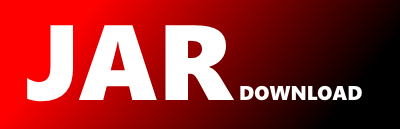
com.aol.cyclops.streams.spliterators.ClosingSpliterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-streams Show documentation
Show all versions of cyclops-streams Show documentation
Sequential Streams and Stream Utilities for Java 8
package com.aol.cyclops.streams.spliterators;
import java.util.Objects;
import java.util.Queue;
import java.util.Spliterator;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.Consumer;
public class ClosingSpliterator implements Spliterator {
private long estimate;
private final Queue queue;
private final AtomicBoolean open;
public ClosingSpliterator(long estimate,
Queue queue, AtomicBoolean open) {
this.estimate = estimate;
this.open = open;
this.queue = queue;
}
@Override
public long estimateSize() {
return estimate;
}
@Override
public int characteristics() {
return IMMUTABLE;
}
@Override
public boolean tryAdvance(Consumer super T> action) {
Objects.requireNonNull(action);
if(!open.get() && queue.size()==0){
System.out.println(open.get() + " : " + queue.size());
return false;
}
T value;
if((value=queue.poll())!=null)
action.accept(nullSafe(value));
return true;
}
private T nullSafe(T value) {
return value;
}
@Override
public Spliterator trySplit() {
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy