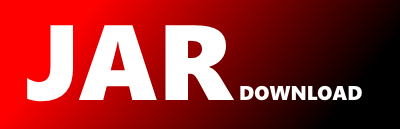
hope.kola.contract.assertj.bridge.LocalDateTimeAssert Maven / Gradle / Ivy
package hope.kola.contract.assertj.bridge;
import com.squareup.javapoet.ClassName;
import hope.kola.contract.assertj.model.DynamicProperty;
import hope.kola.contract.assertj.model.PrimitiveType;
import java.time.LocalDateTime;
import java.time.Month;
import org.assertj.core.data.TemporalUnitOffset;
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert} */
public class LocalDateTimeAssert extends AssertThat {
@Override
public PrimitiveType primitiveType() {
return PrimitiveType.DATE_TIME;
}
@Override
public ClassName assertJClass() {
return ClassName.get("org.assertj.core.api", "AbstractLocalDateTimeAssert");
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isBefore} */
public LocalDateTimeAssert isBefore(LocalDateTime other) {
segment("isBefore").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isBeforeOrEqualTo} */
public LocalDateTimeAssert isBeforeOrEqualTo(LocalDateTime other) {
segment("isBeforeOrEqualTo").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isAfterOrEqualTo} */
public LocalDateTimeAssert isAfterOrEqualTo(LocalDateTime other) {
segment("isAfterOrEqualTo").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isAfter} */
public LocalDateTimeAssert isAfter(LocalDateTime other) {
segment("isAfter").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isEqualTo} */
public LocalDateTimeAssert isEqualTo(Object other) {
// TODO add your logic
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isNotEqualTo} */
public LocalDateTimeAssert isNotEqualTo(Object other) {
// TODO add your logic
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isIn} */
public LocalDateTimeAssert isIn(String[] dateTimesAsString) {
segment("isIn").argument(DynamicProperty.newStringArray(dateTimesAsString));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isNotIn} */
public LocalDateTimeAssert isNotIn(String[] dateTimesAsString) {
segment("isNotIn").argument(DynamicProperty.newStringArray(dateTimesAsString));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isCloseToUtcNow} */
public LocalDateTimeAssert isCloseToUtcNow(TemporalUnitOffset offset) {
// TODO add your logic
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isEqualToIgnoringNanos} */
public LocalDateTimeAssert isEqualToIgnoringNanos(LocalDateTime other) {
segment("isEqualToIgnoringNanos").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isEqualToIgnoringSeconds} */
public LocalDateTimeAssert isEqualToIgnoringSeconds(LocalDateTime other) {
segment("isEqualToIgnoringSeconds").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isEqualToIgnoringMinutes} */
public LocalDateTimeAssert isEqualToIgnoringMinutes(LocalDateTime other) {
segment("isEqualToIgnoringMinutes").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isEqualToIgnoringHours} */
public LocalDateTimeAssert isEqualToIgnoringHours(LocalDateTime other) {
segment("isEqualToIgnoringHours").argument(DynamicProperty.newLocalDateTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isBetween} */
public LocalDateTimeAssert isBetween(LocalDateTime startInclusive, LocalDateTime endInclusive) {
segment("isBetween")
.argument(DynamicProperty.newLocalDateTime(startInclusive))
.argument(DynamicProperty.newLocalDateTime(endInclusive));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#isStrictlyBetween} */
public LocalDateTimeAssert isStrictlyBetween(
LocalDateTime startExclusive, LocalDateTime endExclusive) {
segment("isStrictlyBetween")
.argument(DynamicProperty.newLocalDateTime(startExclusive))
.argument(DynamicProperty.newLocalDateTime(endExclusive));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasYear} */
public LocalDateTimeAssert hasYear(Integer year) {
segment("hasYear").argument(DynamicProperty.newInteger(year));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasMonth} */
public LocalDateTimeAssert hasMonth(Month month) {
segment("hasMonth").argument(DynamicProperty.newMonth(month));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasMonthValue} */
public LocalDateTimeAssert hasMonthValue(Integer monthVal) {
segment("hasMonthValue").argument(DynamicProperty.newInteger(monthVal));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasDayOfMonth} */
public LocalDateTimeAssert hasDayOfMonth(Integer dayOfMonth) {
segment("hasDayOfMonth").argument(DynamicProperty.newInteger(dayOfMonth));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasHour} */
public LocalDateTimeAssert hasHour(Integer hour) {
segment("hasHour").argument(DynamicProperty.newInteger(hour));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasMinute} */
public LocalDateTimeAssert hasMinute(Integer minute) {
segment("hasMinute").argument(DynamicProperty.newInteger(minute));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasSecond} */
public LocalDateTimeAssert hasSecond(Integer second) {
segment("hasSecond").argument(DynamicProperty.newInteger(second));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalDateTimeAssert#hasNano} */
public LocalDateTimeAssert hasNano(Integer nano) {
segment("hasNano").argument(DynamicProperty.newInteger(nano));
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy