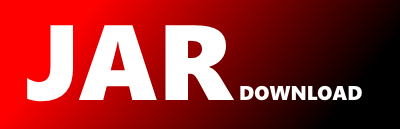
hope.kola.contract.file.FeatureFileScanner Maven / Gradle / Ivy
package hope.kola.contract.file;
import hope.kola.contract.Feature;
import hope.kola.contract.converter.FeatureConverter;
import hope.kola.contract.exception.FeatureParseException;
import java.io.File;
import java.io.IOException;
import java.nio.file.*;
import java.util.*;
import java.util.stream.Stream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/** Scans the provided file path for the DSLs. */
public class FeatureFileScanner {
private static final Logger LOG = LoggerFactory.getLogger(FeatureFileScanner.class);
private final File baseDir;
public FeatureFileScanner(final File baseDir) {
this.baseDir = baseDir;
}
public List findAll() {
final List result = new ArrayList<>();
doFindAll(baseDir, result);
return result;
}
/** We iterate over found features */
private void doFindAll(final File baseDir, final List result) {
try (Stream walk =
Files.walk(baseDir.toPath(), Integer.MAX_VALUE, FileVisitOption.FOLLOW_LINKS)) {
final Path parentPath = baseDir.toPath();
walk.filter(Files::isRegularFile)
.forEach(
path -> {
final String fileName = path.getFileName().toString();
if (fileName.endsWith(".groovy")) {
final File file = path.toFile();
final Collection futures =
FeatureConverter.convertAsCollection(baseDir, file);
if (futures != null && !futures.isEmpty()) {
TestSuiteMetadata featureMetadata = new TestSuiteMetadata(path, futures);
try {
Path relativePath = parentPath.relativize(path.getParent());
String packageName = relativePath.toString().replace(File.separator, ".");
featureMetadata.possiblePkgName(packageName);
} catch (Throwable throwable) {
// Omit
}
result.add(featureMetadata);
}
} else {
if (LOG.isTraceEnabled()) {
LOG.trace("no groovy no process: " + path.toAbsolutePath());
}
}
});
} catch (IOException exception) {
LOG.error("fail find all features from the base dir {}", baseDir.getAbsoluteFile());
throw new FeatureParseException(exception);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy