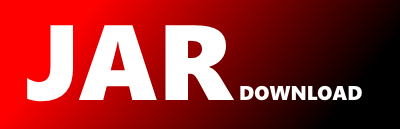
com.aol.cyclops.streams.operators.BatchWhileOperator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-streams Show documentation
Show all versions of cyclops-streams Show documentation
Sequential Streams and Stream Utilities for Java 8
package com.aol.cyclops.streams.operators;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
import lombok.Value;
import com.aol.cyclops.streams.StreamUtils;
@Value
public class BatchWhileOperator> {
private static final Object UNSET = new Object();
Stream stream;
Supplier factory;
public BatchWhileOperator(Stream stream){
this.stream = stream;
factory = ()-> (C)new ArrayList<>();
}
public BatchWhileOperator(Stream stream, Supplier factory) {
super();
this.stream = stream;
this.factory = factory;
}
public Stream batchWhile(Predicate predicate){
Iterator it = stream.iterator();
return StreamUtils.stream(new Iterator(){
T value = (T)UNSET;
@Override
public boolean hasNext() {
return value!=UNSET || it.hasNext();
}
@Override
public C next() {
C list = factory.get();
if(value!=UNSET)
list.add(value);
T value;
label: while(it.hasNext()) {
value=it.next();
list.add(value);
if(!predicate.test(value)){
value=(T)UNSET;
break label;
}
value=(T)UNSET;
}
return list;
}
}).filter(l->l.size()>0);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy