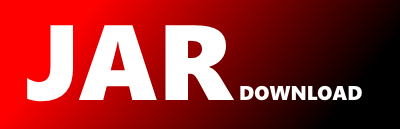
hope.kola.contract.api.ThenResponse Maven / Gradle / Ivy
package hope.kola.contract.api;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.validation.Validatable;
public class ThenResponse implements Validatable {
protected boolean mock = true;
/** if body present, then the body information is high priority, this should be json defined */
protected String json;
/**
* for ApiHug api this post processor script for easy mock response for Stub test
*
* Mock it?
*/
protected String postProcessorScript;
protected Body body;
public String getJson() {
return json;
}
public boolean shouldMock() {
return mock;
}
/**
* Set response mock flag to false, so the response will totally depend on the {@link
* #body(String)} to supply
*/
public void withoutMock() {
mock = true;
}
/**
* Body presentation
*
* @param body String template
*/
public void body(String body) {
this.json = body;
}
public String getPostProcessorScript() {
return postProcessorScript;
}
/**
* {@code Client} side stub logic to mock a more perfect {@code Response}
* {@code Groovy} script to manipulate the mocked response, Context Variables :
*
*
*
*
* Name
* Usage
* Type
* Comment
*
*
*
*
* $T
* 当前测试用例
* hope.kola.contract.verifier.context.T
类
* 操作上下文+帮助类
*
*
* -
* $H
* 方法
* 写回头信息
*
*
* -
* _$H
* 方法
* 删除头信息
*
*
* -
* $P
* 方法
* 写回参数
*
*
* -
* _$P
* 方法
* 删除参数
*
*
* -
* $V
* 方法
* 写回变量
*
*
* -
* _$V
* 方法
* 删除变量
*
*
* $RQ
* Request对象
* Map 类型动态Bean
* 发送前预处理
*
*
* $RQS
* Request Specification 对象
* io.restassured.specification.RequestSpecification
* 组装测试用例而用
*
*
* $RP
* Response对象
* 已经组装成Bean
* 返回结果验证
*
*
* $$RP
* Response对象
* 已经组装成Bean-解包的data部分
* 返回结果验证
*
*
* $RPJ
* Response Json对象
* com.jayway.jsonpath.DocumentContext
* 可进行结果 Path 验证
*
*
*
*
*
* Code sample:
*
*
*
*
* //Add a header to the global context
* $T.H('x-header', 'sample')
* $RP?.name.contract('-a-suffix')
*
*
*
*
* @param postProcessorScript
*/
public void postProcessorScript(String postProcessorScript) {
this.postProcessorScript = postProcessorScript;
}
/**
* Customized the Response body
*
* @param consumer manipulate the body;
*/
public void body(@DelegatesTo(Body.class) Closure consumer) {
this.body = new Body();
consumer.setDelegate(body);
consumer.call();
}
}