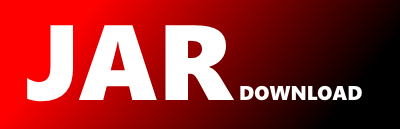
com.aol.cyclops.streams.future.FutureOperationsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-streams Show documentation
Show all versions of cyclops-streams Show documentation
Sequential Streams and Stream Utilities for Java 8
package com.aol.cyclops.streams.future;
import java.util.Collection;
import java.util.Comparator;
import java.util.DoubleSummaryStatistics;
import java.util.IntSummaryStatistics;
import java.util.List;
import java.util.LongSummaryStatistics;
import java.util.Map;
import java.util.Optional;
import java.util.OptionalDouble;
import java.util.OptionalInt;
import java.util.OptionalLong;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executor;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.BinaryOperator;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.IntFunction;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.function.ToDoubleFunction;
import java.util.function.ToIntFunction;
import java.util.function.ToLongFunction;
import java.util.stream.Collector;
import lombok.AllArgsConstructor;
import lombok.Getter;
import com.aol.cyclops.sequence.SequenceM;
import com.aol.cyclops.sequence.future.FutureOperations;
@AllArgsConstructor
public class FutureOperationsImpl implements FutureOperations, DoubleOperatorsMixin,
IntOperatorsMixin, LongOperatorsMixin {
@Getter
private final Executor exec;
@Getter
private final SequenceM stream;
/**
* Asynchronously convert this Stream into a List
*
* {@code
* CompletableFuture> myList = EagerFutureStream.of(1,2,3,4)
* .map(this::loadFromDb)
* .withTaskExecutor(parallelBuilder().getExecutor())
* .map(this::processOnDifferentExecutor)
* .toList();
* }
*
*
* @return Future List
*/
public CompletableFuture> toList(){
return CompletableFuture.supplyAsync(()->stream.toList(),exec);
}
/**
* @return Last value in this Stream (must be non-empty)
*/
public CompletableFuture lastValue(){
return CompletableFuture.supplyAsync(()->{ List l= stream.toList(); return l.get(l.size()-1);},exec);
}
/**
* @return the only entry in this Stream if it is a single entry Stream,
* otherwise throws an UnsupportedOperationException
*/
public CompletableFuture single(){
return CompletableFuture.supplyAsync(()->{ List l= stream.toList(); if(l.size()==1){ return l.get(l.size()-1); }
throw new UnsupportedOperationException("single only works for Streams with a single value");},exec);
}
/**
* Asynchronously convert this Stream into a List
*
* {@code
* CompletableFuture> myList = LazyFutureStream.of(1,2,3,4)
* .map(this::loadFromDb)
* .withTaskExecutor(parallelBuilder().getExecutor())
* .map(this::processOnDifferentExecutor)
* .toSet();
* }
*
*
* @return Future Set
*/
public CompletableFuture> toSet(){
return CompletableFuture.supplyAsync(()->stream.toSet(),exec);
}
/**
* Asynchronously capture the minimum value in this stream using the provided function
*
* @see org.jooq.lambda.Seq#minBy(Function)
*/
public > CompletableFuture> minBy(Function function){
return CompletableFuture.supplyAsync(()->stream.minBy(function));
}
/**
* Asynchronously capture the maximum value in this stream using the provided function
*
* @see org.jooq.lambda.Seq#maxBy(Function)
*/
public > CompletableFuture> maxBy(Function function){
return CompletableFuture.supplyAsync(()->stream.maxBy(function));
}
/**
* Asynchronously perform a Stream collection
*
* @see java.util.stream.Stream#collect(Collector)
*
*/
public CompletableFuture collect(Collector super T, A, R> collector){
return CompletableFuture.supplyAsync(()->stream.collect(collector));
}
/**
* Asynchronously perform a Stream collection
* @see org.jooq.lambda.Seq#toCollection(Supplier)
*/
public > CompletableFuture toCollection(Supplier collectionFactory){
return CompletableFuture.supplyAsync(()->stream.toCollection(collectionFactory),exec);
}
/**
* Asyncrhonously generate an Array
*
* @see java.util.stream.Stream#toArray(IntFunction)
*/
public CompletableFuture toArray(IntFunction generator){
return CompletableFuture.supplyAsync(()->stream.toArray(generator),exec);
}
/**
* Asyncrhonously generate an Array
*
* @see java.util.stream.Stream#toArray(IntFunction)
*/
public CompletableFuture
© 2015 - 2024 Weber Informatics LLC | Privacy Policy