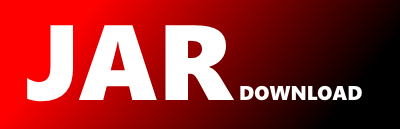
com.aol.cyclops.functionaljava.comprehenders.EitherComprehender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-functionaljava Show documentation
Show all versions of cyclops-functionaljava Show documentation
Converters and Comprehenders for FunctionalJava
package com.aol.cyclops.functionaljava.comprehenders;
import java.util.function.Function;
import java.util.function.Predicate;
import com.aol.cyclops.types.extensability.Comprehender;
import com.aol.cyclops.types.extensability.ValueComprehender;
import fj.data.Either;
import fj.data.Option;
public class EitherComprehender implements ValueComprehender {
public Object filter(Either t, Predicate p) {
return t.right()
.filter(x -> p.test(x));
}
@Override
public Object map(Either t, Function fn) {
return t.right()
.map(e -> fn.apply(e));
}
@Override
public Object flatMap(Either t, Function fn) {
return t.right()
.bind(e -> fn.apply(e));
}
@Override
public Either of(Object o) {
return Either.right(o);
}
@Override
public Either empty() {
return Either.right(Option.none());
}
@Override
public Class getTargetClass() {
return Either.class;
}
public Object resolveForCrossTypeFlatMap(Comprehender comp, Either apply) {
if (apply.isRight())
return comp.of(apply.right()
.value());
return comp.empty();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy