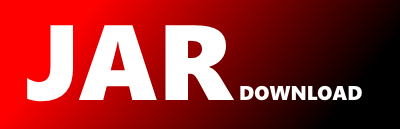
com.aol.cyclops.streams.ToLazyCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-streams Show documentation
Show all versions of cyclops-streams Show documentation
Sequential Streams and Stream Utilities for Java 8
package com.aol.cyclops.streams;
import java.util.AbstractCollection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.locks.ReentrantLock;
import java.util.stream.Stream;
public class ToLazyCollection {
/**
* Projects an immutable collection of this stream. Initial iteration over the collection is not thread safe
* (can't be performed by multiple threads concurrently) subsequent iterations are.
*
* @return An immutable collection of this stream.
*/
public static final Collection toLazyCollection(Stream stream) {
return toLazyCollection(stream.iterator());
}
public static final Collection toLazyCollection(Iterator iterator){
return toLazyCollection(iterator,false);
}
/**
* Lazily constructs a Collection from specified Stream. Collections iterator may be safely used
* concurrently by multiple threads.
* @param stream
* @return
*/
public static final Collection toConcurrentLazyCollection(Stream stream) {
return toConcurrentLazyCollection(stream.iterator());
}
public static final Collection toConcurrentLazyCollection(Iterator iterator){
return toLazyCollection(iterator,true);
}
private static final Collection toLazyCollection(Iterator iterator,boolean concurrent) {
return new AbstractCollection() {
@Override
public boolean equals(Object o){
if(o==null)
return false;
if(! (o instanceof Collection))
return false;
Collection c = (Collection)o;
Iterator it1 = iterator();
Iterator it2 = c.iterator();
while(it1.hasNext()){
if(!it2.hasNext())
return false;
if(!Objects.equals(it1.next(),it2.next()))
return false;
}
if(it2.hasNext())
return false;
return true;
}
@Override
public int hashCode(){
Iterator it1 = iterator();
List arrayList= new ArrayList<>();
while(it1.hasNext()){
arrayList.add(it1.next());
}
return Objects.hashCode(arrayList.toArray());
}
List data =new ArrayList<>();
volatile boolean complete=false;
Object lock = new Object();
ReentrantLock rlock = new ReentrantLock();
public Iterator iterator() {
if(complete)
return data.iterator();
return new Iterator(){
int current = -1;
@Override
public boolean hasNext() {
if(concurrent){
rlock.lock();
}
try{
if(current==data.size()-1 && !complete){
boolean result = iterator.hasNext();
complete = !result;
return result;
}
if(current+1
© 2015 - 2024 Weber Informatics LLC | Privacy Policy