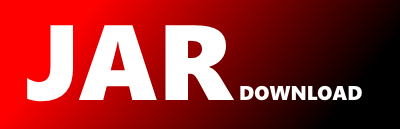
hope.kola.contract.restassured.LogConfigBuilder Maven / Gradle / Ivy
package hope.kola.contract.restassured;
import io.restassured.config.LogConfig;
import io.restassured.filter.log.LogDetail;
import java.util.Set;
import java.util.TreeSet;
public class LogConfigBuilder implements Mergeable {
protected Boolean prettyPrintingEnabled;
protected LogDetail logDetailIfValidationFails;
protected Boolean noLog;
protected Boolean urlEncodeRequestUri;
protected Set headerBlacklist;
public LogConfigBuilder enablePrettyPrinting() {
this.prettyPrintingEnabled = true;
return this;
}
public LogConfigBuilder disablePrettyPrinting() {
this.prettyPrintingEnabled = false;
return this;
}
/** Disable Log even fail */
public LogConfigBuilder disableLogDetailIfValidationFails() {
this.logDetailIfValidationFails = null;
noLog = true;
return this;
}
/** Log only headers */
public LogConfigBuilder logHeadersDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.HEADERS;
noLog = false;
return this;
}
/** Log only cookies */
public LogConfigBuilder logCookiesDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.COOKIES;
noLog = false;
return this;
}
/** Log on the body */
public LogConfigBuilder logBodyDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.BODY;
noLog = false;
return this;
}
/** Log only the status line (works only for responses). */
public LogConfigBuilder logStatusDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.STATUS;
noLog = false;
return this;
}
/** Logs only the request parameters (only works for requests) */
public LogConfigBuilder logParamsDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.PARAMS;
noLog = false;
return this;
}
/** Logs only the request method (only works for requests) */
public LogConfigBuilder logMethodDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.METHOD;
noLog = false;
return this;
}
/** Logs only the request uri (only works for requests) */
public LogConfigBuilder logUriDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.URI;
noLog = false;
return this;
}
/** Logs everything */
public LogConfigBuilder logAllDetailIfValidationFails() {
this.logDetailIfValidationFails = LogDetail.ALL;
noLog = false;
return this;
}
public LogConfigBuilder enableUrlEncodeRequestUri() {
this.urlEncodeRequestUri = true;
noLog = false;
return this;
}
public LogConfigBuilder disableUrlEncodeRequestUri() {
this.urlEncodeRequestUri = false;
noLog = false;
return this;
}
public LogConfigBuilder blackHeader(String hear) {
if (headerBlacklist == null) {
headerBlacklist = new TreeSet<>(String.CASE_INSENSITIVE_ORDER);
}
this.headerBlacklist.add(hear);
return this;
}
/**
* remove all black header list
*
* @return
*/
public LogConfigBuilder noBlackHeader() {
if (headerBlacklist != null) {
headerBlacklist.clear();
}
return this;
}
@Override
public LogConfig transform() {
LogConfig res = new LogConfig();
if (prettyPrintingEnabled != null) {
res = res.enablePrettyPrinting(prettyPrintingEnabled);
}
if (logDetailIfValidationFails != null && !(Boolean.TRUE == noLog)) {
res = res.enableLoggingOfRequestAndResponseIfValidationFails(logDetailIfValidationFails);
}
if (urlEncodeRequestUri != null) {
res = res.urlEncodeRequestUri(urlEncodeRequestUri);
}
if (headerBlacklist != null && !headerBlacklist.isEmpty()) {
res = res.blacklistHeaders(headerBlacklist);
}
return res;
}
@Override
public LogConfigBuilder merger(LogConfigBuilder from) {
LogConfigBuilder res = new LogConfigBuilder();
res.prettyPrintingEnabled = prettyPrintingEnabled;
res.logDetailIfValidationFails = logDetailIfValidationFails;
res.urlEncodeRequestUri = urlEncodeRequestUri;
res.headerBlacklist = new TreeSet<>(String.CASE_INSENSITIVE_ORDER);
res.noLog = noLog;
if (headerBlacklist != null) {
res.headerBlacklist.addAll(headerBlacklist);
}
if (from.prettyPrintingEnabled != null) {
res.prettyPrintingEnabled = from.prettyPrintingEnabled;
}
if (from.noLog != null && from.noLog) {
res.noLog = true;
res.logDetailIfValidationFails = null;
} else {
if (from.logDetailIfValidationFails != null) {
res.logDetailIfValidationFails = from.logDetailIfValidationFails;
}
}
if (from.urlEncodeRequestUri != null) {
res.urlEncodeRequestUri = from.urlEncodeRequestUri;
}
if (from.headerBlacklist != null && !from.headerBlacklist.isEmpty()) {
res.headerBlacklist.addAll(from.headerBlacklist);
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy