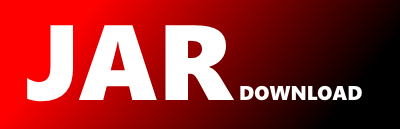
hope.kola.contract.api.HttpStatus Maven / Gradle / Ivy
package hope.kola.contract.api;
public enum HttpStatus {
// 400 CLIENT_ERROR Bad Request
BAD_REQUEST(400, "Bad Request", "错误请求(客户端)"),
// 401 CLIENT_ERROR Unauthorized
UNAUTHORIZED(401, "Unauthorized", "未授权(客户端)"),
// 402 CLIENT_ERROR Payment Required
PAYMENT_REQUIRED(402, "Payment Required", "未支付(客户端)"),
// 403 CLIENT_ERROR Forbidden
FORBIDDEN(403, "Forbidden", "禁止访问(客户端)"),
// 404 CLIENT_ERROR Not Found
NOT_FOUND(404, "Not Found", "资源未发现(客户端)"),
// 405 CLIENT_ERROR Method Not Allowed
METHOD_NOT_ALLOWED(405, "Method Not Allowed", "方法禁止访问(客户端)"),
// 406 CLIENT_ERROR Not Acceptable
NOT_ACCEPTABLE(406, "Not Acceptable", "请求无法接受(客户端)"),
// 407 CLIENT_ERROR Proxy Authentication Required
PROXY_AUTHENTICATION_REQUIRED(407, "Proxy Authentication Required", "代理授权错误(客户端)"),
// 408 CLIENT_ERROR Request Timeout
REQUEST_TIMEOUT(408, "Request Timeout", "请求超时(客户端)"),
// 409 CLIENT_ERROR Conflict
CONFLICT(409, "Conflict", "冲突(客户端)"),
// 410 CLIENT_ERROR Gone
GONE(410, "Gone", "丢失(客户端)"),
// 411 CLIENT_ERROR Length Required
LENGTH_REQUIRED(411, "Length Required", "长度未设置(客户端)"),
// 412 CLIENT_ERROR Precondition Failed
PRECONDITION_FAILED(412, "Precondition Failed", "先决条件检查失败(客户端)"),
// 413 CLIENT_ERROR Payload Too Large
PAYLOAD_TOO_LARGE(413, "Payload Too Large", "请求太大(客户端)"),
// 414 CLIENT_ERROR URI Too Long
URI_TOO_LONG(414, "URI Too Long", "URI长度超过(客户端)"),
// 415 CLIENT_ERROR Unsupported Media Type
UNSUPPORTED_MEDIA_TYPE(415, "Unsupported Media Type", "不支持数据格式(客户端)"),
// 416 CLIENT_ERROR Requested range not satisfiable
REQUESTED_RANGE_NOT_SATISFIABLE(416, "Requested range not satisfiable", "请求范围不满足(客户端)"),
// 417 CLIENT_ERROR Expectation Failed
EXPECTATION_FAILED(417, "Expectation Failed", "期待失败(客户端)"),
// 418 CLIENT_ERROR I'm a teapot
I_AM_A_TEAPOT(418, "I'm a teapot", "Teapot 测试(客户端)"),
// 419 CLIENT_ERROR Insufficient Space On Resource
INSUFFICIENT_SPACE_ON_RESOURCE(419, "Insufficient Space On Resource", "资源空间不足(客户端)"),
// 420 CLIENT_ERROR Method Failure
METHOD_FAILURE(420, "Method Failure", "方法错误(客户端)"),
// 421 CLIENT_ERROR Destination Locked
DESTINATION_LOCKED(421, "Destination Locked", "目标锁定(客户端)"),
// 422 CLIENT_ERROR Unprocessable Entity
UNPROCESSABLE_ENTITY(422, "Unprocessable Entity", "实体不可处理(客户端)"),
// 423 CLIENT_ERROR Locked
LOCKED(423, "Locked", "锁定(客户端)"),
// 424 CLIENT_ERROR Failed Dependency
FAILED_DEPENDENCY(424, "Failed Dependency", "依赖失败(客户端)"),
// 425 CLIENT_ERROR Too Early
TOO_EARLY(425, "Too Early", "过早(客户端)"),
// 426 CLIENT_ERROR Upgrade Required
UPGRADE_REQUIRED(426, "Upgrade Required", "升级失败(客户端)"),
// 428 CLIENT_ERROR Precondition Required
PRECONDITION_REQUIRED(428, "Precondition Required", "先决条件检验失败(客户端)"),
// 429 CLIENT_ERROR Too Many Requests
TOO_MANY_REQUESTS(429, "Too Many Requests", "太多请求(客户端)"),
// 431 CLIENT_ERROR Request Header Fields Too Large
REQUEST_HEADER_FIELDS_TOO_LARGE(431, "Request Header Fields Too Large", "头过大(客户端)"),
// 451 CLIENT_ERROR Unavailable For Legal Reasons
UNAVAILABLE_FOR_LEGAL_REASONS(451, "Unavailable For Legal Reasons", "非法(客户端)"),
// 500 SERVER_ERROR Internal Server Error
INTERNAL_SERVER_ERROR(500, "Internal Server Error", "服务器内部错误(服务端)"),
// 501 SERVER_ERROR Not Implemented
NOT_IMPLEMENTED(501, "Not Implemented", "未实现(服务端)"),
// 502 SERVER_ERROR Bad Gateway
BAD_GATEWAY(502, "Bad Gateway", "网关错误(服务端)"),
// 503 SERVER_ERROR Service Unavailable
SERVICE_UNAVAILABLE(503, "Service Unavailable", "服务不可达(服务端)"),
// 504 SERVER_ERROR Gateway Timeout
GATEWAY_TIMEOUT(504, "Gateway Timeout", "网关超时(服务端)"),
// 505 SERVER_ERROR HTTP Version not supported
HTTP_VERSION_NOT_SUPPORTED(505, "HTTP Version not supported", "HTTP版本不支持(服务端)"),
// 506 SERVER_ERROR Variant Also Negotiates
VARIANT_ALSO_NEGOTIATES(506, "Variant Also Negotiates", "变量协商异常(服务端)"),
// 507 SERVER_ERROR Insufficient Storage
INSUFFICIENT_STORAGE(507, "Insufficient Storage", "磁盘不足(服务端)"),
// 508 SERVER_ERROR Loop Detected
LOOP_DETECTED(508, "Loop Detected", "循环调用(服务端)"),
// 509 SERVER_ERROR Bandwidth Limit Exceeded
BANDWIDTH_LIMIT_EXCEEDED(509, "Bandwidth Limit Exceeded", "带宽限制(服务端)"),
// 510 SERVER_ERROR Not Extended
NOT_EXTENDED(510, "Not Extended", "不可扩展(服务端)"),
// 511 SERVER_ERROR Network Authentication Required
NETWORK_AUTHENTICATION_REQUIRED(511, "Network Authentication Required", "网络授权失败(服务端)");
public final int code;
public final String description;
public final String description2;
HttpStatus(int code, String description, String description2) {
this.code = code;
this.description = description;
this.description2 = description2;
}
public int code() {
return code;
}
public String description() {
return description;
}
public String description2() {
return description2;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy