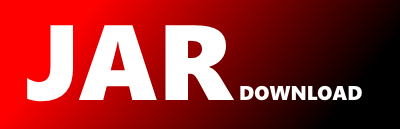
com.aoindustries.aoserv.master.billing.Package_GetTableHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-master Show documentation
Show all versions of aoserv-master Show documentation
Master server for the AOServ Platform.
/*
* aoserv-master - Master server for the AOServ Platform.
* Copyright (C) 2018, 2019 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-master.
*
* aoserv-master is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-master is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-master. If not, see .
*/
package com.aoindustries.aoserv.master.billing;
import com.aoindustries.aoserv.client.billing.Package;
import com.aoindustries.aoserv.client.master.User;
import com.aoindustries.aoserv.client.master.UserHost;
import com.aoindustries.aoserv.client.schema.Table;
import com.aoindustries.aoserv.master.CursorMode;
import com.aoindustries.aoserv.master.MasterServer;
import com.aoindustries.aoserv.master.RequestSource;
import com.aoindustries.aoserv.master.TableHandler;
import com.aoindustries.dbc.DatabaseConnection;
import com.aoindustries.io.stream.StreamableOutput;
import java.io.IOException;
import java.sql.SQLException;
import java.util.EnumSet;
import java.util.Set;
/**
* @author AO Industries, Inc.
*/
public class Package_GetTableHandler extends TableHandler.GetTableHandlerByRole {
@Override
public Set getTableIds() {
return EnumSet.of(Table.TableID.PACKAGES);
}
@Override
protected void getTableMaster(DatabaseConnection conn, RequestSource source, StreamableOutput out, boolean provideProgress, Table.TableID tableID, User masterUser) throws IOException, SQLException {
MasterServer.writeObjects(
conn,
source,
out,
provideProgress,
CursorMode.AUTO,
new Package(),
"select * from billing.\"Package\""
);
}
@Override
protected void getTableDaemon(DatabaseConnection conn, RequestSource source, StreamableOutput out, boolean provideProgress, Table.TableID tableID, User masterUser, UserHost[] masterServers) throws IOException, SQLException {
MasterServer.writeObjects(
conn,
source,
out,
provideProgress,
CursorMode.AUTO,
new Package(),
"select distinct\n"
+ " pk.*\n"
+ "from\n"
+ " master.\"UserHost\" ms,\n"
+ " account.\"AccountHost\" bs,\n"
+ " billing.\"Package\" pk\n"
+ "where\n"
+ " ms.username=?\n"
+ " and ms.server=bs.server\n"
+ " and bs.accounting=pk.accounting",
source.getCurrentAdministrator()
);
}
@Override
protected void getTableAdministrator(DatabaseConnection conn, RequestSource source, StreamableOutput out, boolean provideProgress, Table.TableID tableID) throws IOException, SQLException {
MasterServer.writeObjects(
conn,
source,
out,
provideProgress,
CursorMode.AUTO,
new Package(),
"select\n"
+ " pk2.*\n"
+ "from\n"
+ " account.\"User\" un,\n"
+ " billing.\"Package\" pk1,\n"
+ TableHandler.BU1_PARENTS_JOIN
+ " billing.\"Package\" pk2\n"
+ "where\n"
+ " un.username=?\n"
+ " and un.package=pk1.name\n"
+ " and (\n"
+ TableHandler.PK1_BU1_PARENTS_WHERE
+ " )\n"
+ " and bu1.accounting=pk2.accounting",
source.getCurrentAdministrator()
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy