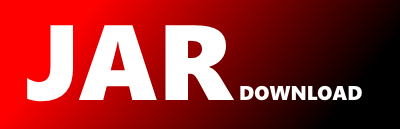
com.aol.micro.server.events.RequestsBeingExecuted Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of micro-events Show documentation
Show all versions of micro-events Show documentation
Opinionated rest microservices
package com.aol.micro.server.events;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import org.springframework.beans.factory.annotation.Qualifier;
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.experimental.Builder;
public class RequestsBeingExecuted {
private final EventBus bus;
private final ActiveEvents> events = new ActiveEvents();
@Getter
private final String type;
public RequestsBeingExecuted(@Qualifier("microserverEventBus") EventBus bus, boolean queryCapture) {
this.bus = bus;
this.type = "default";
if (queryCapture)
bus.register(this);
}
public RequestsBeingExecuted(EventBus bus, String type) {
this.bus = bus;
this.type = type;
bus.register(this);
}
public int events() {
return events.events();
}
public int size() {
return events.size();
}
@Override
public String toString() {
return events.toString();
}
@Subscribe
public void finished(RemoveQuery data) {
if (type.equals(data.getData().type))
events.finished(buildId(data.getData()));
}
@Subscribe
public void processing(AddQuery data) {
if (type.equals(data.getData().type)) {
String id = buildId(data.getData());
events.active(id, data.getData());
}
}
private String buildId(RequestData data) {
String id = "" + data.correlationId;
return id;
}
public static class AddQuery extends AddEvent> {
public AddQuery(RequestData data) {
super(
data);
}
}
public static class RemoveQuery extends RemoveEvent> {
public RemoveQuery(RequestData data) {
super(
data);
}
}
@AllArgsConstructor
@Builder
@XmlAccessorType(XmlAccessType.FIELD)
@Getter
public static class RequestData extends BaseEventInfo {
private final long correlationId;
private final T query;
private final String type;
private final Object additionalData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy