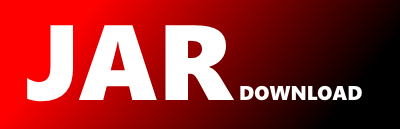
com.aoindustries.website.TextSkin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoweb-struts-core Show documentation
Show all versions of aoweb-struts-core Show documentation
Core API for legacy Struts-based site framework with AOServ Platform control panels.
/*
* aoweb-struts-core - Core API for legacy Struts-based site framework with AOServ Platform control panels.
* Copyright (C) 2007-2013, 2015, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoweb-struts-core.
*
* aoweb-struts-core is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoweb-struts-core is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoweb-struts-core. If not, see .
*/
package com.aoindustries.website;
import com.aoindustries.aoserv.client.AOServConnector;
import com.aoindustries.aoserv.client.Brand;
import com.aoindustries.encoding.ChainWriter;
import com.aoindustries.encoding.NewEncodingUtils;
import com.aoindustries.encoding.TextInJavaScriptEncoder;
import static com.aoindustries.encoding.TextInXhtmlAttributeEncoder.encodeTextInXhtmlAttribute;
import com.aoindustries.encoding.TextInXhtmlEncoder;
import static com.aoindustries.encoding.TextInXhtmlEncoder.encodeTextInXhtml;
import com.aoindustries.net.UrlUtils;
import com.aoindustries.util.i18n.EditableResourceBundle;
import com.aoindustries.website.skintags.Child;
import com.aoindustries.website.skintags.Meta;
import com.aoindustries.website.skintags.PageAttributes;
import com.aoindustries.website.skintags.Parent;
import java.io.IOException;
import java.net.URLEncoder;
import java.sql.SQLException;
import java.util.List;
import java.util.Locale;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.JspWriter;
import org.apache.struts.Globals;
import org.apache.struts.util.MessageResources;
/**
* The skin for the home page of the site.
*
* @author AO Industries, Inc.
*/
public class TextSkin extends Skin {
/**
* Reuse a single instance, not synchronized because if more than one is
* made no big deal.
*/
private static TextSkin instance;
public static TextSkin getInstance() {
if(instance==null) instance = new TextSkin();
return instance;
}
protected TextSkin() {}
@Override
public String getName() {
return "Text";
}
@Override
public String getDisplay(HttpServletRequest req) throws JspException {
HttpSession session = req.getSession();
Locale locale = (Locale)session.getAttribute(Globals.LOCALE_KEY);
MessageResources applicationResources = (MessageResources)req.getAttribute("/ApplicationResources");
if(applicationResources==null) throw new JspException("Unable to load resources: /ApplicationResources");
return applicationResources.getMessage(locale, "TextSkin.name");
}
/**
* Print the logo for the top left part of the page.
*/
public void printLogo(HttpServletRequest req, HttpServletResponse resp, JspWriter out, String urlBase) throws JspException {
// Print no logo by default
}
/**
* Prints the search form, if any exists.
*/
public void printSearch(HttpServletRequest req, HttpServletResponse resp, JspWriter out) throws JspException {
}
/**
* Prints the common pages area, which is at the top of the site.
*/
public void printCommonPages(HttpServletRequest req, HttpServletResponse resp, JspWriter out) throws JspException {
}
/**
* Prints the lines to include any CSS files.
*/
public void printCssIncludes(HttpServletResponse resp, JspWriter out, String urlBase) throws JspException {
}
/**
* Prints the lines for any JavaScript sources.
*/
public void printJavaScriptSources(HttpServletResponse resp, JspWriter out, String urlBase) throws JspException {
}
/**
* Prints the line for the favicon.
*/
public void printFavIcon(HttpServletResponse resp, JspWriter out, String urlBase) throws JspException {
}
public static MessageResources getMessageResources(HttpServletRequest req) throws JspException {
MessageResources resources = (MessageResources)req.getAttribute("/ApplicationResources");
if(resources==null) throw new JspException("Unable to load resources: /ApplicationResources");
return resources;
}
@Override
public void startSkin(HttpServletRequest req, HttpServletResponse resp, JspWriter out, PageAttributes pageAttributes) throws JspException {
try {
String layout = pageAttributes.getLayout();
if(!layout.equals(PageAttributes.LAYOUT_NORMAL)) throw new JspException("TODO: Implement layout: "+layout);
HttpSession session = req.getSession();
Locale locale = (Locale)session.getAttribute(Globals.LOCALE_KEY);
MessageResources applicationResources = getMessageResources(req);
String urlBase = getUrlBase(req);
String path = pageAttributes.getPath();
if(path.startsWith("/")) path=path.substring(1);
final String fullPath = urlBase + path;
final String encodedFullPath = resp.encodeURL(UrlUtils.encodeUrlPath(fullPath, resp.getCharacterEncoding()));
ServletContext servletContext = session.getServletContext();
SiteSettings settings = SiteSettings.getInstance(servletContext);
List skins = settings.getSkins();
boolean isOkResponseStatus;
{
Integer responseStatus = (Integer)req.getAttribute(Constants.HTTP_SERVLET_RESPONSE_STATUS);
isOkResponseStatus = responseStatus==null || responseStatus==HttpServletResponse.SC_OK;
}
out.print(" \n");
// If this is not the default skin, then robots noindex
boolean robotsMetaUsed = false;
if(!isOkResponseStatus || !getName().equals(skins.get(0).getName())) {
out.print(" \n");
robotsMetaUsed = true;
}
// Default style language
out.print(" \n"
+ " \n");
// If this is an authenticated page, redirect to session timeout after one hour
AOServConnector aoConn = AuthenticatedAction.getAoConn(req, resp);
if(isOkResponseStatus && aoConn!=null) {
out.print(" \n");
}
for(Meta meta : pageAttributes.getMetas()) {
// Skip ROBOTS if not on default skin
boolean isRobots = meta.getName().equalsIgnoreCase("ROBOTS");
if(!robotsMetaUsed || !isRobots) {
out.print(" \n");
if(isRobots) robotsMetaUsed = true;
}
}
out.print(" ");
// No more page stack, just show current page only
/*
List parents = pageAttributes.getParents();
for(Parent parent : parents) {
encodeTextInXhtml(parent.getTitle(), out);
out.print(" - ");
}
*/
encodeTextInXhtml(pageAttributes.getTitle(), out);
out.print(" \n"
+ " \n");
Brand brand = settings.getBrand();
if(isOkResponseStatus) {
String googleVerify = brand.getAowebStrutsGoogleVerifyContent();
if(googleVerify!=null) {
out.print(" \n");
}
}
String keywords = pageAttributes.getKeywords();
if(keywords!=null && keywords.length()>0) {
out.print(" \n");
}
String description = pageAttributes.getDescription();
if(description!=null && description.length()>0) {
out.print(" \n"
+ " \n");
}
String copyright = pageAttributes.getCopyright();
if(copyright!=null && copyright.length()>0) {
out.print(" \n");
}
String author = pageAttributes.getAuthor();
if(author!=null && author.length()>0) {
out.print(" \n");
}
List languages = settings.getLanguages(req);
printAlternativeLinks(resp, out, fullPath, languages);
out.print(" \n"
+ " \n");
printCssIncludes(resp, out, urlBase);
defaultPrintLinks(out, pageAttributes, resp.getCharacterEncoding());
printJavaScriptSources(resp, out, urlBase);
out.print(" \n");
String googleAnalyticsNewTrackingCode = brand.getAowebStrutsGoogleAnalyticsNewTrackingCode();
if(googleAnalyticsNewTrackingCode!=null) {
out.print(" \n");
}
printFavIcon(resp, out, urlBase);
out.print(" \n"
+ " 0) {
out.print(" onload=\""); out.print(onload); out.print('"');
}
out.print(">\n"
+ " \n"
+ " \n"
+ " \n");
printLogo(req, resp, out, urlBase);
if(aoConn!=null) {
out.print("
\n"
+ " ");
out.print(applicationResources.getMessage(locale, "TextSkin.logoutPrompt"));
out.print("\n");
} else {
out.print("
\n"
+ " ");
out.print(applicationResources.getMessage(locale, "TextSkin.loginPrompt"));
out.print("\n");
}
out.print("
\n"
+ " \n");
if(skins.size()>1) {
out.print("\n"
+ "
\n");
}
if(languages.size()>1) {
out.print(" ");
for(Language language : languages) {
String url = language.getUrl();
if(language.getCode().equalsIgnoreCase(locale.getLanguage())) {
out.print("
");
} else {
out.print("
");
ChainWriter.writeHtmlImagePreloadJavaScript(resp.encodeURL(urlBase + language.getFlagOnSrc(req, locale)), out);
}
}
out.print("
\n");
}
printSearch(req, resp, out);
out.print(" \n"
+ "
\n"
// Display the parents
+ " ");
out.print(applicationResources.getMessage(locale, "TextSkin.currentLocation"));
out.print("
\n"
+ " \n");
List parents = pageAttributes.getParents();
for(Parent parent : parents) {
String navAlt = parent.getNavImageAlt();
String parentPath = parent.getPath();
if(parentPath.startsWith("/")) parentPath=parentPath.substring(1);
out.print(" ");
encodeTextInXhtml(navAlt, out);
out.print("
\n");
}
// Always include the current page in the current location area
out.print(" ");
encodeTextInXhtml(pageAttributes.getNavImageAlt(), out);
out.print("
\n"
+ " \n"
+ "
\n"
+ " ");
out.print(applicationResources.getMessage(locale, "TextSkin.relatedPages"));
out.print("
\n"
// Display the siblings
+ " \n");
List siblings = pageAttributes.getChildren();
if(siblings.isEmpty() && !parents.isEmpty()) {
siblings = parents.get(parents.size()-1).getChildren();
}
for(Child sibling : siblings) {
String navAlt=sibling.getNavImageAlt();
String siblingPath = sibling.getPath();
if(siblingPath.startsWith("/")) siblingPath=siblingPath.substring(1);
out.print(" ");
encodeTextInXhtml(navAlt, out);
out.print("
\n");
}
out.print(" \n"
+ "
\n");
printBelowRelatedPages(req, out);
out.print(" \n"
+ " \n");
printCommonPages(req, resp, out);
} catch(IOException err) {
throw new JspException(err);
} catch(SQLException err) {
throw new JspException(err);
}
}
public static void defaultPrintLinks(JspWriter out, PageAttributes pageAttributes, String encoding) throws JspException {
try {
for(PageAttributes.Link link : pageAttributes.getLinks()) {
String conditionalCommentExpression = link.getConditionalCommentExpression();
if(conditionalCommentExpression!=null) {
out.print(" \n");
}
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void startContent(HttpServletRequest req, HttpServletResponse resp, JspWriter out, PageAttributes pageAttributes, int[] colspans, String width) throws JspException {
try {
out.print(" 0) {
out.print(" width='");
out.print(width);
out.print('\'');
}
out.print(">\n"
+ " \n");
int totalColumns=0;
for(int c=0;c0) totalColumns++;
totalColumns+=colspans[c];
}
out.print("
\n"
+ " \n");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void printContentTitle(HttpServletRequest req, HttpServletResponse resp, JspWriter out, String title, int colspan) throws JspException {
try {
startContentLine(req, resp, out, colspan, "center", null);
out.print("");
encodeTextInXhtml(title, out);
out.print("
\n");
endContentLine(req, resp, out, 1, false);
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void startContentLine(HttpServletRequest req, HttpServletResponse resp, JspWriter out, int colspan, String align, String width) throws JspException {
try {
out.print(" \n"
+ " 0) {
out.append(" style='width:");
out.append(width);
out.append('\'');
}
out.print(" valign='top'");
if(colspan!=1) {
out.print(" colspan='");
out.print(colspan);
out.print('\'');
}
if(align!=null && (align=align.trim()).length()>0) {
out.print(" align='");
out.print(align);
out.print('\'');
}
out.print('>');
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void printContentVerticalDivider(HttpServletRequest req, HttpServletResponse resp, JspWriter out, boolean visible, int colspan, int rowspan, String align, String width) throws JspException {
try {
out.print(" \n");
if(visible) out.print(" \n");
out.print(" 0) {
out.append(" style='width:");
out.append(width);
out.append('\'');
}
out.print(" valign='top'");
if(colspan!=1) {
out.print(" colspan='");
out.print(colspan);
out.print('\'');
}
if(rowspan!=1) {
out.print(" rowspan='");
out.print(rowspan);
out.print('\'');
}
if(align!=null && (align=align.trim()).length()>0) {
out.print(" align='");
out.print(align);
out.print('\'');
}
out.print('>');
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void endContentLine(HttpServletRequest req, HttpServletResponse resp, JspWriter out, int rowspan, boolean endsInternal) throws JspException {
try {
out.print(" \n"
+ " \n");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void printContentHorizontalDivider(HttpServletRequest req, HttpServletResponse resp, JspWriter out, int[] colspansAndDirections, boolean endsInternal) throws JspException {
try {
out.print(" \n");
for(int c=0;c0) {
int direction=colspansAndDirections[c-1];
switch(direction) {
case UP:
out.print(" \n");
break;
case DOWN:
out.print(" \n");
break;
case UP_AND_DOWN:
out.print(" \n");
break;
default: throw new IllegalArgumentException("Unknown direction: "+direction);
}
}
int colspan=colspansAndDirections[c];
out.print("
\n");
}
out.print(" \n");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void endContent(HttpServletRequest req, HttpServletResponse resp, JspWriter out, PageAttributes pageAttributes, int[] colspans) throws JspException {
try {
int totalColumns=0;
for(int c=0;c0) totalColumns+=1;
totalColumns+=colspans[c];
}
out.print("
\n");
String copyright = pageAttributes.getCopyright();
if(copyright!=null && copyright.length()>0) {
out.print(" ");
out.print(copyright);
out.print(" \n");
}
out.print("
\n");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void endSkin(HttpServletRequest req, HttpServletResponse resp, JspWriter out, PageAttributes pageAttributes) throws JspException {
try {
out.print(" \n"
+ " \n"
+ "
\n");
EditableResourceBundle.printEditableResourceBundleLookups(
TextInJavaScriptEncoder.textInJavaScriptEncoder,
TextInXhtmlEncoder.textInXhtmlEncoder,
out,
4,
true
);
printGoogleAnalyticsTrackPageViewScript(req, out, SiteSettings.getInstance(req.getSession().getServletContext()).getBrand().getAowebStrutsGoogleAnalyticsNewTrackingCode());
out.print(" \n");
} catch(IOException err) {
throw new JspException(err);
} catch(SQLException err) {
throw new JspException(err);
}
}
/**
* Reusable implemention of Google analytics pageview tracking script.
*
* @param googleAnalyticsNewTrackingCode if null
will not print anything
*/
public static void printGoogleAnalyticsTrackPageViewScript(HttpServletRequest req, Appendable out, String googleAnalyticsNewTrackingCode) throws IOException {
if(googleAnalyticsNewTrackingCode!=null) {
Integer responseStatus = (Integer)req.getAttribute(Constants.HTTP_SERVLET_RESPONSE_STATUS);
boolean isOk = responseStatus==null || responseStatus==HttpServletResponse.SC_OK;
out.append(" \n");
}
}
@Override
public void beginLightArea(HttpServletRequest req, HttpServletResponse resp, JspWriter out, String width, boolean nowrap) throws JspException {
try {
out.print("\n"
+ " \n"
+ " ");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void endLightArea(HttpServletRequest req, HttpServletResponse resp, JspWriter out) throws JspException {
try {
out.print(" \n"
+ " \n"
+ "
\n");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void beginWhiteArea(HttpServletRequest req, HttpServletResponse resp, JspWriter out, String width, boolean nowrap) throws JspException {
try {
out.print("\n"
+ " \n"
+ " ");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void endWhiteArea(HttpServletRequest req, HttpServletResponse resp, JspWriter out) throws JspException {
try {
out.print(" \n"
+ " \n"
+ "
\n");
} catch(IOException err) {
throw new JspException(err);
}
}
@Override
public void printAutoIndex(HttpServletRequest req, HttpServletResponse resp, JspWriter out, PageAttributes pageAttributes) throws JspException {
try {
String urlBase = getUrlBase(req);
//Locale locale = resp.getLocale();
out.print("\n");
List siblings = pageAttributes.getChildren();
if(siblings.isEmpty()) {
List parents = pageAttributes.getParents();
if(!parents.isEmpty()) siblings = parents.get(parents.size()-1).getChildren();
}
for(Child sibling : siblings) {
String navAlt=sibling.getNavImageAlt();
String siblingPath = sibling.getPath();
if(siblingPath.startsWith("/")) siblingPath=siblingPath.substring(1);
out.print(" \n"
+ " ");
encodeTextInXhtml(navAlt, out);
out.print(" \n"
+ " \n"
+ " ");
String description = sibling.getDescription();
if(description!=null && (description=description.trim()).length()>0) {
encodeTextInXhtml(description, out);
} else {
String title = sibling.getTitle();
if(title!=null && (title=title.trim()).length()>0) {
encodeTextInXhtml(title, out);
} else {
out.print(" ");
}
}
out.print(" \n"
+ " \n")
;
}
out.print("
\n");
} catch(IOException err) {
throw new JspException(err);
}
}
/**
* Prints content below the related pages area on the left.
*/
public void printBelowRelatedPages(HttpServletRequest req, JspWriter out) throws JspException {
}
/**
* Begins a popup group.
*
* @see #defaultBeginPopupGroup(javax.servlet.http.HttpServletRequest, javax.servlet.jsp.JspWriter, long)
*/
@Override
public void beginPopupGroup(HttpServletRequest req, JspWriter out, long groupId) throws JspException {
defaultBeginPopupGroup(req, out, groupId);
}
/**
* Default implementation of beginPopupGroup.
*/
public static void defaultBeginPopupGroup(HttpServletRequest req, JspWriter out, long groupId) throws JspException {
try {
out.print("\n");
} catch(IOException err) {
throw new JspException(err);
}
}
/**
* Ends a popup group.
*
* @see #defaultEndPopupGroup(javax.servlet.http.HttpServletRequest, javax.servlet.jsp.JspWriter, long)
*/
@Override
public void endPopupGroup(HttpServletRequest req, JspWriter out, long groupId) throws JspException {
defaultEndPopupGroup(req, out, groupId);
}
/**
* Default implementation of endPopupGroup.
*/
public static void defaultEndPopupGroup(HttpServletRequest req, JspWriter out, long groupId) throws JspException {
// Nothing at the popup group end
}
/**
* Begins a popup that is in a popup group.
*
* @see #defaultBeginPopup(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, javax.servlet.jsp.JspWriter, long, long, java.lang.String, java.lang.String)
*/
@Override
public void beginPopup(HttpServletRequest req, HttpServletResponse resp, JspWriter out, long groupId, long popupId, String width) throws JspException {
defaultBeginPopup(req, resp, out, groupId, popupId, width, getUrlBase(req));
}
/**
* Default implementation of beginPopup.
*/
public static void defaultBeginPopup(HttpServletRequest req, HttpServletResponse resp, JspWriter out, long groupId, long popupId, String width, String urlBase) throws JspException {
try {
HttpSession session = req.getSession();
Locale locale = (Locale)session.getAttribute(Globals.LOCALE_KEY);
MessageResources applicationResources = getMessageResources(req);
out.print("
\n"
+ " 0) {
out.print("width:");
try {
int widthInt = Integer.parseInt(width);
out.print(widthInt);
out.print("px");
} catch(NumberFormatException err) {
out.print(width);
}
out.print(';');
}
out.print("\">\n"
+ " \n"
+ " \n"
+ " ;)
\n"
+ " \n"
+ " ;)
\n"
+ " \n"
+ " \n"
+ " \n"
+ " ");
} catch(IOException err) {
throw new JspException(err);
}
}
/**
* Prints a popup close link/image/button for a popup that is part of a popup group.
*
* @see #defaultPrintPopupClose(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, javax.servlet.jsp.JspWriter, long, long, java.lang.String)
*/
@Override
public void printPopupClose(HttpServletRequest req, HttpServletResponse resp, JspWriter out, long groupId, long popupId) throws JspException {
defaultPrintPopupClose(req, resp, out, groupId, popupId, getUrlBase(req));
}
/**
* Default implementation of printPopupClose.
*/
public static void defaultPrintPopupClose(HttpServletRequest req, HttpServletResponse resp, JspWriter out, long groupId, long popupId, String urlBase) throws JspException {
try {
HttpSession session = req.getSession();
Locale locale = (Locale)session.getAttribute(Globals.LOCALE_KEY);
MessageResources applicationResources = getMessageResources(req);
out.print("
");
} catch(IOException err) {
throw new JspException(err);
}
}
/**
* Ends a popup that is in a popup group.
*
* @see #defaultEndPopup(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, javax.servlet.jsp.JspWriter, long, long, java.lang.String, java.lang.String)
*/
@Override
public void endPopup(HttpServletRequest req, HttpServletResponse resp, JspWriter out, long groupId, long popupId, String width) throws JspException {
TextSkin.defaultEndPopup(req, resp, out, groupId, popupId, width, getUrlBase(req));
}
/**
* Default implementation of endPopup.
*/
public static void defaultEndPopup(HttpServletRequest req, HttpServletResponse resp, JspWriter out, long groupId, long popupId, String width, String urlBase) throws JspException {
try {
out.print(" \n"
+ " \n"
+ " \n"
+ " \n"
+ " ;)
\n"
+ " \n"
+ " ;)
\n"
+ " \n"
+ "
\n"
+ " \n"
+ "\n"
+ "");
} catch(IOException err) {
throw new JspException(err);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy