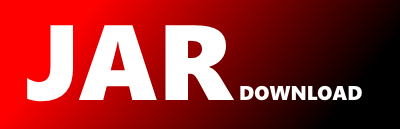
com.aol.cyclops.guava.Guava Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-guava Show documentation
Show all versions of cyclops-guava Show documentation
Converters and Comprehenders for Guava
package com.aol.cyclops.guava;
import java.util.function.BiFunction;
import java.util.function.Function;
import com.aol.cyclops.control.AnyM;
import com.aol.cyclops.control.For;
import com.aol.cyclops.control.Maybe;
import com.aol.cyclops.types.anyM.AnyMSeq;
import com.aol.cyclops.types.anyM.AnyMValue;
import com.aol.cyclops.util.function.QuadFunction;
import com.aol.cyclops.util.function.TriFunction;
import com.google.common.base.Optional;
import com.google.common.collect.FluentIterable;
public class Guava {
public static Maybe asMaybe(Optional option){
return option.isPresent() ? Maybe.just(option.get()) : Maybe.none();
}
/**
*
* {@code
* Guava.optional(Optional.of("hello world"))
.map(String::toUpperCase)
.toSequence()
.toList()
* }
* //[HELLO WORLD]
*
*
* @param optionM to construct AnyM from
* @return AnyM
*/
public static AnyMValue optional(Optional optionM){
return AnyM.ofValue(optionM);
}
/**
*
* {@code
* Guava.anyM(FluentIterable.of(new String[]{"hello world"}))
.map(String::toUpperCase)
.flatMap(i->AnyMonads.anyM(java.util.stream.Stream.of(i)))
.toSequence()
.toList()
* }
* //[HELLO WORLD]
*
*
* @param streamM to construct AnyM from
* @return AnyM
*/
public static AnyMSeq fluentIterable(FluentIterable streamM){
return AnyM.ofSeq(streamM);
}
public interface ForOptional {
static Optional each4(Optional extends T1> value1,
Function super T1, ? extends Optional> value2,
BiFunction super T1, ? super R1, ? extends Optional> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Optional> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(optional(value1)).anyM(a -> optional(value2.apply(a))).anyM(a -> b -> optional(value3.apply(a, b)))
.anyM(a -> b -> c -> optional(value4.apply(a, b, c))).yield4(yieldingFunction).unwrap())
.unwrap();
}
static Optional each4(Optional extends T1> value1,
Function super T1, ? extends Optional> value2,
BiFunction super T1, ? super R1, ? extends Optional> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends Optional> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(optional(value1)).anyM(a -> optional(value2.apply(a)))
.anyM(a -> b -> optional(value3.apply(a, b))).anyM(a -> b -> c -> optional(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d)).yield4(yieldingFunction).unwrap())
.unwrap();
}
static Optional each3(Optional extends T1> value1,
Function super T1, ? extends Optional> value2,
BiFunction super T1, ? super R1, ? extends Optional> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(optional(value1)).anyM(a -> optional(value2.apply(a)))
.anyM(a -> b -> optional(value3.apply(a, b))).yield3(yieldingFunction).unwrap()).unwrap();
}
static Optional each3(Optional extends T1> value1,
Function super T1, ? extends Optional> value2,
BiFunction super T1, ? super R1, ? extends Optional> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM
.ofValue(For.anyM(optional(value1)).anyM(a -> optional(value2.apply(a))).anyM(a -> b -> optional(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c)).yield3(yieldingFunction).unwrap())
.unwrap();
}
static Optional each2(Optional extends T> value1, Function super T, Optional> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(optional(value1)).anyM(a -> optional(value2.apply(a))).yield2(yieldingFunction).unwrap())
.unwrap();
}
static Optional each2(Optional extends T> value1, Function super T, ? extends Optional> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofValue(For.anyM(optional(value1)).anyM(a -> optional(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b)).yield2(yieldingFunction).unwrap()).unwrap();
}
}
public interface ForFluentIterable {
static FluentIterable each4(FluentIterable extends T1> value1,
Function super T1, ? extends FluentIterable> value2,
BiFunction super T1, ? super R1, ? extends FluentIterable> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends FluentIterable> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofSeq(For.anyM(fluentIterable(value1)).anyM(a -> fluentIterable(value2.apply(a))).anyM(a -> b -> fluentIterable(value3.apply(a, b)))
.anyM(a -> b -> c -> fluentIterable(value4.apply(a, b, c))).yield4(yieldingFunction).unwrap())
.unwrap();
}
static FluentIterable each4(FluentIterable extends T1> value1,
Function super T1, ? extends FluentIterable> value2,
BiFunction super T1, ? super R1, ? extends FluentIterable> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends FluentIterable> value4,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, Boolean> filterFunction,
QuadFunction super T1, ? super R1, ? super R2, ? super R3, ? extends R> yieldingFunction) {
return AnyM.ofSeq(For.anyM(fluentIterable(value1)).anyM(a -> fluentIterable(value2.apply(a)))
.anyM(a -> b -> fluentIterable(value3.apply(a, b))).anyM(a -> b -> c -> fluentIterable(value4.apply(a, b, c)))
.filter(a -> b -> c -> d -> filterFunction.apply(a, b, c, d)).yield4(yieldingFunction).unwrap())
.unwrap();
}
static FluentIterable each3(FluentIterable extends T1> value1,
Function super T1, ? extends FluentIterable> value2,
BiFunction super T1, ? super R1, ? extends FluentIterable> value3,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM.ofSeq(For.anyM(fluentIterable(value1)).anyM(a -> fluentIterable(value2.apply(a)))
.anyM(a -> b -> fluentIterable(value3.apply(a, b))).yield3(yieldingFunction).unwrap()).unwrap();
}
static FluentIterable each3(FluentIterable extends T1> value1,
Function super T1, ? extends FluentIterable> value2,
BiFunction super T1, ? super R1, ? extends FluentIterable> value3,
TriFunction super T1, ? super R1, ? super R2, Boolean> filterFunction,
TriFunction super T1, ? super R1, ? super R2, ? extends R> yieldingFunction) {
return AnyM
.ofSeq(For.anyM(fluentIterable(value1)).anyM(a -> fluentIterable(value2.apply(a))).anyM(a -> b -> fluentIterable(value3.apply(a, b)))
.filter(a -> b -> c -> filterFunction.apply(a, b, c)).yield3(yieldingFunction).unwrap())
.unwrap();
}
static FluentIterable each2(FluentIterable extends T> value1, Function super T, FluentIterable> value2,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofSeq(For.anyM(fluentIterable(value1)).anyM(a -> fluentIterable(value2.apply(a))).yield2(yieldingFunction).unwrap())
.unwrap();
}
static FluentIterable each2(FluentIterable extends T> value1, Function super T, ? extends FluentIterable> value2,
BiFunction super T, ? super R1, Boolean> filterFunction,
BiFunction super T, ? super R1, ? extends R> yieldingFunction) {
return AnyM.ofSeq(For.anyM(fluentIterable(value1)).anyM(a -> fluentIterable(value2.apply(a)))
.filter(a -> b -> filterFunction.apply(a, b)).yield2(yieldingFunction).unwrap()).unwrap();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy