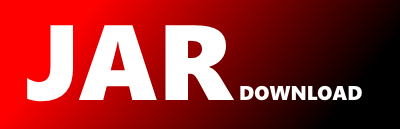
hope.kola.contract.api.Pageable Maven / Gradle / Ivy
package hope.kola.contract.api;
import hope.kola.contract.validation.Validatable;
import java.util.ArrayList;
import java.util.List;
import org.springframework.util.Assert;
public class Pageable implements Validatable {
/** Zero-based page index {@code (0..N)} */
protected Integer page = 0;
/**
* The size of the page to be returned
*
* {@code [1, 1024]}
*/
protected Integer size;
/** infinite scroll load this is the last offset if any key specific */
protected String offSet;
/**
* Sorting criteria in the format: property,(asc|desc),Default sort order is ascending,Multiple
* sort criteria are supported
*/
protected List sorts = new ArrayList<>();
public Integer page() {
return page;
}
public boolean hasSort() {
return !sorts.isEmpty();
}
/**
* Zero-based page index {@code (0..N)}
*
* @param page {@code (0..N)}
*/
public void page(Integer page) {
Assert.state(page >= 0, "page index must >= 0");
this.page = page;
}
public Integer size() {
return size;
}
/**
* The size of the page to be returned
*
* @param size {@code [1, 1024]}
*/
public void size(Integer size) {
Assert.state(size >= 1, "page size must >= 1");
this.size = size;
}
public List sorts() {
return sorts;
}
/**
* add sort field
*
* @param field which field to sort
* @param desc whether sort by desc
*/
public Pageable sort(final String field, final boolean desc) {
Assert.hasText(field, "sort field can not be empty");
sorts.add(new SortField(field, desc));
return this;
}
public record SortField(String field, boolean desc) {}
}