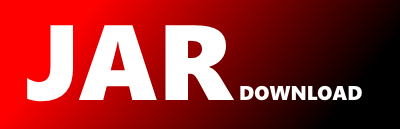
hope.kola.contract.api.WhenRequest Maven / Gradle / Ivy
package hope.kola.contract.api;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
public class WhenRequest {
public String json;
/** Body DSL */
public Body body;
private Pageable pageable;
private Headers headers;
private Cookies cookies;
private QueryParameters queryParameters;
private PathParameters pathParameters;
private Multipart multipart;
/**
* Allows to configure HTTP Path Parameters
*
* @param consumer function to manipulate the Path Parameters
*/
public void paths(@DelegatesTo(PathParameters.class) Closure consumer) {
this.pathParameters = new PathParameters();
consumer.setDelegate(this.pathParameters);
consumer.call();
}
/**
* Get all the Path Parameters
*
* @return {@link PathParameters}
*/
public PathParameters getPaths() {
return pathParameters;
}
/**
* (JAVA) Allows to configure HTTP Path Parameters.
*
* @param paths {@link PathParameters} setting
*/
public void setPaths(final PathParameters paths) {
this.pathParameters = paths;
}
/**
* Allows to configure HTTP Query Parameters
*
* @param consumer function to manipulate the Query Parameters
*/
public void queries(@DelegatesTo(QueryParameters.class) Closure consumer) {
this.queryParameters = new QueryParameters();
consumer.setDelegate(this.queryParameters);
consumer.call();
}
/**
* Get all the Query Parameters
*
* @return {@link QueryParameters}
*/
public QueryParameters getQueries() {
return queryParameters;
}
/**
* (JAVA) Allows to configure HTTP Query Parameters.
*
* @param queries {@link QueryParameters} setting
*/
public void setQueries(final QueryParameters queries) {
this.queryParameters = queries;
}
/**
* Allows to configure HTTP cookies
*
* @param consumer function to manipulate the cookies
*/
public void cookies(@DelegatesTo(Cookies.class) Closure consumer) {
this.cookies = new Cookies();
consumer.setDelegate(this.cookies);
consumer.call();
}
/**
* Get all the cookies
*
* @return {@link Cookies}
*/
public Cookies getCookies() {
return cookies;
}
/**
* (JAVA) Allows to configure HTTP cookies.
*
* @param cookies {@link Cookies} setting
*/
public void setCookies(final Cookies cookies) {
this.cookies = cookies;
}
/**
* Allows to configure HTTP headers.
*
* @param consumer function to manipulate the headers
*/
public void headers(@DelegatesTo(Headers.class) Closure consumer) {
this.headers = new Headers();
consumer.setDelegate(this.headers);
consumer.call();
}
/**
* Get the headers
*
* @return {@link Headers}
*/
public Headers getHeaders() {
return headers;
}
/**
* (JAVA) Allows to configure HTTP headers.
*
* @param headers {@link Headers} setting
*/
public void setHeaders(final Headers headers) {
this.headers = headers;
}
public String getJson() {
return json;
}
public void json(String json) {
this.json = json;
}
/**
* Customized the request body
*
* @param consumer manipulate the {@link Body};
*/
public void body(@DelegatesTo(Body.class) Closure consumer) {
this.body = new Body();
consumer.setDelegate(body);
consumer.call();
}
/**
* (JAVA) Customized the request body
*
* @param body {@link Body}
*/
public void setBody(final Body body) {
this.body = body;
}
public Pageable getPageable() {
return pageable;
}
/**
* (JAVA) Mark this request as pageable
*
* @param pageable {@link Pageable}
*/
public void setPageable(final Pageable pageable) {
this.pageable = pageable;
}
/**
* Mark this request as pageable
*
* @param consumer manipulate the {@link Pageable}
*/
public void pageable(@DelegatesTo(Pageable.class) Closure consumer) {
this.pageable = new Pageable();
consumer.setDelegate(pageable);
consumer.call();
}
/**
* Add multiple part logic
*
* @param consumer manipulate the {@link Multipart}
*/
public void multipart(@DelegatesTo(Multipart.class) Closure consumer) {
this.multipart = new Multipart();
consumer.setDelegate(multipart);
consumer.call();
}
public Multipart getMultipart() {
return multipart;
}
/**
* (JAVA) Add multiple part logic
*
* @param multipart {@lin Multipart} instance
*/
public void setMultipart(Multipart multipart) {
this.multipart = multipart;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy