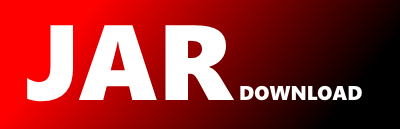
hope.kola.contract.assertj.model.DynamicProperty Maven / Gradle / Ivy
package hope.kola.contract.assertj.model;
import com.squareup.javapoet.ClassName;
import com.squareup.javapoet.CodeBlock;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.*;
import java.util.Arrays;
import java.util.List;
import java.util.UUID;
import java.util.function.Consumer;
import org.apache.commons.lang3.StringUtils;
import org.assertj.core.data.Offset;
public class DynamicProperty implements ObjectFragmentTrait {
/** Property type default is {@link PrimitiveType#STRING} */
protected PrimitiveType type = null;
/** Static value, not changeable, must be primitive or enum */
protected T value;
/** Whether this property is List? */
protected boolean plural;
/** if not Pre-defined type then customized */
protected String clz;
protected CodeBlock.Builder code;
public static DynamicProperty newLong(final Long value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.LONG;
res.code = CodeBlock.builder().add("$Ll", value);
return res;
}
public static DynamicProperty newInteger(final Integer value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.INTEGER;
res.code = CodeBlock.builder().add("$L", value);
return res;
}
public static DynamicProperty newDouble(final Double value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.DOUBLE;
res.code = CodeBlock.builder().add("$Ld", value);
return res;
}
public static DynamicProperty newFloat(final Float value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.FLOAT;
res.code = CodeBlock.builder().add("$Lf", value);
return res;
}
public static DynamicProperty newString(final String value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.STRING;
res.code = CodeBlock.builder().add("$S", value);
return res;
}
public static DynamicProperty newBigDecimal(final BigDecimal value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.BIG_DECIMAL;
res.code = res.ofBigDecimal(value);
return res;
}
public static DynamicProperty newBoolean(final Boolean value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.BOOLEAN;
res.code = CodeBlock.builder().add("$L", value);
return res;
}
public static DynamicProperty newBigInteger(final BigInteger value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.BIG_INTEGER;
res.code = res.ofBigInteger(value);
return res;
}
/**
* @see LocalDate#of(int, int, int)
* @param value
* @return
*/
public static DynamicProperty newLocalDate(final LocalDate value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.DATE;
// LocalDate of(int year, int month, int dayOfMonth)
res.code = res.ofLocalDate(value);
return res;
}
public static DynamicProperty newLocalDateTime(final LocalDateTime value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.DATE_TIME;
res.code = res.ofLocalDateTime(value);
return res;
}
public static DynamicProperty newLocalTime(final LocalTime value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.TIME;
// LocalTime.of(int hour, int minute, int second, int nanoOfSecond)
res.code = res.ofLocalTime(value);
return res;
}
public static DynamicProperty newUUID(final UUID value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.UUID;
res.code = res.ofUUID(value);
return res;
}
public static DynamicProperty newUUID(final String value) {
final DynamicProperty res = new DynamicProperty();
res.value = UUID.fromString(value);
res.type = PrimitiveType.UUID;
res.code = res.ofUUID(value);
return res;
}
public static DynamicProperty newByte(final Byte value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.BYTE;
res.code = CodeBlock.builder().add("'$L'", value);
return res;
}
public static DynamicProperty newShort(final Short value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.SHORT;
res.code = CodeBlock.builder().add("$L", value);
return res;
}
public static DynamicProperty newEnum(final T value) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.EUM;
res.code = CodeBlock.builder().add("$T.$L", ClassName.get(value.getClass()), value.name());
return res;
}
public static DynamicProperty newGeneric(
final T value, final Consumer builder) {
final DynamicProperty res = new DynamicProperty();
res.value = value;
res.type = PrimitiveType.CODE;
res.code = CodeBlock.builder();
builder.accept(res.code);
return res;
}
public static DynamicProperty newMonth(final Month month) {
return DynamicProperty.newGeneric(
month, builder -> builder.add("$T.$L", Month.class, month.name()));
}
public static DynamicProperty newOffset(final Offset offset) {
return DynamicProperty.newGeneric(offset, offsetBuilder(offset));
}
public static DynamicProperty newInstant(final Instant instant) {
return DynamicProperty.newGeneric(instant, instantBuilder(instant));
}
public static DynamicProperty newStringArray(final String[] values) {
return DynamicProperty.newGeneric(
values,
builder -> {
// $S, $S, $S
String _t = StringUtils.repeat("$S", ", ", values.length);
builder.add(_t, values);
});
}
public static DynamicProperty newIntegerArray(final Integer[] values) {
return DynamicProperty.newGeneric(
values,
builder -> {
// $S, $S, $S
String _t = StringUtils.repeat("$L", ", ", values.length);
builder.add(_t, values);
});
}
public static DynamicProperty newLongArray(final Long[] values) {
return DynamicProperty.newGeneric(
values,
builder -> {
// $S, $S, $S
String _t = StringUtils.repeat("$Ll", ", ", values.length);
builder.add(_t, values);
});
}
public static DynamicProperty newFloatArray(final Float[] values) {
return DynamicProperty.newGeneric(
values,
builder -> {
// $S, $S, $S
String _t = StringUtils.repeat("$Lf", ", ", values.length);
builder.add(_t, values);
});
}
public static DynamicProperty newDoubleArray(final Double[] values) {
return DynamicProperty.newGeneric(
values,
builder -> {
// $S, $S, $S
String _t = StringUtils.repeat("$Ld", ", ", values.length);
builder.add(_t, values);
});
}
public static DynamicProperty newDoubleArray(final Byte[] values) {
return DynamicProperty.newGeneric(
values,
builder -> {
// $L, $L, $L
String _t = StringUtils.repeat("'$L'", ", ", values.length);
builder.add(_t, values);
});
}
private static Consumer instantBuilder(final Instant instant) {
return builder -> builder.add("$T.ofEpochMilli($L)", Instant.class, instant.toEpochMilli());
}
private static Consumer offsetBuilder(final Offset offset) {
return builder -> {
if (offset.strict) {
builder.add("$T.strictOffset($L)", Offset.class, offset.value);
} else {
builder.add("$T.offset($L)", Offset.class, offset.value);
}
};
}
private static Object[] asArg(final Object first, final Object... values) {
Object[] res = new Object[values.length + 1];
res[0] = first;
int idx = 1;
for (final Object v : values) {
res[idx++] = v;
}
return res;
}
public void type(PrimitiveType type) {
this.type = type;
}
public void code(CodeBlock.Builder code) {
this.code = code;
}
public void withLong(final Long value) {
this.value = (T) value;
this.type = PrimitiveType.LONG;
this.code = CodeBlock.builder().add("$Ll", value);
}
public void withLongArray(final Long... values) {
this.value = (T) values;
this.type = PrimitiveType.LONG;
plural = true;
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$Ll", ", ", values.length) + ")",
asArg(Arrays.class, values));
}
public void asInteger() {
type = PrimitiveType.INTEGER;
}
public void withInteger(final Integer value) {
this.value = (T) value;
this.type = PrimitiveType.INTEGER;
this.code = CodeBlock.builder().add("$L", value);
}
public void asDouble() {
type = PrimitiveType.DOUBLE;
}
public void withDouble(final Double value) {
this.value = (T) value;
this.type = PrimitiveType.DOUBLE;
this.code = CodeBlock.builder().add("$Ld", value);
}
public void asFloat() {
type = PrimitiveType.FLOAT;
}
public void withFloat(final Float value) {
this.value = (T) value;
this.type = PrimitiveType.FLOAT;
this.code = CodeBlock.builder().add("$Lf", value);
}
public void asString() {
type = PrimitiveType.STRING;
}
public void withString(final String value) {
this.value = (T) value;
this.type = PrimitiveType.STRING;
this.code = CodeBlock.builder().add("$S", value);
}
public void withBigDecimalArray(final BigDecimal... values) {
this.value = (T) values;
plural = true;
this.type = PrimitiveType.BIG_DECIMAL;
CodeBlock[] blocks = new CodeBlock[values.length];
int i = 0;
for (final BigDecimal value : values) {
blocks[i++] = ofBigDecimal(value).build();
}
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$L", ", ", values.length) + ")",
asArg(Arrays.class, blocks));
}
public void asBigDecimal() {
type = PrimitiveType.BIG_DECIMAL;
}
public void withBigDecimal(final BigDecimal value) {
this.value = (T) value;
this.type = PrimitiveType.BIG_DECIMAL;
this.code = CodeBlock.builder().add("new $T($S)", BigDecimal.class, value.toPlainString());
}
public void asBoolean() {
type = PrimitiveType.BOOLEAN;
}
public void withBoolean(final Boolean value) {
this.value = (T) value;
this.type = PrimitiveType.BOOLEAN;
this.code = CodeBlock.builder().add("$L", value);
}
public void withBigIntegerArray(final BigInteger... values) {
this.value = (T) values;
plural = true;
this.type = PrimitiveType.BIG_INTEGER;
CodeBlock[] blocks = new CodeBlock[values.length];
int i = 0;
for (final BigInteger value : values) {
blocks[i++] = ofBigInteger(value).build();
}
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$L", ",", values.length) + ")",
asArg(Arrays.class, blocks));
}
public void withBigInteger(final BigInteger value) {
this.value = (T) value;
this.type = PrimitiveType.BIG_INTEGER;
this.code = CodeBlock.builder().add("new $T($S)", BigInteger.class, value.toString());
}
public void withLocalDateArray(final LocalDate... values) {
this.value = (T) values;
this.type = PrimitiveType.DATE;
plural = true;
CodeBlock[] blocks = new CodeBlock[values.length];
int i = 0;
for (final LocalDate value : values) {
blocks[i++] = ofLocalDate(value).build();
}
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$L", ", ", values.length) + ")",
asArg(Arrays.class, blocks));
}
public void withLocalDate(final LocalDate value) {
this.value = (T) value;
this.type = PrimitiveType.DATE;
this.code = ofLocalDate(value);
}
public void withLocalDateTimeArray(final LocalDateTime... values) {
this.value = (T) values;
this.type = PrimitiveType.DATE_TIME;
plural = true;
CodeBlock[] blocks = new CodeBlock[values.length];
int i = 0;
for (final LocalDateTime value : values) {
blocks[i++] = ofLocalDateTime(value).build();
}
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$L", ", ", values.length) + ")",
asArg(Arrays.class, blocks));
}
public void withLocalDateTime(final LocalDateTime value) {
this.value = (T) value;
this.type = PrimitiveType.DATE_TIME;
this.code = ofLocalDateTime(value);
}
public void withLocalTimeArray(final LocalTime... values) {
this.value = (T) values;
this.type = PrimitiveType.TIME;
plural = true;
CodeBlock[] blocks = new CodeBlock[values.length];
int i = 0;
for (final LocalTime value : values) {
blocks[i++] = ofLocalTime(value).build();
}
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$L", ", ", values.length) + ")",
asArg(Arrays.class, blocks));
}
public void withLocalTime(final LocalTime value) {
this.value = (T) value;
this.type = PrimitiveType.TIME;
this.code = ofLocalTime(value);
}
public void withUUID(final UUID value) {
this.value = (T) value;
this.type = PrimitiveType.UUID;
this.code = ofUUID(value);
}
public void withUUID(final String value) {
this.value = (T) value;
this.type = PrimitiveType.UUID;
this.code = ofUUID(value);
}
public void withByte(final Byte value) {
this.value = (T) value;
this.type = PrimitiveType.BYTE;
this.code = CodeBlock.builder().add("'$L'", value);
}
public void withShort(final Short value) {
this.value = (T) value;
this.type = PrimitiveType.SHORT;
this.code = CodeBlock.builder().add("$L", value);
}
public void withEnum(final Enum value) {
this.value = (T) value;
this.type = PrimitiveType.EUM;
this.code = CodeBlock.builder().add("$T.$L", ClassName.get(value.getClass()), value.name());
}
public void withGeneric(final T value, final Consumer builder) {
this.value = value;
type = PrimitiveType.CODE;
code = CodeBlock.builder();
builder.accept(code);
}
public void withGenericList(final List values, final Consumer builder) {
this.value = (T) values;
type = PrimitiveType.CODE;
code = CodeBlock.builder();
builder.accept(code);
}
public void withMonth(final Month value) {
this.value = (T) value;
this.type = PrimitiveType.CODE;
this.code = CodeBlock.builder().add("$T.$L", Month.class, value.name());
}
public void withInstant(final Instant value) {
this.value = (T) value;
this.type = PrimitiveType.CODE;
this.code = CodeBlock.builder().add("$T.ofEpochMilli($L)", Instant.class, value.toEpochMilli());
}
public void withStringArray(final String... values) {
this.value = (T) values;
plural = true;
this.type = PrimitiveType.STRING;
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$S", ", ", values.length) + ")",
asArg(Arrays.class, values));
}
public void withIntegerArray(final Integer... values) {
this.value = (T) values;
plural = true;
this.type = PrimitiveType.INTEGER;
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$L", ", ", values.length) + ")",
asArg(Arrays.class, values));
}
public void withDoubleArray(final Double... values) {
this.value = (T) values;
plural = true;
this.type = PrimitiveType.DOUBLE;
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$Ld", ", ", values.length) + ")",
asArg(Arrays.class, values));
}
public void withFloatArray(final Float... values) {
this.value = (T) values;
plural = true;
this.type = PrimitiveType.FLOAT;
code =
CodeBlock.builder()
.add(
"$T.asList(" + StringUtils.repeat("$Lf", ", ", values.length) + ")",
asArg(Arrays.class, values));
}
public boolean isPlural() {
return plural;
}
/**
* get the dynamic code of this property
*
* @return
*/
public CodeBlock.Builder code() {
return code;
}
/**
* Get the primitive type of this property
*
* @return
*/
public PrimitiveType type() {
return type;
}
/**
* get the underling value of this property
*
* @return
*/
public T value() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy