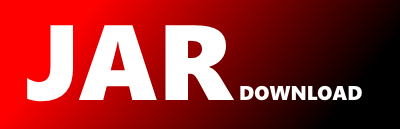
hope.kola.contract.api.ScriptFragment Maven / Gradle / Ivy
package hope.kola.contract.api;
import com.squareup.javapoet.CodeBlock;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.assertj.model.ObjectFragmentTrait;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.util.function.Consumer;
/** This Script Clip */
public class ScriptFragment implements ObjectFragmentTrait, KolaTestFunctions {
private final CodeBlock.Builder block = CodeBlock.builder();
public CodeBlock.Builder block() {
return block;
}
/**
* (JAVA) Add code block directly
*
* @param consumer pass by the underlying {@link CodeBlock.Builder}
*/
public void add(final Consumer consumer) {
consumer.accept(block);
}
/**
* Add code block directly
*
* @param consumer manipulate the code block directly
*/
public void add(@DelegatesTo(CodeBlock.Builder.class) Closure consumer) {
consumer.setDelegate(block);
consumer.call();
}
// LOCAL Operation === BEGIN
/**
* Set back value to local(this) property
*
* @param property the name of this property
* @param value the value of this property
*/
public void localSet(final String property, Integer value) {
block.addStatement("$L = $L", property, value);
}
public void localSet(final String property, String value) {
block.addStatement("$L = $S", property, value);
}
public void localSet(final String property, Long value) {
block.addStatement("$L = $Ll", property, value);
}
public void localSet(final String property, Double value) {
block.addStatement("$L = $Ld", property, value);
}
public void localSet(final String property, Float value) {
block.addStatement("$L = $Lf", property, value);
}
public void localSet(final String property, BigDecimal value) {
block.addStatement("$L = $L", property, ofBigDecimal(value).build());
}
public void localSet(final String property, BigInteger value) {
block.addStatement("$L = $L", property, ofBigInteger(value).build());
}
public void localSet(final String property, LocalDate value) {
block.addStatement("$L = $L", property, ofLocalDate(value).build());
}
public void localSetDate(final String property, String value) {
block.addStatement("$L = $L", property, ofLocalDate(value).build());
}
public void localSetDate(final String property, String value, String pattern) {
block.addStatement("$L = $L", property, ofLocalDate(value, pattern).build());
}
public void localSet(final String property, LocalDateTime value) {
block.addStatement("$L = $L", property, ofLocalDateTime(value).build());
}
public void localSetDateTime(final String property, String value) {
block.addStatement("$L = $L", property, ofLocalDateTime(value).build());
}
public void localSetDateTime(final String property, String value, String pattern) {
block.addStatement("$L = $L", property, ofLocalDateTime(value, pattern).build());
}
public void localSet(final String property, LocalTime value) {
block.addStatement("$L = $L", property, ofLocalTime(value).build());
}
public void localSetTime(final String property, String value) {
block.addStatement("$L = $L", property, ofLocalTime(value).build());
}
public void localSetTime(final String property, String value, String pattern) {
block.addStatement("$L = $L", property, ofLocalTime(value, pattern).build());
}
// LOCAL Operation === END
// Global Operation === BEGIN
public void removeGlobalValue(final String property) {
block.addStatement("$L($S)", _$V, property);
}
public void globalSet(final String property, Integer value) {
block.addStatement("$L($S,$L)", $V, property, value);
}
public void globalSet(final String property, String value) {
block.addStatement("$L($S,$S)", $V, property, value);
}
public void globalSet(final String property, Long value) {
block.addStatement("$L($S,$Ll)", $V, property, value);
}
public void globalSet(final String property, Double value) {
block.addStatement("$L($S,$Ld)", $V, property, value);
}
public void globalSet(final String property, Float value) {
block.addStatement("$L($S,$Lf)", $V, property, value);
}
public void globalSet(final String property, BigDecimal value) {
block.addStatement("$L($S,$L)", $V, property, ofBigDecimal(value).build());
}
public void globalSet(final String property, BigInteger value) {
block.addStatement("$L($S,$L)", $V, property, ofBigInteger(value).build());
}
public void globalSet(final String property, LocalDate value) {
block.addStatement("$L($S,$L)", $V, property, ofLocalDate(value).build());
}
public void globalSetDate(final String property, String value) {
block.addStatement("$L($S,$L)", $V, property, ofLocalDate(value).build());
}
public void globalSetDate(final String property, String value, String pattern) {
block.addStatement("$L($S,$L)", $V, property, ofLocalDate(value, pattern).build());
}
public void globalSet(final String property, LocalDateTime value) {
block.addStatement("$L($S,$L)", $V, property, ofLocalDateTime(value).build());
}
public void globalSetDateTime(final String property, String value) {
block.addStatement("$L($S,$L)", $V, property, ofLocalDateTime(value).build());
}
public void globalSetDateTime(final String property, String value, String pattern) {
block.addStatement("$L($S,$L)", $V, property, ofLocalDateTime(value, pattern).build());
}
public void globalSet(final String property, LocalTime value) {
block.addStatement("$L($S,$L)", $V, property, ofLocalTime(value).build());
}
public void globalSetTime(final String property, String value) {
block.addStatement("$L($S,$L)", $V, property, ofLocalTime(value).build());
}
public void globalSetTime(final String property, String value, String pattern) {
block.addStatement("$L($S,$L)", $V, property, ofLocalTime(value, pattern).build());
}
/**
* Set the header from the response body with path which will read from {@code
* com.jayway.jsonpath.DocumentContext} at runtime
*
* @param property the global head property
* @param path the path of the response body
*/
public void globalSetFromResponse(final String property, final String path) {
block.addStatement("$L($S, parsedResponse.read($S))", $V, property, path);
}
/**
* Set the global value from the response Head
*
* @param property name of the property
* @param headerName name of the header from the response
*/
public void globalSetFromResponseHead(final String property, final String headerName) {
block.addStatement("$L($S, response.header($S))", $V, property, headerName);
}
/**
* Set the global value from the response Cookie
*
* @param property name of the property
* @param cookieName name of the Cookie from the response
*/
public void globalSetFromResponseCookie(final String property, final String cookieName) {
block.addStatement("$L($S, response.cookie($S))", $V, property, cookieName);
}
// Global Operation === END
// Global Header Operation === BEGIN
public void removeHeadValue(final String property) {
block.addStatement("$L($S)", _$H, property);
}
public void headSet(final String property, Integer value) {
block.addStatement("$L($S,$L)", $H, property, value);
}
public void headSet(final String property, String value) {
block.addStatement("$L($S,$S)", $H, property, value);
}
public void headSet(final String property, Long value) {
block.addStatement("$L($S,$Ll)", $H, property, value);
}
public void headSet(final String property, Double value) {
block.addStatement("$L($S,$Ld)", $H, property, value);
}
public void headSet(final String property, Float value) {
block.addStatement("$L($S,$Lf)", $H, property, value);
}
public void HeadSet(final String property, BigDecimal value) {
block.addStatement("$L($S,$L)", $H, property, ofBigDecimal(value).build());
}
public void headSet(final String property, BigInteger value) {
block.addStatement("$L($S,$L)", $H, property, ofBigInteger(value).build());
}
public void headSet(final String property, LocalDate value) {
block.addStatement("$L($S,$L)", $H, property, ofLocalDate(value).build());
}
public void headSetDate(final String property, String value) {
block.addStatement("$L($S,$L)", $H, property, ofLocalDate(value).build());
}
public void headSetDate(final String property, String value, String pattern) {
block.addStatement("$L($S,$L)", $H, property, ofLocalDate(value, pattern).build());
}
public void headSet(final String property, LocalDateTime value) {
block.addStatement("$L($S,$L)", $H, property, ofLocalDateTime(value).build());
}
public void headSetDateTime(final String property, String value) {
block.addStatement("$L($S,$L)", $H, property, ofLocalDateTime(value).build());
}
public void headSetDateTime(final String property, String value, String pattern) {
block.addStatement("$L($S,$L)", $H, property, ofLocalDateTime(value, pattern).build());
}
public void headSet(final String property, LocalTime value) {
block.addStatement("$L($S,$L)", $H, property, ofLocalTime(value).build());
}
public void headSetTime(final String property, String value) {
block.addStatement("$L($S,$L)", $H, property, ofLocalTime(value).build());
}
public void headSetTime(final String property, String value, String pattern) {
block.addStatement("$L($S,$L)", $H, property, ofLocalTime(value, pattern).build());
}
/**
* Set the header from the response body with path which will read from {@code
* com.jayway.jsonpath.DocumentContext} at runtime
*
* @param property the global head property
* @param path the path of the response body
*/
public void headSetFromResponse(final String property, final String path) {
block.addStatement("$L($S, parsedResponse.read($S))", $H, property, path);
}
/**
* Set the header from the response Head
*
* @param property name of the property
* @param headerName name of the header from the response
*/
public void headSetFromResponseHead(final String property, final String headerName) {
block.addStatement("$L($S, response.header($S))", $H, property, headerName);
}
/**
* Set the header from the response Cookie
*
* @param property name of the property
* @param cookieName name of the Cookie from the response
*/
public void headSetFromResponseCookie(final String property, final String cookieName) {
block.addStatement("$L($S, response.cookie($S))", $H, property, cookieName);
}
// Global Header Operation === End
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy