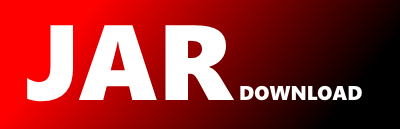
com.aol.cyclops.functionaljava.FJ Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-functionaljava Show documentation
Show all versions of cyclops-functionaljava Show documentation
Converters and Comprehenders for FunctionalJava
package com.aol.cyclops.functionaljava;
import java.util.Optional;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
import com.aol.cyclops.control.AnyM;
import com.aol.cyclops.control.For;
import com.aol.cyclops.control.Maybe;
import com.aol.cyclops.internal.monads.ComprehenderSelector;
import com.aol.cyclops.types.anyM.AnyMSeq;
import com.aol.cyclops.types.anyM.AnyMValue;
import com.aol.cyclops.util.function.QuadFunction;
import com.aol.cyclops.util.function.TriFunction;
import fj.F0;
import fj.P1;
import fj.control.Trampoline;
import fj.data.Either;
import fj.data.IO;
import fj.data.IOFunctions;
import fj.data.IterableW;
import fj.data.List;
import fj.data.Option;
import fj.data.Reader;
import fj.data.State;
import fj.data.Stream;
import fj.data.Validation;
import fj.data.Writer;
/**
* FunctionalJava Cyclops integration point
*
* @author johnmcclean
*
*/
public interface FJ {
public static Maybe maybe(Option opt){
return opt.isNone() ? Maybe.none() : Maybe.just(opt.some());
}
/**
* Methods for making working with FJ's Trampoline a little more Java8 friendly
*
*/
public static class Trampoline8 {
/**
*
*
* {@code
* List list = FJ.anyM(FJ.Trampoline.suspend(() -> Trampoline.pure("hello world")))
.map(String::toUpperCase)
.asSequence()
.toList();
// ["HELLO WORLD"]
* }
*
*
* @param s Suspend using a Supplier
*
* @return Next Trampoline stage
*/
public static fj.control.Trampoline suspend(Supplier> s) {
return fj.control.Trampoline.suspend(new P1>() {
@Override
public fj.control.Trampoline _1() {
return s.get();
}
});
}
}
/**
* Unwrap an AnyM to a Reader
*
*
* {@code
* FJ.unwrapReader(FJ.anyM(Reader.unit( (Integer a) -> "hello "+a ))
.map(String::toUpperCase))
.f(10)
*
* }
*
*
* @param anyM Monad to unwrap
* @return unwrapped reader
*/
public static Reader unwrapReader(AnyM anyM) {
Reader unwrapper = Reader.unit(a -> 1);
return (Reader) new ComprehenderSelector().selectComprehender(unwrapper)
.executeflatMap(unwrapper, i -> anyM.unwrap());
}
/**
*
* {@code
* FJ.unwrapWriter(FJ.anyM(writer)
.map(String::toUpperCase),writer)
.value()
* }
*
*
* @param anyM AnyM to unwrap to Writer
* @param unwrapper Writer of same type to do unwrapping
* @return Unwrapped writer
*/
public static Writer unwrapWriter(AnyM anyM, Writer unwrapper) {
return (Writer) new ComprehenderSelector().selectComprehender(unwrapper)
.executeflatMap(unwrapper, i -> anyM.unwrap());
}
/**
*
* {@code
* FJ.unwrapState(FJ.anyM(State.constant("hello"))
.map(String::toUpperCase))
.run("")
._2()
*
* }
*
* @param anyM AnyM to unwrap to State monad
* @return State monad
*/
public static State unwrapState(AnyM anyM) {
State unwrapper = State.constant(1);
return (State) new ComprehenderSelector().selectComprehender(unwrapper)
.executeflatMap(unwrapper, i -> anyM.unwrap());
}
/**
*
* {@code
* FJ.unwrapIO(
FJ.anyM(IOFunctions.lazy(a->{ System.out.println("hello world"); return a;}))
.map(a-> {System.out.println("hello world2"); return a;}) )
.run();
*
* }
*
* @param anyM to unwrap to IO Monad
* @return IO Monad
*/
public static IO unwrapIO(AnyMValue anyM) {
IO unwrapper = IOFunctions.unit(1);
return (IO) new ComprehenderSelector().selectComprehender(unwrapper)
.executeflatMap(unwrapper, i -> anyM.unwrap());
}
/**
*
*
* {@code
* FJ.anyM(IOFunctions.lazy(a->{ System.out.println("hello world"); return a;}))
* }
*
*
* @param ioM Construct an AnyM from the supplied IO Monad
* @return AnyM
*/
public static AnyMValue io(IO ioM) {
return AnyM.ofValue(ioM);
}
/**
*
* {@code
* AnyM anyM = FJ.anyM(State.constant("hello"))
.map(String::toUpperCase)
}
* @param stateM Construct an AnyM from the supplied State Monad
* @return AnyM
*/
public static AnyMValue state(State, T> stateM) {
return AnyM.ofValue(stateM);
}
/**
*
* {@code
* FJ.anyM(Validation.success(success()))
.map(String::toUpperCase)
.toSequence()
.toList()
* }
*
* @param validationM to construct an AnyM from
* @return AnyM
*/
public static AnyMValue validation(Validation, T> validationM) {
return AnyM.ofValue(validationM);
}
/**
*
* {@code
* FJ.anyMValue(Writer.unit("hello",Monoid.stringMonoid))
* }
*
*
* @param writerM to construct an AnyM from
* @return AnyM
*/
public static AnyMValue writer(Writer writerM) {
return AnyM.ofValue(writerM);
}
/**
*
* {@code
* FJ.reader(Reader.unit( (Integer a) -> "hello "+a )
* }
*
*
* Create an AnyM, input type will be ignored, while Reader is wrapped in AnyM
* Extract to access and provide input value
*
* @param readerM to create AnyM from
* @return AnyM
*/
public static AnyMValue reader(Reader, T> readerM) {
return AnyM.ofValue(readerM);
}
/**
*
* {@code
* FJ.trampoline(FJ.Trampoline.suspend(()-> Trampoline.pure(finalStage()))
* }
*
*
* @param trampolineM to create AnyM from
* @return AnyM
*/
public static AnyMValue trampoline(fj.control.Trampoline trampolineM) {
return AnyM.ofValue(trampolineM);
}
/**
*
* {@code
* FJ.iterableW(IterableW.wrap(Arrays.asList("hello world")))
.map(String::toUpperCase)
.toSequence()
.toList()
*
* }
*
* @param iterableWM to create AnyM from
* @return AnyM
*/
public static AnyMSeq iterableW(IterableW iterableWM) {
return AnyM.ofSeq(iterableWM);
}
/**
* (Right biased)
*
* {@code
* FJ.either(Either.right("hello world"))
.map(String::toUpperCase)
.flatMapOptional(Optional::of)
.toSequence()
.toList()
* }
*
*
* @param eitherM to construct AnyM from
* @return AnyM
*/
public static AnyMValue either(Either, T> eitherM) {
return AnyM.ofValue(eitherM);
}
/**
*
* {@code
* FJ.right(Either.right("hello world").right())
.map(String::toUpperCase)
.toSequence()
.toList()
//[HELLO WORLD]
* }
*
*
* @param rM Right projection to construct AnyM from
* @return AnyM
*/
public static AnyMValue right(Either, T>.RightProjection, T> rM) {
if (rM.toOption()
.isSome())
return AnyM.ofValue(Either.right(rM.value())
.right());
else
return AnyM.ofValue(Optional.empty());
}
/**
*
* {@code
* FJ.left(Either.left("hello world").left())
.map(String::toUpperCase)
.flatMapOptional(Optional::of)
.toSequence()
.toList()
* }
* //[HELLO WORLD]
*
*
* @param lM Left Projection to construct AnyM from
* @return AnyM
*/
public static AnyMValue left(Either.LeftProjection lM) {
if (lM.toOption()
.isSome()) // works in the opposite way to javaslang
return AnyM.ofValue(Either.right(lM.value())
.right());
else
return AnyM.ofValue(Optional.empty());
}
/**
*
* {@code
* FJ.anyM(Option.some("hello world"))
.map(String::toUpperCase)
.toSequence()
.toList()
* }
* //[HELLO WORLD]
*
*
* @param optionM to construct AnyM from
* @return AnyM
*/
public static AnyMValue option(Option optionM) {
return AnyM.ofValue(optionM);
}
/**
*
* {@code
* FJ.stream(Stream.stream("hello world"))
.map(String::toUpperCase)
.flatMap(i->AnyMonads.anyM(java.util.stream.Stream.of(i)))
.toSequence()
.toList()
* }
* //[HELLO WORLD]
*
*
* @param streamM to construct AnyM from
* @return AnyM
*/
public static AnyMSeq stream(Stream streamM) {
return AnyM.ofSeq(streamM);
}
/**
*
* {@code
* FJ.list(List.list("hello world"))
.map(String::toUpperCase)
.toSequence()
.toList()
* }
*
* @param listM to Construct AnyM from
* @return AnyM
*/
public static AnyMSeq list(List listM) {
return AnyM.ofSeq(listM);
}
public interface ForOption {
static Option each4(Option extends T1> value1,
Function super T1, ? extends Option> value2,
BiFunction super T1, ? super R1, ? extends Option> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Option> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.iterable(value1)
.iterable(a -> value2.apply(a))
.iterable(a -> b -> value3.apply(a, b))
.iterable(a -> b -> c -> value4.apply(a, b, c))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Option each4(Option extends T1> value1,
Function super T1, ? extends Option> value2,
BiFunction super T1, ? super R1, ? extends Option> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Option> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.iterable(value1)
.iterable(a -> value2.apply(a))
.iterable(a -> b -> value3.apply(a, b))
.iterable(a -> b -> c -> value4.apply(a, b, c))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Option each3(Option extends T1> value1,
Function super T1, ? extends Option> value2,
BiFunction super T1, ? super R1, ? extends Option> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.iterable(value1)
.iterable(a -> value2.apply(a))
.iterable(a -> b -> value3.apply(a, b))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Option each3(Option extends T1> value1,
Function super T1, ? extends Option> value2,
BiFunction super T1, ? super R1, ? extends Option> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.iterable(value1)
.iterable(a -> value2.apply(a))
.iterable(a -> b -> value3.apply(a, b))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Option each2(Option extends T> value1, Function super T, Option> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.iterable(value1)
.iterable(a -> value2.apply(a))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static Option each2(Option extends T> value1, Function super T, ? extends Option> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.iterable(value1)
.iterable(a -> value2.apply(a))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForState {
static State each4(State value1,
Function super T1, ? extends State> value2,
BiFunction super T1, ? super R1, ? extends State> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends State> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(state(value1))
.anyM(a -> state(value2.apply(a)))
.anyM(a -> b -> state(value3.apply(a, b)))
.anyM(a -> b -> c -> state(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static State each4(State value1,
Function super T1, ? extends State> value2,
BiFunction super T1, ? super R1, ? extends State> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends State> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(state(value1))
.anyM(a -> state(value2.apply(a)))
.anyM(a -> b -> state(value3.apply(a, b)))
.anyM(a -> b -> c -> state(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static State each3(State value1,
Function super T1, ? extends State> value2,
BiFunction super T1, ? super R1, ? extends State> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(state(value1))
.anyM(a -> state(value2.apply(a)))
.anyM(a -> b -> state(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static State each3(State value1,
Function super T1, ? extends State> value2,
BiFunction super T1, ? super R1, ? extends State> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(state(value1))
.anyM(a -> state(value2.apply(a)))
.anyM(a -> b -> state(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static State each2(State value1, Function super T, State> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(state(value1))
.anyM(a -> state(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static State each2(State value1,
Function super T, ? extends State> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(state(value1))
.anyM(a -> state(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForEither {
static Either each4(Either value1,
Function super T1, ? extends Either> value2,
BiFunction super T1, ? super R1, ? extends Either> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Either> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(either(value1))
.anyM(a -> either(value2.apply(a)))
.anyM(a -> b -> either(value3.apply(a, b)))
.anyM(a -> b -> c -> either(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Either each4(Either value1,
Function super T1, ? extends Either> value2,
BiFunction super T1, ? super R1, ? extends Either> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Either> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(either(value1))
.anyM(a -> either(value2.apply(a)))
.anyM(a -> b -> either(value3.apply(a, b)))
.anyM(a -> b -> c -> either(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Either each3(Either value1,
Function super T1, ? extends Either> value2,
BiFunction super T1, ? super R1, ? extends Either> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(either(value1))
.anyM(a -> either(value2.apply(a)))
.anyM(a -> b -> either(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Either each3(Either value1,
Function super T1, ? extends Either> value2,
BiFunction super T1, ? super R1, ? extends Either> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(either(value1))
.anyM(a -> either(value2.apply(a)))
.anyM(a -> b -> either(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Either each2(Either value1,
Function super T, Either> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(either(value1))
.anyM(a -> either(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static Either each2(Either value1,
Function super T, ? extends Either> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(either(value1))
.anyM(a -> either(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForReader {
static Reader each4(Reader value1,
Function super T1, ? extends Reader> value2,
BiFunction super T1, ? super R1, ? extends Reader> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Reader> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(reader(value1))
.anyM(a -> reader(value2.apply(a)))
.anyM(a -> b -> reader(value3.apply(a, b)))
.anyM(a -> b -> c -> reader(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Reader each4(Reader value1,
Function super T1, ? extends Reader> value2,
BiFunction super T1, ? super R1, ? extends Reader> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Reader> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(reader(value1))
.anyM(a -> reader(value2.apply(a)))
.anyM(a -> b -> reader(value3.apply(a, b)))
.anyM(a -> b -> c -> reader(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Reader each3(Reader value1,
Function super T1, ? extends Reader> value2,
BiFunction super T1, ? super R1, ? extends Reader> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(reader(value1))
.anyM(a -> reader(value2.apply(a)))
.anyM(a -> b -> reader(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Reader each3(Reader value1,
Function super T1, ? extends Reader> value2,
BiFunction super T1, ? super R1, ? extends Reader> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(reader(value1))
.anyM(a -> reader(value2.apply(a)))
.anyM(a -> b -> reader(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Reader each2(Reader value1,
Function super T, Reader> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(reader(value1))
.anyM(a -> reader(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static Reader each2(Reader value1,
Function super T, ? extends Reader> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(reader(value1))
.anyM(a -> reader(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForWriter {
static Writer each4(Writer value1,
Function super T1, ? extends Writer> value2,
BiFunction super T1, ? super R1, ? extends Writer> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Writer> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(writer(value1))
.anyM(a -> writer(value2.apply(a)))
.anyM(a -> b -> writer(value3.apply(a, b)))
.anyM(a -> b -> c -> writer(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Writer each4(Writer value1,
Function super T1, ? extends Writer> value2,
BiFunction super T1, ? super R1, ? extends Writer> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Writer> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(writer(value1))
.anyM(a -> writer(value2.apply(a)))
.anyM(a -> b -> writer(value3.apply(a, b)))
.anyM(a -> b -> c -> writer(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Writer each3(Writer value1,
Function super T1, ? extends Writer> value2,
BiFunction super T1, ? super R1, ? extends Writer> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(writer(value1))
.anyM(a -> writer(value2.apply(a)))
.anyM(a -> b -> writer(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Writer each3(Writer value1,
Function super T1, ? extends Writer> value2,
BiFunction super T1, ? super R1, ? extends Writer> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(writer(value1))
.anyM(a -> writer(value2.apply(a)))
.anyM(a -> b -> writer(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Writer each2(Writer value1,
Function super T, Writer> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(writer(value1))
.anyM(a -> writer(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static Writer each2(Writer value1,
Function super T, ? extends Writer> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(writer(value1))
.anyM(a -> writer(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForValidation {
static Validation each4(Validation value1,
Function super T1, ? extends Validation> value2,
BiFunction super T1, ? super R1, ? extends Validation> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Validation> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(validation(value1))
.anyM(a -> validation(value2.apply(a)))
.anyM(a -> b -> validation(value3.apply(a, b)))
.anyM(a -> b -> c -> validation(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Validation each4(Validation value1,
Function super T1, ? extends Validation> value2,
BiFunction super T1, ? super R1, ? extends Validation> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Validation> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(validation(value1))
.anyM(a -> validation(value2.apply(a)))
.anyM(a -> b -> validation(value3.apply(a, b)))
.anyM(a -> b -> c -> validation(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Validation each3(Validation value1,
Function super T1, ? extends Validation> value2,
BiFunction super T1, ? super R1, ? extends Validation> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(validation(value1))
.anyM(a -> validation(value2.apply(a)))
.anyM(a -> b -> validation(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Validation each3(Validation value1,
Function super T1, ? extends Validation> value2,
BiFunction super T1, ? super R1, ? extends Validation> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(validation(value1))
.anyM(a -> validation(value2.apply(a)))
.anyM(a -> b -> validation(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Validation each2(Validation value1,
Function super T, Validation> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(validation(value1))
.anyM(a -> validation(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static Validation each2(Validation value1,
Function super T, ? extends Validation> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(validation(value1))
.anyM(a -> validation(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForIO {
static IO each4(IO extends T1> value1,
Function super T1, ? extends IO> value2,
BiFunction super T1, ? super R1, ? extends IO> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends IO> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(io(value1))
.anyM(a -> io(value2.apply(a)))
.anyM(a -> b -> io(value3.apply(a, b)))
.anyM(a -> b -> c -> io(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static IO each4(IO extends T1> value1,
Function super T1, ? extends IO> value2,
BiFunction super T1, ? super R1, ? extends IO> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends IO> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(io(value1))
.anyM(a -> io(value2.apply(a)))
.anyM(a -> b -> io(value3.apply(a, b)))
.anyM(a -> b -> c -> io(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static IO each3(IO extends T1> value1, Function super T1, ? extends IO> value2,
BiFunction super T1, ? super R1, ? extends IO> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(io(value1))
.anyM(a -> io(value2.apply(a)))
.anyM(a -> b -> io(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static IO each3(IO extends T1> value1, Function super T1, ? extends IO> value2,
BiFunction super T1, ? super R1, ? extends IO> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(io(value1))
.anyM(a -> io(value2.apply(a)))
.anyM(a -> b -> io(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static IO each2(IO extends T> value1, Function super T, IO> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(io(value1))
.anyM(a -> io(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static IO each2(IO extends T> value1, Function super T, ? extends IO> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(io(value1))
.anyM(a -> io(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForTrampoline {
static Trampoline each4(Trampoline extends T1> value1,
Function super T1, ? extends Trampoline> value2,
BiFunction super T1, ? super R1, ? extends Trampoline> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Trampoline> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(trampoline(value1))
.anyM(a -> trampoline(value2.apply(a)))
.anyM(a -> b -> trampoline(value3.apply(a, b)))
.anyM(a -> b -> c -> trampoline(value4.apply(a, b, c)))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Trampoline each4(Trampoline extends T1> value1,
Function super T1, ? extends Trampoline> value2,
BiFunction super T1, ? super R1, ? extends Trampoline> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Trampoline> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(trampoline(value1))
.anyM(a -> trampoline(value2.apply(a)))
.anyM(a -> b -> trampoline(value3.apply(a, b)))
.anyM(a -> b -> c -> trampoline(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d))
.yield4(yieldingFunction)
.unwrap())
.unwrap();
}
static Trampoline each3(Trampoline extends T1> value1,
Function super T1, ? extends Trampoline> value2,
BiFunction super T1, ? super R1, ? extends Trampoline> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(trampoline(value1))
.anyM(a -> trampoline(value2.apply(a)))
.anyM(a -> b -> trampoline(value3.apply(a, b)))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Trampoline each3(Trampoline extends T1> value1,
Function super T1, ? extends Trampoline> value2,
BiFunction super T1, ? super R1, ? extends Trampoline> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(trampoline(value1))
.anyM(a -> trampoline(value2.apply(a)))
.anyM(a -> b -> trampoline(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c))
.yield3(yieldingFunction)
.unwrap())
.unwrap();
}
static Trampoline each2(Trampoline extends T> value1,
Function super T, Trampoline> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(trampoline(value1))
.anyM(a -> trampoline(value2.apply(a)))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
static Trampoline each2(Trampoline extends T> value1,
Function super T, ? extends Trampoline> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(trampoline(value1))
.anyM(a -> trampoline(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b))
.yield2(yieldingFunction)
.unwrap())
.unwrap();
}
}
public interface ForList {
static List each4(List extends T1> value1,
Function super T1, ? extends List