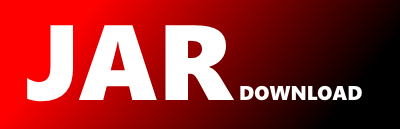
hope.kola.contract.api.ObjectFactory Maven / Gradle / Ivy
package hope.kola.contract.api;
import com.mifmif.common.regex.Generex;
import hope.kola.contract.faker.FakerObjectFactory;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.util.Arrays;
import java.util.List;
import java.util.UUID;
public interface ObjectFactory {
/** This is the mock factory helper to do all the mock things */
FakerObjectFactory Mocker = FakerObjectFactory.FAKER_OBJECT_FACTORY;
default List asList(T... values) {
return Arrays.asList(values);
}
default LocalDateTime nowDateTime() {
return LocalDateTime.now();
}
default LocalDateTime ofDateTime(int year, int month, int dayOfMonth, int hour, int minute) {
return LocalDateTime.of(year, month, dayOfMonth, hour, minute);
}
default DateTimeFormatter basicIsoDateFormatter() {
return DateTimeFormatter.BASIC_ISO_DATE;
}
default DateTimeFormatter isoDateFormatter() {
return DateTimeFormatter.ISO_DATE;
}
default LocalDateTime ofDateTime(String value) {
return LocalDateTime.parse(value);
}
default LocalDateTime ofDateTime(String value, String pattern) {
return LocalDateTime.parse(value, DateTimeFormatter.ofPattern(pattern));
}
default LocalDateTime ofDateTime(
int year, int month, int dayOfMonth, int hour, int minute, int second, int nanosecond) {
return LocalDateTime.of(year, month, dayOfMonth, hour, minute, second, nanosecond);
}
default LocalDate ofDate(int year, int month, int dayOfMonth) {
return LocalDate.of(year, month, dayOfMonth);
}
default LocalDate ofDate(String value) {
return LocalDate.parse(value);
}
default LocalDate ofDate(String value, String pattern) {
return LocalDate.parse(value, DateTimeFormatter.ofPattern(pattern));
}
default LocalTime ofTime(int hour, int minute) {
return LocalTime.of(hour, minute);
}
default LocalTime ofTime(String value) {
return LocalTime.parse(value);
}
default LocalTime ofTime(String value, String pattern) {
return LocalTime.parse(value, DateTimeFormatter.ofPattern(pattern));
}
default LocalTime nowTime() {
return LocalTime.now();
}
/**
* Gets the year field.
*
* This method returns the primitive {@code int} value for the year.
*
*
The year returned by this method is proleptic as per {@code get(YEAR)}. To obtain the
* year-of-era, use {@code get(YEAR_OF_ERA)}.
*
* @return the year, from MIN_YEAR to MAX_YEAR
*/
default Integer nowYear() {
return LocalDate.now().getYear();
}
/**
* Gets the month-of-year field from 1 to 12.
*
*
This method returns the month as an {@code int} from 1 to 12. Application code is frequently
* clearer if of enum {@link Month}
*
* @return the month-of-year, from 1 to 12
*/
default Integer nowMonth() {
return LocalDate.now().getMonthValue();
}
default Integer nowDayOfMonth() {
return LocalDate.now().getDayOfMonth();
}
/**
* Gets the day-of-year field.
*
*
This method returns the primitive {@code int} value for the day-of-year.
*
* @return the day-of-year, from 1 to 365, or 366 in a leap year
*/
default Integer nowDayOfYear() {
return LocalDate.now().getDayOfYear();
}
/**
* Gets the day-of-week field, which is an enum {@code DayOfWeek}.
*
*
This method returns the enum {@link DayOfWeek} for the day-of-week. This avoids confusion as
* to what {@code int} values mean. If you need access to the primitive {@code int} value then the
* enum provides the {@link DayOfWeek#getValue() int value}.
*
*
Additional information can be obtained from the {@code DayOfWeek}. This includes textual
* names of the values.
*
* @return the day-of-week, not null
*/
default DayOfWeek nowDayOfWeek() {
return LocalDate.now().getDayOfWeek();
}
/**
* Gets the hour-of-day field.
*
* @return the hour-of-day, from 0 to 23
*/
default Integer nowHour() {
return LocalTime.now().getHour();
}
/**
* Gets the minute-of-hour field.
*
* @return the minute-of-hour, from 0 to 59
*/
default Integer nowMinute() {
return LocalTime.now().getMinute();
}
/**
* Gets the second-of-minute field.
*
* @return the second-of-minute, from 0 to 59
*/
default Integer nowSecond() {
return LocalTime.now().getSecond();
}
/**
* Generate random string according to the given regular expression
*
* @param reg the regular expression
* @return string match the expression
*/
default String regular(final String reg) {
return new Generex(reg).random();
}
default String regular(final String reg, final int minLength) {
return new Generex(reg).random(minLength);
}
default String regular(final String reg, final int minLength, final int maxLength) {
return new Generex(reg).random(minLength, maxLength);
}
default BigDecimal ofBigDecimal(String value) {
return new BigDecimal(value);
}
default BigDecimal ofBigDecimal(Long value) {
return new BigDecimal(value);
}
default BigDecimal ofBigDecimal(Double value) {
return new BigDecimal(value);
}
default BigDecimal ofBigDecimal(Integer value) {
return new BigDecimal(value);
}
default BigDecimal ofBigDecimal(Float value) {
return new BigDecimal(value);
}
default BigInteger ofBigInteger(String value) {
return new BigInteger(value);
}
default BigInteger ofBigInteger(Long value) {
return BigInteger.valueOf(value);
}
/**
* delegate to {@link System#currentTimeMillis()}
*
* @return the difference, measured in milliseconds, between the current time and midnight,
* January 1, 1970 UTC.
*/
default Long currentTimeMillis() {
return System.currentTimeMillis();
}
/**
* delegate to {@link System#nanoTime()}
*
* @return the current value of the running Java Virtual Machine's high-resolution time source, in
* nanoseconds
*/
default Long nanoTime() {
return System.nanoTime();
}
default UUID randomUUID() {
return UUID.randomUUID();
}
}