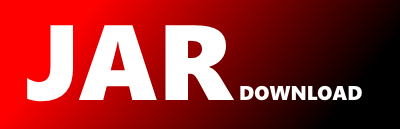
hope.kola.contract.api.DirectCall Maven / Gradle / Ivy
package hope.kola.contract.api;
import hope.kola.contract.validation.Validatable;
import org.springframework.util.Assert;
public class DirectCall implements Validatable {
protected String path;
/** default as {@link HttpMethod#GET} */
protected HttpMethod method = HttpMethod.GET;
public String getPath() {
return path;
}
/**
* Set the path of this request
*
* @param path request path
*/
public void path(String path) {
Assert.hasText(path, "path can not be blank");
this.path = path;
}
/** Mark request as {@link HttpMethod#POST} */
public void post(final String path) {
this.path = path;
method = HttpMethod.POST;
}
/** Mark request as {@link HttpMethod#GET} */
public void get(final String path) {
this.path = path;
method = HttpMethod.GET;
}
/** Mark request as {@link HttpMethod#PUT} */
public void put(String path) {
this.path = path;
method = HttpMethod.PUT;
}
/** Mark request as {@link HttpMethod#DELETE} */
public void delete(String path) {
this.path = path;
method = HttpMethod.DELETE;
}
/** Mark request as {@link HttpMethod#HEAD} */
public void head(String path) {
this.path = path;
method = HttpMethod.HEAD;
}
/** Mark request as {@link HttpMethod#PATCH} */
public void patch(final String path) {
this.path = path;
method = HttpMethod.PATCH;
}
/**
* Get request method
*
* @return {@link HttpMethod}
*/
public HttpMethod getMethod() {
return method;
}
/**
* set the request method
*
* @param method {@link HttpMethod}
*/
public void method(HttpMethod method) {
this.method = method;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy