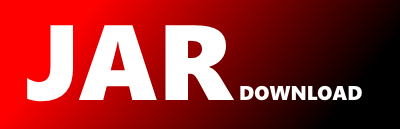
hope.kola.contract.utils.Helper Maven / Gradle / Ivy
package hope.kola.contract.utils;
import java.util.function.Consumer;
import java.util.function.Supplier;
public class Helper {
public static T firstNotNull(T... candidate) {
for (final T c : candidate) {
if (c != null) {
return c;
}
}
return null;
}
public static void firstNotNullAssign(Consumer set, T... candidate) {
for (final T c : candidate) {
if (c != null) {
set.accept(c);
}
}
}
public static void doMerger(Supplier get1, Supplier get2, Consumer set) {
T _new = get2.get();
if (_new != null) {
set.accept(_new);
}
set.accept(get1.get());
}
public static T _get(
final Supplier getter, final Supplier factory, final Consumer setter) {
T target = getter.get();
if (target == null) {
target = factory.get();
setter.accept(target);
}
return target;
}
// There will be a chain call down
// $?.student?.address
public static T _set(
final Supplier getter,
final Supplier factory,
final Consumer setter,
final Consumer assign) {
T target = _get(getter, factory, setter);
assign.accept(target);
return target;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy