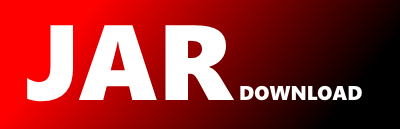
hope.kola.contract.utils.FileUtils Maven / Gradle / Ivy
package hope.kola.contract.utils;
import java.io.*;
import java.nio.charset.StandardCharsets;
public class FileUtils {
public static final File WORKING_DIR = new File("").getAbsoluteFile();
public static byte[] toBytes(String string) {
if (string == null) {
return null;
}
return string.getBytes(StandardCharsets.UTF_8);
}
public static String toString(InputStream is) {
try {
return toByteStream(is).toString(StandardCharsets.UTF_8);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static String toString(byte[] bytes) {
if (bytes == null) {
return null;
}
return new String(bytes, StandardCharsets.UTF_8);
}
public static byte[] toBytes(File file) {
try {
return toBytes(new FileInputStream(file));
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static byte[] toBytes(InputStream is) {
return toByteStream(is).toByteArray();
}
private static ByteArrayOutputStream toByteStream(InputStream is) {
ByteArrayOutputStream result = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int length;
try {
while ((length = is.read(buffer)) != -1) {
result.write(buffer, 0, length);
}
return result;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static void writeToFile(File file, String json) {
writeToFile(file, json.getBytes(StandardCharsets.UTF_8));
}
public static void writeToFile(File file, byte[] data) {
try {
File parent = file.getAbsoluteFile().getParentFile();
if (!parent.exists()) {
parent.mkdirs();
}
// try with resources, so will be closed automatically
try (FileOutputStream fos = new FileOutputStream(file)) {
fos.write(data);
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy