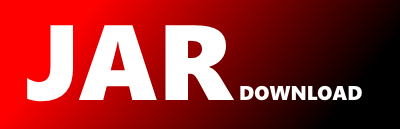
hope.kola.contract.assertj.bridge.LocalTimeAssert Maven / Gradle / Ivy
package hope.kola.contract.assertj.bridge;
import com.squareup.javapoet.ClassName;
import hope.kola.contract.assertj.model.DynamicProperty;
import hope.kola.contract.assertj.model.PrimitiveType;
import java.time.LocalTime;
/** {@link org.assertj.core.api.AbstractLocalTimeAssert} */
public class LocalTimeAssert extends AssertThat {
@Override
public PrimitiveType primitiveType() {
return PrimitiveType.TIME;
}
@Override
public ClassName assertJClass() {
return ClassName.get("org.assertj.core.api", "AbstractLocalTimeAssert");
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isBefore} */
public LocalTimeAssert isBefore(LocalTime other) {
segment("isBefore").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isBeforeOrEqualTo} */
public LocalTimeAssert isBeforeOrEqualTo(LocalTime other) {
segment("isBeforeOrEqualTo").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isAfterOrEqualTo} */
public LocalTimeAssert isAfterOrEqualTo(LocalTime other) {
segment("isAfterOrEqualTo").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isAfter} */
public LocalTimeAssert isAfter(LocalTime other) {
segment("isAfter").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isEqualTo} */
public LocalTimeAssert isEqualTo(String localTimeAsString) {
segment("isEqualTo").argument(DynamicProperty.newString(localTimeAsString));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isNotEqualTo} */
public LocalTimeAssert isNotEqualTo(String localTimeAsString) {
segment("isNotEqualTo").argument(DynamicProperty.newString(localTimeAsString));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isIn} */
public LocalTimeAssert isIn(String[] localTimesAsString) {
segment("isIn").argument(DynamicProperty.newStringArray(localTimesAsString));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isNotIn} */
public LocalTimeAssert isNotIn(String[] localTimesAsString) {
segment("isNotIn").argument(DynamicProperty.newStringArray(localTimesAsString));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isEqualToIgnoringNanos} */
public LocalTimeAssert isEqualToIgnoringNanos(LocalTime other) {
segment("isEqualToIgnoringNanos").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isEqualToIgnoringSeconds} */
public LocalTimeAssert isEqualToIgnoringSeconds(LocalTime other) {
segment("isEqualToIgnoringSeconds").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#hasSameHourAs} */
public LocalTimeAssert hasSameHourAs(LocalTime other) {
segment("hasSameHourAs").argument(DynamicProperty.newLocalTime(other));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isBetween} */
public LocalTimeAssert isBetween(LocalTime startInclusive, LocalTime endInclusive) {
segment("isBetween")
.argument(DynamicProperty.newLocalTime(startInclusive))
.argument(DynamicProperty.newLocalTime(endInclusive));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#isStrictlyBetween} */
public LocalTimeAssert isStrictlyBetween(LocalTime startExclusive, LocalTime endExclusive) {
segment("isStrictlyBetween")
.argument(DynamicProperty.newLocalTime(startExclusive))
.argument(DynamicProperty.newLocalTime(endExclusive));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#hasHour} */
public LocalTimeAssert hasHour(Integer hour) {
segment("hasHour").argument(DynamicProperty.newInteger(hour));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#hasMinute} */
public LocalTimeAssert hasMinute(Integer minute) {
segment("hasMinute").argument(DynamicProperty.newInteger(minute));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#hasSecond} */
public LocalTimeAssert hasSecond(Integer second) {
segment("hasSecond").argument(DynamicProperty.newInteger(second));
return this;
}
/** {@link org.assertj.core.api.AbstractLocalTimeAssert#hasNano} */
public LocalTimeAssert hasNano(Integer nano) {
segment("hasNano").argument(DynamicProperty.newInteger(nano));
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy