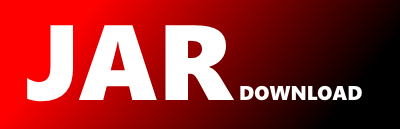
com.aol.cyclops.vavr.adapter.TryAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyclops-vavr Show documentation
Show all versions of cyclops-vavr Show documentation
Converters and Comprehenders for Javaslang
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy