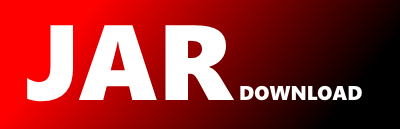
hope.kola.contract.api.GivenApi Maven / Gradle / Ivy
package hope.kola.contract.api;
import hope.kola.contract.validation.Validatable;
import org.springframework.util.Assert;
public class GivenApi implements Validatable {
/** The Service information of this API example: {@code com.example.UserService} */
protected String service;
/**
* The Method information of this API example: {@code LoginRequest} of {@code
* com.example.UserService#LoginRequest}
*/
protected String action;
/** Direct call from a {@link java.net.URL}, not {@code ApiHug} style */
protected DirectCall direct;
public String getService() {
return service;
}
/**
* {@code ApiHug} style API service: {@code com.example.UserService#LoginRequest}
*
* @param service the name of this service {@code com.example.UserService}
* @param action the action of this service {@code LoginRequest}
*/
public void api(final String service, final String action) {
Assert.hasText(service, "Service can not be blank");
Assert.hasText(action, "Action can not be blank");
this.service = service;
this.action = action;
direct = null;
}
public String getAction() {
return action;
}
public DirectCall direct() {
return direct;
}
/** {@link DirectCall} post to this given path */
public void post(final String path) {
this.direct = new DirectCall();
direct.post(path);
}
/** {@link DirectCall} get this given path */
public void get(final String path) {
this.direct = new DirectCall();
direct.get(path);
}
/** {@link DirectCall} put to this given path */
public void put(String path) {
this.direct = new DirectCall();
direct.put(path);
}
/** {@link DirectCall} delete to this given path */
public void delete(String path) {
this.direct = new DirectCall();
direct.delete(path);
}
/** {@link DirectCall} head to this given path */
public void head(String path) {
this.direct = new DirectCall();
direct.head(path);
}
/** {@link DirectCall} patch to this given path */
public void patch(final String path) {
this.direct = new DirectCall();
direct.path(path);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy