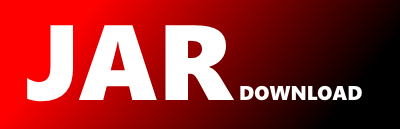
hope.kola.contract.Scenario Maven / Gradle / Ivy
package hope.kola.contract;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.api.*;
import hope.kola.contract.validation.Validatable;
public class Scenario implements Validatable {
private final Feature feature;
private final int index;
private String name;
private String description;
private Tags tags = new Tags();
private Variables variables;
// Those for he BDD == BEGIN
private GivenApi given;
private WhenRequest when;
private ThenVerification then;
// Those for he BDD == END
private ScriptFragment postScript;
private ScriptFragment preScript;
public Scenario(Feature feature, final int index) {
this.feature = feature;
this.index = index;
}
public Feature getFeature() {
return feature;
}
public int getIndex() {
return index;
}
public String name() {
return name;
}
public void setName(final String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public Scenario setDescription(String description) {
this.description = description;
return this;
}
/**
* Active base on specific tag(s), any tag match will execute this feature
*
* @param tags list of tag name
*/
public void enable(final String... tags) {
this.tags.enable(tags);
}
/**
* Ignore base on specific tag(s), any tag match will ignore this feature
*
* @param tags list of tag name
*/
public void disable(final String... tags) {
this.tags.disable(tags);
}
public Tags getTags() {
return tags;
}
public Scenario setTags(Tags tags) {
this.tags = tags;
return this;
}
public Variables getVariables() {
return variables;
}
public Scenario setVariables(Variables variables) {
this.variables = variables;
return this;
}
public GivenApi getGiven() {
return given;
}
/**
* Given a specific API context
*
* @param consumer function to manipulate the API information
*/
public void Given(@DelegatesTo(GivenApi.class) Closure consumer) {
this.given = new GivenApi();
consumer.setDelegate(given);
consumer.call();
}
/**
* (JAVA)Given a specific API context
*
* @param given {@link GivenApi}
*/
public void setGiven(GivenApi given) {
this.given = given;
}
public WhenRequest getWhen() {
return when;
}
/**
* When Composite the Request
*
* @param consumer function to manipulate the Request
*/
public void When(@DelegatesTo(WhenRequest.class) Closure consumer) {
this.when = new WhenRequest();
consumer.setDelegate(when);
consumer.call();
}
/**
* (JAVA) When Composite the Request
*
* @param request {@link WhenRequest}
*/
public void setWhen(final WhenRequest request) {
this.when = request;
}
public ThenVerification getThen() {
return then;
}
/**
* {@code Server} side test logic
* And Verify logic base on the Response
*
* @param consumer function to verify the Response
*/
@Deprecated
public void And(@DelegatesTo(ThenVerification.class) Closure consumer) {
this.then = new ThenVerification();
consumer.setDelegate(then);
consumer.call();
}
public void Then(@DelegatesTo(ThenVerification.class) Closure consumer) {
this.then = new ThenVerification();
consumer.setDelegate(then);
consumer.call();
}
/**
* (JAVA) {@code Server} side test logic
* And Verify logic base on the Response
*
* @param and {@link ThenVerification}
*/
public void setThen(final ThenVerification then) {
this.then = then;
}
public ScriptFragment getPostScript() {
return postScript;
}
/**
* Last piece of code of this {@link Scenario} may perform:
*
* 1. clean up job
* 2. global env manipulate
*
*
* @param consumer function to write dynamic code
*/
public void postScript(@DelegatesTo(ScriptFragment.class) Closure consumer) {
this.postScript = new ScriptFragment();
consumer.setDelegate(postScript);
consumer.call();
}
/**
* (JAVA) Last piece of code of this {@link Scenario} may perform:
*
* 1. clean up job
* 2. global env manipulate
*
*
* @param postScript {@link ScriptFragment}
*/
public void setPostScript(final ScriptFragment postScript) {
this.postScript = postScript;
}
public ScriptFragment getPreScript() {
return preScript;
}
/**
* First piece of code of this {@link Scenario} may perform:
*
* 1. prepare job
* 2. global env manipulate
*
*
* @param consumer function to write dynamic code
*/
public void preScript(@DelegatesTo(ScriptFragment.class) Closure consumer) {
this.preScript = new ScriptFragment();
consumer.setDelegate(preScript);
consumer.call();
}
/**
* (JAVA) First piece of code of this {@link Scenario} may perform:
*
* 1. prepare job
* 2. global env manipulate
*
*
* @param preScript {@link ScriptFragment}
*/
public void setPreScript(ScriptFragment preScript) {
this.preScript = preScript;
}
public boolean hasTags() {
return tags != null && !tags.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy