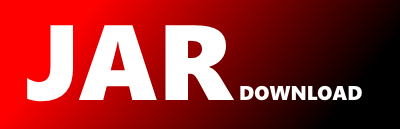
hope.kola.contract.Configuration Maven / Gradle / Ivy
package hope.kola.contract;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.api.ObjectFactory;
import hope.kola.contract.restassured.RestConfiguration;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Consumer;
import org.apache.commons.lang3.StringUtils;
public class Configuration implements ObjectFactory {
/** Avoid stupid guide name conflict */
public static final String COMMON = "__common__";
private final String env;
protected Map properties = new LinkedHashMap<>();
private String baseURI;
private Integer port;
private String basePath;
private RestConfiguration rest;
public Configuration(String env) {
this.env = env;
}
/**
* Point of entry to build a Configuration.
*
* @param consumer function to manipulate the Configuration
* @return manipulated Configuration
*/
public static Configuration env(final String name, final Consumer consumer) {
final Configuration config = new Configuration(name);
consumer.accept(config);
return config;
}
/**
* Groovy point of entry to build a Configuration.
*
* @param closure function to manipulate the Configuration
* @return manipulated Configuration
*/
public static Configuration env(
final String name, @DelegatesTo(Configuration.class) Closure closure) {
Configuration config = new Configuration(name);
closure.setDelegate(config);
closure.call();
return config;
}
/**
* default shared base(common), With default name of {@link #COMMON}, which is {@code __common__},
* make sure you NEVER take this name!
*
* @param closure function to manipulate the Configuration
* @return manipulated Configuration
*/
public static Configuration common(@DelegatesTo(Configuration.class) Closure closure) {
Configuration config = new Configuration(COMMON);
closure.setDelegate(config);
closure.call();
return config;
}
public String basePath() {
return basePath;
}
/**
* Set base path of this env configuration
*
* @param basePath base Path
*/
public void basePath(String basePath) {
this.basePath = basePath;
}
public String baseURI() {
return baseURI;
}
/**
* base url of this environment default is {@link io.restassured.RestAssured#DEFAULT_URI}
*
* @param baseURI
*/
public void baseURI(String baseURI) {
this.baseURI = baseURI;
}
public Integer port() {
return port;
}
/**
* port of this environment default is {@link io.restassured.RestAssured#DEFAULT_PORT}
*
* @param port
*/
public void port(Integer port) {
this.port = port;
}
/** Let the framework decide the port */
public void defaultPort() {
this.port = -1;
}
public String env() {
return env;
}
/**
* define property with key and value;
*
* They can be accessed within your tester context by call {@code $V(key)}
*
*
for example:
*
*
*
*
* p("magicNumber", 42)
* p("name", "jake")
*
*
*
*
* then you can in your Feature define access like:
*
*
*
*
* json (
* """
* {
* "name": "$V('hello')"
* "age": "$V('magicNumber')"
* }
* """
* )
*
*
*
*
* @param name name of this property
* @param value any kind {@link Object}
*/
public void p(final String name, final Object value) {
properties.put(name, value);
}
/**
* get all the properties
*
* @return
*/
public Map properties() {
return properties;
}
public void rest(@DelegatesTo(RestConfiguration.class) Closure consumer) {
if (rest == null) {
rest = new RestConfiguration();
}
consumer.setDelegate(rest);
consumer.call();
}
public RestConfiguration rest() {
return rest;
}
/**
* From has high priority:
*
* @param from another Configuration
* @return a new Configuration
*/
public Configuration merger(Configuration from) {
final Configuration merger = new Configuration(env + " " + from.env);
merger.basePath = basePath;
merger.baseURI = baseURI;
merger.port = port;
merger.properties.putAll(properties);
merger.rest = rest;
if (StringUtils.isNotBlank(from.basePath)) {
merger.basePath = from.basePath;
}
if (StringUtils.isNotBlank(from.baseURI)
&& !from.baseURI.equals(io.restassured.RestAssured.DEFAULT_URI)) {
merger.baseURI = from.baseURI;
}
if (from.port != null) {
merger.port = from.port;
}
if (from.rest != null) {
if (rest == null) {
merger.rest = from.rest;
} else {
merger.rest = rest.merger(from.rest);
}
}
// Merger properties:
merger.properties.putAll(from.properties);
return merger;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy