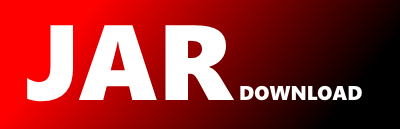
hope.kola.contract.Feature Maven / Gradle / Ivy
package hope.kola.contract;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.validation.Validatable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
public class Feature implements Validatable {
/** Mapping of metadata. Can be used for external integrations. */
private final Map metadata = new HashMap<>();
protected String name;
protected String description;
protected boolean ignore = false;
protected Tags tags = new Tags();
protected Background background;
protected List scenarios = new ArrayList<>();
/**
* You can set the level of priority of this feature. If there are two features mapped for example
* to the same endpoint, then the one with greater priority should take precedence. A priority of
* 1 is highest and takes precedence over a priority of 2.
*/
private Integer priority;
/**
* Whether the feature is in progress. It's not ignored, but the feature is not yet finished. Used
* together with the {@code generateStubs} option.
*/
private boolean inProgress;
/**
* Point of entry to build a feature.
*
* @param consumer function to manipulate the feature
* @return manipulated feature
*/
public static Feature make(Consumer consumer) {
Feature feature = new Feature();
consumer.accept(feature);
return feature;
}
/**
* Groovy point of entry to build a Feature. Left for backward compatibility reasons.
*
* @param closure function to manipulate the Feature
* @return manipulated Feature
*/
public static Feature make(@DelegatesTo(Feature.class) Closure closure) {
Feature feature = new Feature();
closure.setDelegate(feature);
closure.call();
// TODO verify the feature is validated
return feature;
}
/**
* Active base on specific tag(s), any tag match will execute this feature
*
* @param tags list of tag name
*/
public void enable(final String... tags) {
this.tags.enable(tags);
}
/**
* Ignore base on specific tag(s), any tag match will ignore this feature
*
* @param tags list of tag name
*/
public void disable(final String... tags) {
this.tags.disable(tags);
}
/**
* Add a new Scenario to this feature
*
* @param name the name of this scenario* required
* @param consumer function to manipulate the Scenario
*/
public void Scenario(String name, @DelegatesTo(Scenario.class) Closure consumer) {
Scenario scenario = new Scenario(this, scenarios.size());
scenario.setName(name);
consumer.setDelegate(scenario);
consumer.call();
this.scenarios.add(scenario);
}
/**
* Add a new Scenario to this feature
*
* @param consumer function to manipulate the Scenario
*/
public void Scenario(@DelegatesTo(Scenario.class) Closure consumer) {
Scenario scenario = new Scenario(this, scenarios.size());
consumer.setDelegate(scenario);
consumer.call();
this.scenarios.add(scenario);
}
/**
* interface for java to add {@link Scenario}
*
* @param scenario the target {@link Scenario}
*/
public void setScenario(final Scenario scenario) {
this.scenarios.add(scenario);
}
/**
* Add {@link Background} to this feature
*
* @param description description of this background
* @param consumer function to manipulate the {@link Background}
*/
public void Background(String description, @DelegatesTo(Background.class) Closure consumer) {
this.background = new Background();
background.description(description);
consumer.setDelegate(background);
consumer.call();
}
/**
* Get all the backgrounds of this feature
*
* @return background
*/
public Background getBackground() {
return background;
}
/**
* (JAVA) Add background to this feature
*
* @param background {@link Background} of this Feature
*/
public void setBackground(final Background background) {
this.background = background;
}
/**
* Get all the scenario of this feature
*
* @return list of Scenario
*/
public List getScenarios() {
return scenarios;
}
/**
* Add {@link Background} to this feature
*
* @param consumer function to manipulate the {@link Background}
*/
public void Background(@DelegatesTo(Background.class) Closure consumer) {
this.background = new Background();
consumer.setDelegate(background);
consumer.call();
}
// -------------------------Each pieces =================BEGIN
public Integer getPriority() {
return priority;
}
/**
* You can set the level of priority of this contract. If there are two contracts mapped for
* example to the same endpoint, then the one with greater priority should take precedence. A
* priority of 1 is highest and takes precedence over a priority of 2.
*
* @param priority the higher the value the lower the priority
*/
public void priority(int priority) {
this.priority = priority;
}
public String getName() {
return name;
}
/**
* the name of this feature
*
* @param name easy to pick
*/
public void name(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
/**
* set the description of this feature;
*
* @param description what this feature do
*/
public void description(String description) {
this.description = description;
}
public boolean isIgnore() {
return ignore;
}
public void ignore() {
this.ignore = true;
}
public Tags getTags() {
return tags;
}
public boolean isInProgress() {
return inProgress;
}
public void inProgress() {
this.inProgress = true;
}
public Map getMetadata() {
return metadata;
}
public boolean hasTags() {
return tags != null && !tags.isEmpty();
}
// -------------------------Each pieces =================END
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy