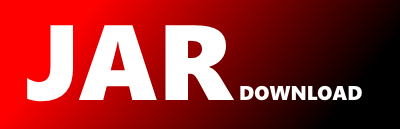
hope.kola.contract.api.ThenVerification Maven / Gradle / Ivy
package hope.kola.contract.api;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import hope.kola.contract.assertj.bridge.*;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
public class ThenVerification {
protected List pathVerifyList = new ArrayList<>();
/** Headers verification */
protected HeadVerifications headers;
/** Cookie verification */
protected CookieVerifications cookies;
private Integer status;
private Boolean expectSuccess;
/**
* TODO check Spring's logic Expect a success {@code [HttpStatus.SC_OK,
* HttpStatus.SC_MULTIPLE_CHOICES) -> [200,300)} status
*/
public void isOk() {
// [200, 300)
this.expectSuccess = true;
}
/** Expect the status is reverse {@link #isOk()} that is not {@code [200,300)} */
public void isFail() {
this.expectSuccess = false;
}
/**
* Expect the response status {@link HttpStatus}, for example {@link HttpStatus#BAD_REQUEST}
* meaning bad request
*
* @param status status of the response
*/
public void status(HttpStatus status) {
this.status = status.code();
}
/**
* Direct set the status of this response, for example {@code 200} meaning successfully
*
* @param status status of the response
*/
public void status(final int status) {
this.status = status;
}
public Integer status() {
return status;
}
public Boolean expectSuccess() {
return expectSuccess;
}
public List pathVerifyList() {
return pathVerifyList;
}
public boolean hasPathVerification() {
return pathVerifyList != null && !pathVerifyList.isEmpty();
}
/**
* Whether it has header verifications
*
* @return if exist
*/
public boolean hasHeaders() {
return headers != null && headers.hasEntries();
}
public HeadVerifications headers() {
return headers;
}
/**
* (JAVA) set up the header verification logic
*
* @param consumer function to manipulate the {@link HeadVerifications}
*/
public void headers(Consumer consumer) {
this.headers = new HeadVerifications();
consumer.accept(headers);
}
/**
* set up the header verification logic
*
* @param consumer function to manipulate the {@link HeadVerifications}
*/
public void headers(@DelegatesTo(HeadVerifications.class) Closure consumer) {
this.headers = new HeadVerifications();
consumer.setDelegate(headers);
consumer.call();
}
/**
* Whether it has Cookie verifications
*
* @return if exist
*/
public boolean hasCookies() {
return cookies != null && cookies.hasEntries();
}
public CookieVerifications cookies() {
return cookies;
}
/**
* (JAVA) set up the cookie verification logic
*
* @param consumer function to manipulate the {@link CookieVerifications}
*/
public void cookies(Consumer consumer) {
this.cookies = new CookieVerifications();
consumer.accept(cookies);
}
/**
* set up the cookie verification logic
*
* @param consumer function to manipulate the {@link CookieVerifications}
*/
public void cookies(@DelegatesTo(CookieVerifications.class) Closure consumer) {
this.cookies = new CookieVerifications();
consumer.setDelegate(cookies);
consumer.call();
}
/**
* {@link org.assertj.core.api.BigDecimalAssert} similar, verify {@link BigDecimal } kind property
*
*
*
*
*
* bigDecimalAssert("user.bigDecimalValue", {
* isEqualTo(new BigDecimal('12345678.99'))
* })
*
*
*
*
* @param path property path {@code user.bigDecimalValue} equal to {@code
* $.getUser().getBigDecimalValue()}
* @param closure function to manipulate the {@link BigDecimalAssert}
*/
public void bigDecimalAssert(
final String path, @DelegatesTo(BigDecimalAssert.class) Closure closure) {
final BigDecimalAssert bigDecimalAssert = new BigDecimalAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, bigDecimalAssert);
closure.setDelegate(bigDecimalAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.BigIntegerAssert} similar, verify {@link java.math.BigInteger }
* kind property
*
*
*
*
* bigIntegerAssert("user.bigIntegerValue", {
* isEqualTo(new BigInteger('12323233'))
* })
*
*
*
*
* @param path property path {@code user.bigIntegerValue} equal to {@code
* $.getUser().getBigIntegerValue()}
* @param closure function to manipulate the {@link BigIntegerAssert}
*/
public void bigIntegerAssert(
final String path, @DelegatesTo(BigIntegerAssert.class) Closure closure) {
final BigIntegerAssert bigIntegerAssert = new BigIntegerAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, bigIntegerAssert);
closure.setDelegate(bigIntegerAssert);
closure.call();
pathVerifyList.add(verify);
}
public void bigIntegerAssert(final String path, final Consumer consumer) {
final BigIntegerAssert bigIntegerAssert = new BigIntegerAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, bigIntegerAssert);
consumer.accept(bigIntegerAssert);
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.BooleanAssert} similar, verify {@link Boolean } kind property
*
*
*
*
* booleanAssert("user.booleanValue", {
* isTrue()
* })
*
*
*
*
* @param path property path {@code user.booleanValue} equal to {@code
* $.getUser().getBooleanValue()}
* @param closure function to manipulate the {@link BooleanAssert}
*/
public void booleanAssert(final String path, @DelegatesTo(BooleanAssert.class) Closure closure) {
final BooleanAssert booleanAssert = new BooleanAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, booleanAssert);
closure.setDelegate(booleanAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.ByteAssert} similar, verify {@link Byte } kind property
*
*
*
*
* byteAssert("user.byteValue", {
* isEqualTo('0')
* })
*
*
*
*
* @param path property path {@code user.byteValue} equal to {@code $.getUser().getByteValue()}
* @param closure function to manipulate the {@link ByteAssert}
*/
public void byteAssert(final String path, @DelegatesTo(ByteAssert.class) Closure closure) {
final ByteAssert byteAssert = new ByteAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, byteAssert);
closure.setDelegate(byteAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.DoubleAssert} similar, verify {@link Double } kind property
*
*
*
*
* doubleAssert("user.doubleValue", {
* isEqualTo(124d)
* })
*
*
*
*
* @param path property path {@code user.doubleValue} equal to {@code
* $.getUser().getDoubleValue()}
* @param closure function to manipulate the {@link DoubleAssert}
*/
public void doubleAssert(final String path, @DelegatesTo(DoubleAssert.class) Closure closure) {
final DoubleAssert doubleAssert = new DoubleAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, doubleAssert);
closure.setDelegate(doubleAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.FloatAssert} similar, verify {@link Float } kind property
*
*
*
*
* floatAssert("user.floatValue", {
* isEqualTo(123.1f)
* })
*
*
*
*
* @param path property path {@code user.floatValue} equal to {@code $.getUser().getFloatValue()}
* @param closure function to manipulate the {@link FloatAssert}
*/
public void floatAssert(final String path, @DelegatesTo(FloatAssert.class) Closure closure) {
final FloatAssert floatAssert = new FloatAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, floatAssert);
closure.setDelegate(floatAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.InstantAssert} similar, verify {@link java.time.Instant } kind
* property
*
*
*
*
* instantAssert("user.instantValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.instantValue} equal to {@code
* $.getUser().getInstantValue()}
* @param closure function to manipulate the {@link InstantAssert}
*/
public void instantAssert(final String path, @DelegatesTo(InstantAssert.class) Closure closure) {
final InstantAssert instantAssert = new InstantAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, instantAssert);
closure.setDelegate(instantAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.IntegerAssert} similar, verify {@link Integer } kind property
*
*
*
*
* integerAssert("user.integerValue", {
* isEqualTo(12)
* })
*
*
*
*
* @param path property path {@code user.integerValue} equal to {@code
* $.getUser().getIntegerValue()}
* @param closure function to manipulate the {@link IntegerAssert}
*/
public void integerAssert(final String path, @DelegatesTo(IntegerAssert.class) Closure closure) {
final IntegerAssert integerAssert = new IntegerAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, integerAssert);
closure.setDelegate(integerAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.LocalDateAssert} similar, verify {@link java.time.LocalDate } kind
* property
*
*
*
*
* localDateAssert("user.localDateValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.localDateValue} equal to {@code
* $.getUser().getLocalDateValue()}
* @param closure function to manipulate the {@link LocalDateAssert}
*/
public void localDateAssert(
final String path, @DelegatesTo(LocalDateAssert.class) Closure closure) {
final LocalDateAssert localDateAssert = new LocalDateAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, localDateAssert);
closure.setDelegate(localDateAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.LocalDateTimeAssert} similar, verify {@link java.time.LocalDateTime
* } kind property
*
*
*
*
* localDateTimeAssert("user.localDateTimeValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.localDateTimeValue} equal to {@code
* $.getUser().getLocalDateTimeValue()}
* @param closure function to manipulate the {@link LocalDateTimeAssert}
*/
public void localDateTimeAssert(
final String path, @DelegatesTo(LocalDateTimeAssert.class) Closure closure) {
final LocalDateTimeAssert localDateTimeAssert = new LocalDateTimeAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, localDateTimeAssert);
closure.setDelegate(localDateTimeAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.LocalTimeAssert} similar, verify {@link java.time.LocalDateTime }
* kind property
*
*
*
*
* localTimeAssert("user.localTimeValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.localTimeValue} equal to {@code
* $.getUser().getLocalTimeValue()}
* @param closure function to manipulate the {@link LocalTimeAssert}
*/
public void localTimeAssert(
final String path, @DelegatesTo(LocalTimeAssert.class) Closure closure) {
final LocalTimeAssert localTimeAssert = new LocalTimeAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, localTimeAssert);
closure.setDelegate(localTimeAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.LongAssert} similar, verify {@link Long } kind property
*
*
*
*
* longAssert("user.longValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.longValue} equal to {@code $.getUser().getLongValue()}
* @param closure function to manipulate the {@link LongAssert}
*/
public void longAssert(final String path, @DelegatesTo(LongAssert.class) Closure closure) {
final LongAssert longAssert = new LongAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, longAssert);
closure.setDelegate(longAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.PeriodAssert} similar, verify {@link java.time.Period } kind
* property
*
*
*
*
* periodAssert("user.periodValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.periodValue} equal to {@code
* $.getUser().getPeriodValue()}
* @param closure function to manipulate the {@link PeriodAssert}
*/
public void periodAssert(final String path, @DelegatesTo(PeriodAssert.class) Closure closure) {
final PeriodAssert periodAssert = new PeriodAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, periodAssert);
closure.setDelegate(periodAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.ShortAssert} similar, verify {@link Short } kind property
*
*
*
*
* shortAssert("user.shortValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.shortValue} equal to {@code $.getUser().getShortValue()}
* @param closure function to manipulate the {@link ShortAssert}
*/
public void shortAssert(final String path, @DelegatesTo(ShortAssert.class) Closure closure) {
final ShortAssert shortAssert = new ShortAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, shortAssert);
closure.setDelegate(shortAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* {@link org.assertj.core.api.StringAssert} similar, verify {@link String } kind property
*
*
*
*
* stringAssert("user.stringValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.stringValue} equal to {@code
* $.getUser().getStringValue()}
* @param closure function to manipulate the {@link StringAssert}
*/
public void stringAssert(final String path, @DelegatesTo(StringAssert.class) Closure closure) {
final StringAssert stringAssert = new StringAssert();
ResponseBodyVerification verify = new ResponseBodyVerification(path, stringAssert);
closure.setDelegate(stringAssert);
closure.call();
pathVerifyList.add(verify);
}
/**
* (JAVA) {@link org.assertj.core.api.StringAssert} similar, verify {@link String } kind property
*
*
*
*
*
* stringAssert("user.stringValue", {
* isEqualTo()
* })
*
*
*
*
* @param path property path {@code user.stringValue} equal to {@code
* $.getUser().getStringValue()}
* @param stringAssert instance of {@link StringAssert}
*/
public void stringAssert(final String path, final StringAssert stringAssert) {
ResponseBodyVerification verify = new ResponseBodyVerification(path, stringAssert);
pathVerifyList.add(verify);
}
// Easy helper
/** Bad Request {@code 400} */
public void isBadRequest() {
this.status = HttpStatus.BAD_REQUEST.code();
}
/** Unauthorized {@code 401} */
public void isUnauthorized() {
this.status = HttpStatus.UNAUTHORIZED.code();
}
/** Payment Required {@code 402} */
public void isPaymentRequired() {
this.status = HttpStatus.PAYMENT_REQUIRED.code();
}
/** Forbidden {@code 403} */
public void isForbidden() {
this.status = HttpStatus.FORBIDDEN.code();
}
/** Not Found {@code 404} */
public void isNotFound() {
this.status = HttpStatus.NOT_FOUND.code();
}
/** Method Not Allowed {@code 405} */
public void isMethodNotAllowed() {
this.status = HttpStatus.METHOD_NOT_ALLOWED.code();
}
/** Not Acceptable {@code 406} */
public void isNotAcceptable() {
this.status = HttpStatus.NOT_ACCEPTABLE.code();
}
/** Proxy Authentication Required {@code 407} */
public void isProxyAuthenticationRequired() {
this.status = HttpStatus.PROXY_AUTHENTICATION_REQUIRED.code();
}
/** Request Timeout {@code 408} */
public void isRequestTimeout() {
this.status = HttpStatus.REQUEST_TIMEOUT.code();
}
/** Conflict {@code 409} */
public void isConflict() {
this.status = HttpStatus.CONFLICT.code();
}
/** Gone {@code 410} */
public void isGone() {
this.status = HttpStatus.GONE.code();
}
/** Length Required {@code 411} */
public void isLengthRequired() {
this.status = HttpStatus.LENGTH_REQUIRED.code();
}
/** Precondition Failed {@code 412} */
public void isPreconditionFailed() {
this.status = HttpStatus.PRECONDITION_FAILED.code();
}
/** Payload Too Large {@code 413} */
public void isPayloadTooLarge() {
this.status = HttpStatus.PAYLOAD_TOO_LARGE.code();
}
/** URI Too Long {@code 414} */
public void isUriTooLong() {
this.status = HttpStatus.URI_TOO_LONG.code();
}
/** Unsupported Media Type {@code 415} */
public void isUnsupportedMediaType() {
this.status = HttpStatus.UNSUPPORTED_MEDIA_TYPE.code();
}
/** Requested range not satisfiable {@code 416} */
public void isRequestedRangeNotSatisfiable() {
this.status = HttpStatus.REQUESTED_RANGE_NOT_SATISFIABLE.code();
}
/** Expectation Failed {@code 417} */
public void isExpectationFailed() {
this.status = HttpStatus.EXPECTATION_FAILED.code();
}
/** I'm a teapot {@code 418} */
public void isIAmATeapot() {
this.status = HttpStatus.I_AM_A_TEAPOT.code();
}
/** Insufficient Space On Resource {@code 419} */
public void isInsufficientSpaceOnResource() {
this.status = HttpStatus.INSUFFICIENT_SPACE_ON_RESOURCE.code();
}
/** Method Failure {@code 420} */
public void isMethodFailure() {
this.status = HttpStatus.METHOD_FAILURE.code();
}
/** Destination Locked {@code 421} */
public void isDestinationLocked() {
this.status = HttpStatus.DESTINATION_LOCKED.code();
}
/** Unprocessable Entity {@code 422} */
public void isUnprocessableEntity() {
this.status = HttpStatus.UNPROCESSABLE_ENTITY.code();
}
/** Locked {@code 423} */
public void isLocked() {
this.status = HttpStatus.LOCKED.code();
}
/** Failed Dependency {@code 424} */
public void isFailedDependency() {
this.status = HttpStatus.FAILED_DEPENDENCY.code();
}
/** Too Early {@code 425} */
public void isTooEarly() {
this.status = HttpStatus.TOO_EARLY.code();
}
/** Upgrade Required {@code 426} */
public void isUpgradeRequired() {
this.status = HttpStatus.UPGRADE_REQUIRED.code();
}
/** Precondition Required {@code 428} */
public void isPreconditionRequired() {
this.status = HttpStatus.PRECONDITION_REQUIRED.code();
}
/** Too Many Requests {@code 429} */
public void isTooManyRequests() {
this.status = HttpStatus.TOO_MANY_REQUESTS.code();
}
/** Request Header Fields Too Large {@code 431} */
public void isRequestHeaderFieldsTooLarge() {
this.status = HttpStatus.REQUEST_HEADER_FIELDS_TOO_LARGE.code();
}
/** Unavailable For Legal Reasons {@code 451} */
public void isUnavailableForLegalReasons() {
this.status = HttpStatus.UNAVAILABLE_FOR_LEGAL_REASONS.code();
}
/** Internal Server Error {@code 500} */
public void isInternalServerError() {
this.status = HttpStatus.INTERNAL_SERVER_ERROR.code();
}
/** Not Implemented {@code 501} */
public void isNotImplemented() {
this.status = HttpStatus.NOT_IMPLEMENTED.code();
}
/** Bad Gateway {@code 502} */
public void isBadGateway() {
this.status = HttpStatus.BAD_GATEWAY.code();
}
/** Service Unavailable {@code 503} */
public void isServiceUnavailable() {
this.status = HttpStatus.SERVICE_UNAVAILABLE.code();
}
/** Gateway Timeout {@code 504} */
public void isGatewayTimeout() {
this.status = HttpStatus.GATEWAY_TIMEOUT.code();
}
/** HTTP Version not supported {@code 505} */
public void isHttpVersionNotSupported() {
this.status = HttpStatus.HTTP_VERSION_NOT_SUPPORTED.code();
}
/** Variant Also Negotiates {@code 506} */
public void isVariantAlsoNegotiates() {
this.status = HttpStatus.VARIANT_ALSO_NEGOTIATES.code();
}
/** Insufficient Storage {@code 507} */
public void isInsufficientStorage() {
this.status = HttpStatus.INSUFFICIENT_STORAGE.code();
}
/** Loop Detected {@code 508} */
public void isLoopDetected() {
this.status = HttpStatus.LOOP_DETECTED.code();
}
/** Bandwidth Limit Exceeded {@code 509} */
public void isBandwidthLimitExceeded() {
this.status = HttpStatus.BANDWIDTH_LIMIT_EXCEEDED.code();
}
/** Not Extended {@code 510} */
public void isNotExtended() {
this.status = HttpStatus.NOT_EXTENDED.code();
}
/** Network Authentication Required {@code 511} */
public void isNetworkAuthenticationRequired() {
this.status = HttpStatus.NETWORK_AUTHENTICATION_REQUIRED.code();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy