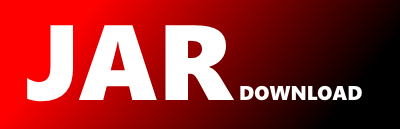
com.aoindustries.website.framework.HTMLInputStreamPage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoweb-framework Show documentation
Show all versions of aoweb-framework Show documentation
Legacy servlet-based web framework, superfast and capable but tedious to use.
/*
* aoweb-framework - Legacy servlet-based web framework, superfast and capable but tedious to use.
* Copyright (C) 2000-2013, 2015, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoweb-framework.
*
* aoweb-framework is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoweb-framework is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoweb-framework. If not, see .
*/
package com.aoindustries.website.framework;
import com.aoindustries.encoding.ChainWriter;
import com.aoindustries.io.IoUtils;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.sql.SQLException;
import javax.servlet.http.HttpServletResponse;
/**
* Pulls the content from a file with the same name and location as the .class
* and .java
but with a .html
extension. As the file is being
* sent to the client, any href='@classname'
URL is rewritten and
* maintains the current WebSiteRequest
parameters.
*
* @author AO Industries, Inc.
*/
public abstract class HTMLInputStreamPage extends InputStreamPage {
private static final long serialVersionUID = 1L;
public HTMLInputStreamPage(LoggerAccessor loggerAccessor) {
super(loggerAccessor);
}
public HTMLInputStreamPage(WebSiteRequest req) {
super(req);
}
public HTMLInputStreamPage(LoggerAccessor loggerAccessor, Object param) {
super(loggerAccessor, param);
}
@Override
public void printStream(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, InputStream in) throws IOException, SQLException {
printHTMLStream(out, req, resp, getWebPageLayout(req), in, "aoLightLink");
}
/**
* Gets the file that the text should be read from.
*/
@Override
public InputStream getInputStream() throws IOException {
return getHTMLInputStream(getClass());
}
/**
* Gets the HTML file with the same name as the provided Class.
*/
public static InputStream getHTMLInputStream(Class> clazz) throws IOException {
return HTMLInputStreamPage.class.getResourceAsStream('/'+clazz.getName().replace('.', '/')+".html");
}
/**
* Prints HTML content, parsing for special @
tags. Types of tags include:
*
* - @URL(classname) Loads a WebPage of the given class and builds a URL to it
* - @BEGIN_LIGHT_AREA Calls
layout.beginLightArea(ChainWriter)
* - @END_LIGHT_AREA Calls
layout.endLightArea(ChainWriter)
* - @END_CONTENT_LINE Calls
layout.endContentLine
* - @PRINT_CONTENT_HORIZONTAL_DIVIDER Calls
layout.printContentHorizontalDivider
* - @START_CONTENT_LINE Calls
layout.startContentLine
* - @LINK_CLASS The preferred link class for this element
*
*/
public static void printHTML(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, WebPageLayout layout, String html, String linkClass) throws IOException, SQLException {
if(req==null) out.print(html);
else {
int len=html.length();
int pos=0;
while(pos=tagPart.length()) {
if(tags[c].equalsIgnoreCase(tagPart)) {
if(c==0) layout.printContentHorizontalDivider(out, req, resp, 1, false);
else if(c==1) layout.startContentLine(out, req, resp, 1, null, null);
else if(c==2) layout.beginLightArea(req, resp, out);
else if(c==3) layout.endContentLine(out, req, resp, 1, false);
else if(c==4) layout.endLightArea(req, resp, out);
else if(c==5) out.print(linkClass==null?"aoLightLink":linkClass);
else if(c==6) {
// Read up to a ')'
while((ch=reader.read())!=-1) {
if(ch==')') {
String className=buffer.toString().substring(5, buffer.length());
out.encodeXmlAttribute(resp.encodeURL(req.getContextPath()+req.getURL(className)));
buffer.setLength(0);
break;
} else buffer.append((char)ch);
}
if(buffer.length()>0) throw new IllegalArgumentException("Unable to find closing parenthesis for @URL( substitution, buffer="+buffer.toString());
} else throw new RuntimeException("This index should not be used because it is biffer than tags.length");
buffer.setLength(0);
break Loop;
} else if(tags[c].toUpperCase().startsWith(tagPart.toUpperCase())) {
found=true;
break;
}
} else {
// Sorted with longest first, can break here
break;
}
}
if(!found) {
out.print(tagPart);
buffer.setLength(0);
break;
}
}
}
} else out.print((char)ch);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy