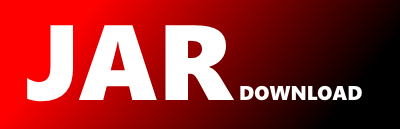
hope.kola.contract.Variable Maven / Gradle / Ivy
package hope.kola.contract;
import com.squareup.javapoet.*;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.assertj.model.DynamicProperty;
import hope.kola.contract.assertj.model.Factory;
import hope.kola.contract.assertj.model.PrimitiveType;
import hope.kola.contract.exception.FeatureParseException;
import java.util.List;
import java.util.Map;
@SuppressWarnings("Duplicates")
public class Variable extends DynamicProperty {
protected final String name;
/** if not Pre-defined type then customized */
protected String clz;
protected String clz2;
protected CodeBlock.Builder initiator;
protected boolean noInitiator;
public Variable(String name) {
this.name = name;
}
public void valueType(final Class clz) {
type = PrimitiveType.MAP;
clz2 = clz.getCanonicalName();
}
public void noInitiator() {
noInitiator = true;
}
/**
* (JAVA)the initiator of this Variable;
* This Closure's build must with a {@code return res} otherwise runtime will break
*
* @param initiator script for define value initiator
*/
public void initWith(final CodeBlock.Builder initiator) {
this.initiator = initiator;
}
/**
* the initiator of this Variable;
* This Closure's build must with a {@code return res} otherwise runtime will break
*
* @param consumer function to define how to init this Variable
*/
public void initWith(@DelegatesTo(CodeBlock.Builder.class) Closure consumer) {
initiator = CodeBlock.builder();
consumer.setDelegate(initiator);
consumer.call();
}
public void build(TypeSpec.Builder clzBuilder) {
FieldSpec.Builder fb = declarePart();
initPart(fb);
clzBuilder.addField(fb.build());
}
private FieldSpec.Builder declarePart() {
if (type != null) {
if (type == PrimitiveType.LIST || isPlural()) {
// List>
return FieldSpec.builder(
ParameterizedTypeName.get(ClassName.get(List.class), type.className()), name);
} else if (type == PrimitiveType.MAP) {
// Map,?>
return FieldSpec.builder(
ParameterizedTypeName.get(
ClassName.get(Map.class), ClassName.bestGuess(clz), ClassName.bestGuess(clz2)),
name);
} else {
// Raw Type
return FieldSpec.builder(type.className(), name);
}
} else {
if (clz != null) {
if (clz2 != null) {
// This is a MAP
return FieldSpec.builder(
ParameterizedTypeName.get(
ClassName.get(Map.class), ClassName.bestGuess(clz), ClassName.bestGuess(clz2)),
name);
} else {
if (isPlural()) {
return FieldSpec.builder(
ParameterizedTypeName.get(ClassName.get(List.class), ClassName.bestGuess(clz)),
name);
} else {
return FieldSpec.builder(ClassName.bestGuess(clz), name);
}
}
} else {
throw new FeatureParseException(
"Variable can not judge type and the type is null and clz and clz2 all blank ");
}
}
}
private void initPart(FieldSpec.Builder fb) {
if (initiator != null) {
/**
*
* Student x = ((Factory) () -> {
* Student res1 = new Student();
* res1.setName("jake");
* return res1;
* }).create();
*
*/
CodeBlock.Builder init = CodeBlock.builder();
init.add("(($T<$T>)()->{\n\n", Factory.class, ClassName.bestGuess(clz));
init.add("$L", initiator.build());
init.add("}).create()");
fb.initializer(init.build());
return;
}
if (code != null) {
fb.initializer(code.build());
} else {
// Let it go NOTHING to init
}
}
public String clz() {
if (type != null) {
return type().clz().getCanonicalName();
}
return clz == null ? Object.class.getCanonicalName() : clz;
}
public void clz(String clz) {
this.clz = clz;
}
public void clz(Class clz) {
this.clz = clz.getCanonicalName();
}
public String valueClz() {
return clz2;
}
public void valueClz(String clz2) {
this.clz2 = clz2;
}
public String name() {
return name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy