.master.SSLServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-master Show documentation
Show all versions of aoserv-master Show documentation
Master server for the AOServ Platform.
/*
* aoserv-master - Master server for the AOServ Platform.
* Copyright (C) 2000-2013, 2018, 2019, 2020 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-master.
*
* aoserv-master is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-master is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-master. If not, see .
*/
package com.aoindustries.aoserv.master;
import com.aoindustries.io.AOPool;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.net.ssl.SSLServerSocket;
import javax.net.ssl.SSLServerSocketFactory;
/**
* The AOServServer
accepts connections from an SimpleAOClient
.
* Once the connection is accepted and authenticated, the server carries out all actions requested
* by the client while providing the necessary security checks and data filters.
*
* This server is completely threaded to handle multiple, simultaneous clients.
*
* @author AO Industries, Inc.
*/
public class SSLServer extends TCPServer {
private static final Logger logger = Logger.getLogger(NetHostHandler.class.getName());
/**
* The protocol of this server.
*/
static final String PROTOCOL_SSL = "ssl";
/**
* Creates a new, running AOServServer
.
*/
SSLServer(String serverBind, int serverPort) {
super(serverBind, serverPort);
}
@Override
public String getProtocol() {
return PROTOCOL_SSL;
}
/**
* Determines if communication on this server is secure.
*/
@Override
public boolean isSecure() throws UnknownHostException {
return true;
}
@Override
public void run() {
try {
System.setProperty(
"javax.net.ssl.keyStorePassword",
MasterConfiguration.getSSLKeystorePassword()
);
System.setProperty(
"javax.net.ssl.keyStore",
MasterConfiguration.getSSLKeystorePath()
);
} catch(IOException err) {
logger.log(Level.SEVERE, null, err);
return;
}
SSLServerSocketFactory factory = (SSLServerSocketFactory)SSLServerSocketFactory.getDefault();
while (true) {
try {
InetAddress address = InetAddress.getByName(serverBind);
synchronized(System.out) {
System.out.println("Accepting SSL connections on " + address.getHostAddress() + ':' + serverPort);
}
try (SSLServerSocket SS = (SSLServerSocket)factory.createServerSocket(serverPort, 50, address)) {
while (true) {
Socket socket = SS.accept();
incConnectionCount();
try {
socket.setKeepAlive(true);
socket.setSoLinger(true, AOPool.DEFAULT_SOCKET_SO_LINGER);
//socket.setTcpNoDelay(true);
new SocketServerThread(this, socket).start();
} catch(ThreadDeath TD) {
throw TD;
} catch(Throwable T) {
logger.log(Level.SEVERE, "serverPort=" + serverPort + ", address=" + address, T);
}
}
}
} catch (ThreadDeath TD) {
throw TD;
} catch (Throwable T) {
logger.log(Level.SEVERE, null, T);
}
try {
Thread.sleep(15000);
} catch (InterruptedException err) {
logger.log(Level.WARNING, null, err);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy