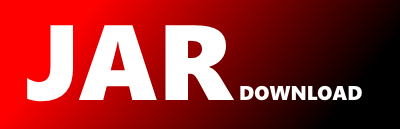
hope.kola.contract.file.Helper Maven / Gradle / Ivy
package hope.kola.contract.file;
import java.io.File;
import java.nio.file.FileSystem;
import java.nio.file.FileSystems;
import java.nio.file.Path;
import java.nio.file.PathMatcher;
import java.util.HashSet;
import java.util.Set;
import java.util.regex.Pattern;
public class Helper {
public static final Pattern SCENARIO_STEP_FILENAME_PATTERN = Pattern.compile("[0-9]+_.*");
public static final String MATCH_PREFIX = "glob:";
public static final String OS_NAME = System.getProperty("os.name");
public static final String OS_NAME_WINDOWS_PREFIX = "Windows";
public static final boolean IS_OS_WINDOWS = getOSMatchesName(OS_NAME_WINDOWS_PREFIX);
public static boolean isFeatureFile(File file) {
return file.isFile() && (file.getName().endsWith(".groovy"));
}
/**
* Decides if the operating system matches.
*
* @param osNamePrefix the prefix for the os name
* @return true if matches, or false if not or can't determine
*/
private static boolean getOSMatchesName(final String osNamePrefix) {
return isOSNameMatch(OS_NAME, osNamePrefix);
}
/**
* Decides if the operating system matches.
*
* This method is package private instead of private to support unit test invocation.
*
* @param osName the actual OS name
* @param osNamePrefix the prefix for the expected OS name
* @return true if matches, or false if not or can't determine
*/
private static boolean isOSNameMatch(final String osName, final String osNamePrefix) {
if (osName == null) {
return false;
}
return osName.startsWith(osNamePrefix);
}
public static boolean matchesPattern(File file, Set matchers) {
for (PathMatcher matcher : matchers) {
if (matcher.matches(file.toPath())) {
return true;
}
}
return false;
}
public static boolean hasScenarioFilenamePattern(Path path) {
return SCENARIO_STEP_FILENAME_PATTERN.matcher(path.getFileName().toString()).matches();
}
public static Set processPatterns(Set patterns) {
FileSystem fileSystem = FileSystems.getDefault();
Set pathMatchers = new HashSet<>();
for (String pattern : patterns) {
String syntaxAndPattern = MATCH_PREFIX + "**" + File.separator + pattern;
// FIXME: This looks strange, need to be checked on windows
if (IS_OS_WINDOWS) {
syntaxAndPattern = syntaxAndPattern.replace("\\", "\\\\");
}
pathMatchers.add(fileSystem.getPathMatcher(syntaxAndPattern));
}
return pathMatchers;
}
}