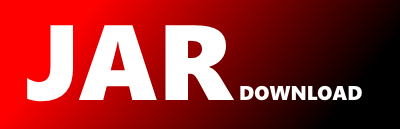
com.aoindustries.website.framework.TextOnlyLayout Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoweb-framework Show documentation
Show all versions of aoweb-framework Show documentation
Legacy servlet-based web framework, superfast and capable but tedious to use.
/*
* aoweb-framework - Legacy servlet-based web framework, superfast and capable but tedious to use.
* Copyright (C) 2003-2013, 2015, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoweb-framework.
*
* aoweb-framework is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoweb-framework is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoweb-framework. If not, see .
*/
package com.aoindustries.website.framework;
import com.aoindustries.encoding.ChainWriter;
import java.io.IOException;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletResponse;
/**
* The default text-only layout.
*
* @author AO Industries, Inc.
*/
public class TextOnlyLayout extends WebPageLayout {
public TextOnlyLayout(String[] layoutChoices) {
super(layoutChoices);
}
@Override
public void beginLightArea(WebSiteRequest req, HttpServletResponse resp, ChainWriter out, String width, boolean nowrap) {
out.print("\n"
+ " \n"
+ " ");
}
@Override
public void endLightArea(WebSiteRequest req, HttpServletResponse resp, ChainWriter out) {
out.print(" \n"
+ " \n"
+ "
\n");
}
@Override
public void beginWhiteArea(WebSiteRequest req, HttpServletResponse resp, ChainWriter out, String width, boolean nowrap) {
out.print("\n"
+ " \n"
+ " ");
}
@Override
public void endWhiteArea(WebSiteRequest req, HttpServletResponse resp, ChainWriter out) {
out.print(" \n"
+ " \n"
+ "
\n");
}
/**
* Until version 3.0 there will not be a getStatus method on the HttpServletResponse class.
* To allow code to detect the current status, anytime the status is set one should
* also set the request attribute of this name to a java.lang.Integer of the status.
*
* Matches value in com.aoindustries.website.Constants.HTTP_SERVLET_RESPONSE_STATUS for
* interoperability between the frameworks.
*/
public static final String HTTP_SERVLET_RESPONSE_STATUS = "httpServletResponseStatus";
@Override
public void startHTML(
WebPage page,
WebSiteRequest req,
HttpServletResponse resp,
ChainWriter out,
String onload
) throws IOException, SQLException {
boolean isOkResponseStatus;
{
Integer responseStatus = (Integer)req.getAttribute(HTTP_SERVLET_RESPONSE_STATUS);
isOkResponseStatus = responseStatus==null || responseStatus==HttpServletResponse.SC_OK;
}
out.print("\n"
+ "\n"
+ " \n");
// If this is not the default layout, then robots noindex
if(!isOkResponseStatus || !getName().equals(getLayoutChoices()[0])) {
out.print(" \n");
}
// Default style language
out.print("\n"
+ " ");
List parents=new ArrayList<>();
WebPage parent=page;
while(parent!=null) {
if(parent.showInLocationPath(req)) parents.add(parent);
parent=parent.getParent();
}
for(int c=(parents.size()-1);c>=0;c--) {
if(c<(parents.size()-1)) out.print(" - ");
parent=parents.get(c);
out.encodeXhtml(parent.getTitle());
}
out.print(" \n"
+ " \n"
+ " \n"
+ " \n"
+ " \n");
String copyright = page.getCopyright(req, resp, page);
if(copyright!=null && copyright.length()>0) {
out.print(" \n");
}
String author = page.getAuthor();
if(author!=null && author.length()>0) {
out.print(" \n");
}
out.print(" \n"
+ " \n");
String googleAnalyticsNewTrackingCode = getGoogleAnalyticsNewTrackingCode();
if(googleAnalyticsNewTrackingCode!=null) {
out.print(" \n");
}
printJavaScriptIncludes(req, resp, out, page);
out.print(" \n"
+ " \n"
+ " \n");
out.print(" \n"
+ " \n");
printLogo(page, out, req, resp);
boolean isLoggedIn=req.isLoggedIn();
if(isLoggedIn) {
out.print("
\n"
+ " Logout: \n");
} else {
out.print("
\n"
+ " Login: \n");
}
out.print("
\n"
+ " \n");
if(getLayoutChoices().length>=2) out.print("Layout: ");
if(printWebPageLayoutSelector(page, out, req, resp)) out.print("
\n"
+ " Search:
\n"
+ " \n"
+ "
\n"
+ " Current Location
\n"
+ " \n");
parents.clear();
parent=page;
while(parent!=null) {
if(parent.showInLocationPath(req)) parents.add(parent);
parent=parent.getParent();
}
for(int c=(parents.size()-1);c>=0;c--) {
parent=parents.get(c);
String navAlt=parent.getNavImageAlt(req);
String navSuffix=parent.getNavImageSuffix(req);
out.print(" ").print(TreePage.replaceHTML(navAlt));
if(navSuffix!=null) out.print(" (").encodeXhtml(navSuffix).print(')');
out.print("
\n");
}
out.print(" \n"
+ "
\n"
+ " Related Pages
\n"
+ " \n");
WebPage[] pages=page.getCachedPages(req);
parent=page;
if(pages.length==0) {
parent=page.getParent();
if(parent!=null) pages=parent.getCachedPages(req);
}
for(int c=-1;c").encodeXhtml(TreePage.replaceHTML(navAlt));
if(navSuffix!=null) out.print(" (").encodeXhtml(navSuffix).print(')');
out.print("
\n");
}
}
out.print(" \n"
+ "
\n");
printBelowRelatedPages(out, req);
out.print(" \n"
+ " ");
WebPage[] commonPages=getCommonPages(page, req);
if(commonPages!=null && commonPages.length>0) {
out.print(" \n");
for(int c=0;c0) out.print(" | \n");
WebPage tpage=commonPages[c];
out.print(" ").print(tpage.getNavImageAlt(req)).print(" \n");
}
out.print("
\n");
}
}
/**
* Gets the Google Analytics New Tracking Code (ga.js) or null
* if unavailable.
*/
public String getGoogleAnalyticsNewTrackingCode() {
return null;
}
@Override
public void endHTML(
WebPage page,
WebSiteRequest req,
HttpServletResponse resp,
ChainWriter out
) throws IOException, SQLException {
out.print(" \n"
+ " \n"
+ "
\n");
String googleAnalyticsNewTrackingCode = getGoogleAnalyticsNewTrackingCode();
if(googleAnalyticsNewTrackingCode!=null) {
out.print(" \n");
}
out.print(" \n"
+ "\n");
}
/**
* Starts the content area of a page.
*/
@Override
public void startContent(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, int[] contentColumnSpans, int preferredWidth) {
out.print("\n"
+ " \n");
int totalColumns=0;
for(int c=0;c0) totalColumns++;
totalColumns+=contentColumnSpans[c];
}
out.print("
\n"
+ " \n");
}
/**
* Prints a horizontal divider of the provided colspan.
*/
@Override
public void printContentHorizontalDivider(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, int[] colspansAndDirections, boolean endsInternal) {
out.print(" \n");
for(int c=0;c \n");
break;
case DOWN:
out.print(" \n");
break;
case UP_AND_DOWN:
out.print(" \n");
break;
default: throw new IllegalArgumentException("Unknown direction: "+direction);
}
}
int colspan=colspansAndDirections[c];
out.print("
\n");
}
out.print(" \n");
}
/**
* Prints the title of the page in one row in the content area.
*/
@Override
public void printContentTitle(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, String title, int contentColumns) {
startContentLine(out, req, resp, contentColumns, "center", null);
out.print("").print(title).print("
\n");
endContentLine(out, req, resp, 1, false);
}
/**
* Starts one line of content with the initial colspan set to the provided colspan.
*/
@Override
public void startContentLine(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, int colspan, String align, String width) {
out.print(" \n"
+ " 0) {
out.append(" style='width:");
out.append(width);
out.append('\'');
}
out.print(" valign='top'");
if(colspan!=1) out.print(" colspan='").print(colspan).print('\'');
if(align!=null && !align.equalsIgnoreCase("left")) out.print(" align='").print(align).print('\'');
out.print('>');
}
/**
* Starts one line of content with the initial colspan set to the provided colspan.
*/
@Override
public void printContentVerticalDivider(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, int direction, int colspan, int rowspan, String align, String width) {
out.print(" \n");
switch(direction) {
case UP_AND_DOWN:
out.print(" \n");
break;
case NONE:
break;
default: throw new IllegalArgumentException("Unknown direction: "+direction);
}
out.print(" 0) {
out.append(" style='width:");
out.append(width);
out.append('\'');
}
out.print(" valign='top'");
if(colspan!=1) out.print(" colspan='").print(colspan).print('\'');
if(rowspan!=1) out.print(" rowspan='").print(rowspan).print('\'');
if(align!=null && !align.equals("left")) out.print(" align='").print(align).print('\'');
out.print('>');
}
/**
* Ends one line of content.
*/
@Override
public void endContentLine(ChainWriter out, WebSiteRequest req, HttpServletResponse resp, int rowspan, boolean endsInternal) {
out.print(" \n"
+ " \n");
}
/**
* Ends the content area of a page.
*/
@Override
public void endContent(WebPage page, ChainWriter out, WebSiteRequest req, HttpServletResponse resp, int[] contentColumnSpans) throws IOException, SQLException {
int totalColumns=0;
for(int c=0;c0) totalColumns+=1;
totalColumns+=contentColumnSpans[c];
}
out.print("
\n");
String copyright=page.getCopyright(req, resp, page);
if(copyright!=null && copyright.length()>0) {
out.print(" ").print(copyright).print(" \n");
}
out.print("
\n");
}
@Override
public String getName() {
return "Text";
}
public WebPage[] getCommonPages(WebPage page, WebSiteRequest req) throws IOException, SQLException {
return null;
}
public void printLogo(WebPage page, ChainWriter out, WebSiteRequest req, HttpServletResponse resp) throws IOException, SQLException {
}
/**
* Prints content below the related pages area on the left.
*/
public void printBelowRelatedPages(ChainWriter out, WebSiteRequest req) throws IOException, SQLException {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy