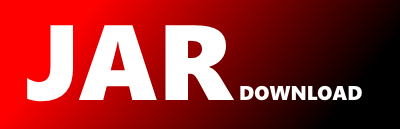
com.aol.cyclops.javaslang.Javaslang Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-javaslang Show documentation
Show all versions of cyclops-javaslang Show documentation
Converters and Comprehenders for Javaslang
package com.aol.cyclops.javaslang;
import java.util.Optional;
import javaslang.collection.List;
import javaslang.collection.Stream;
import javaslang.control.Either;
import javaslang.control.Either.LeftProjection;
import javaslang.control.Either.RightProjection;
import javaslang.control.Option;
import javaslang.control.Right;
import javaslang.control.Try;
import javaslang.test.Arbitrary;
import com.aol.cyclops.lambda.api.AsAnyM;
import com.aol.cyclops.monad.AnyM;
public class Javaslang {
public static AnyM anyM(Arbitrary arbM){
return AsAnyM.notTypeSafeAnyM(arbM);
}
public static AnyM anyM(Try tryM){
return AsAnyM.notTypeSafeAnyM(tryM);
}
public static AnyM anyMFailure(Try tryM){
if(tryM.isFailure())
return AsAnyM.notTypeSafeAnyM(Try.of(()->tryM.toEither().left().get()));
return AsAnyM.notTypeSafeAnyM(Optional.empty());
}
public static AnyM anyM(Either,T> tryM){
return AsAnyM.notTypeSafeAnyM(tryM);
}
public static AnyM anyM(RightProjection,T> tryM){
if(tryM.toJavaOptional().isPresent())
return AsAnyM.notTypeSafeAnyM(new Right(tryM.get()));
else
return AsAnyM.notTypeSafeAnyM(Optional.empty());
}
public static AnyM anyM(LeftProjection tryM){
if(tryM.toJavaOptional().isPresent())
return AsAnyM.notTypeSafeAnyM(new Right(tryM.get()));
else
return AsAnyM.notTypeSafeAnyM(Optional.empty());
}
public static AnyM anyM(Option tryM){
return AsAnyM.notTypeSafeAnyM(tryM);
}
public static AnyM anyM(Stream tryM){
return AsAnyM.notTypeSafeAnyM(tryM);
}
public static AnyM anyM(List tryM){
return AsAnyM.notTypeSafeAnyM(tryM);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy