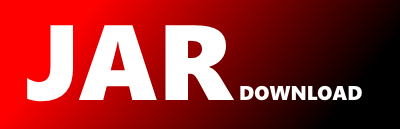
com.aol.cyclops.trampoline.Trampoline Maven / Gradle / Ivy
package com.aol.cyclops.trampoline;
import java.util.stream.Stream;
/**
* simple Trampoline implementation : inspired by excellent TotallyLazy Java 8 impl
* and Mario Fusco presentation
*
* @author johnmcclean
*
* @param Return type
*/
public interface Trampoline {
/**
* @return next stage in Trampolining
*/
default Trampoline bounce(){
return this;
}
/**
* @return The result of Trampoline execution
*/
T result();
/**
* @return true if complete
*
*/
default boolean complete() {
return true;
}
/**
* Created a completed Trampoline
*
* @param result Completed result
* @return Completed Trampoline
*/
public static Trampoline done(T result) {
return () -> result;
}
/**
* Create a Trampoline that has more work to do
*
* @param trampoline Next stage in Trampoline
* @return Trampoline with more work
*/
public static Trampoline more(Trampoline> trampoline) {
return new Trampoline() {
@Override
public boolean complete() {
return false;
}
@Override
public Trampoline bounce() {
return trampoline.result();
}
public T result() {
return trampoline(this);
}
T trampoline(Trampoline trampoline) {
return Stream.iterate(trampoline,Trampoline::bounce)
.filter(Trampoline::complete)
.findFirst()
.get()
.result();
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy