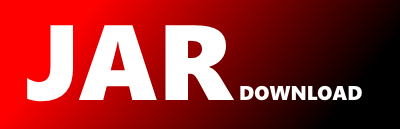
commonMain.com.apollographql.apollo.api.Optional.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-api-jvm Show documentation
Show all versions of apollo-api-jvm Show documentation
Apollo GraphQL API classes
The newest version!
package com.apollographql.apollo.api
import com.apollographql.apollo.annotations.ApolloExperimental
import com.apollographql.apollo.api.Optional.Absent
import com.apollographql.apollo.api.Optional.Present
import com.apollographql.apollo.exception.MissingValueException
import kotlin.jvm.JvmStatic
/**
* A sealed class that can either be [Present] or [Absent]
*
* It provides a convenient way to distinguish the case when a value is provided explicitly and should be
* serialized (even if it's null) and the case where it's absent and shouldn't be serialized.
*/
sealed class Optional {
/**
* Returns the value if this [Optional] is [Present] or null else.
*/
fun getOrNull(): V? = (this as? Present)?.value
/**
* Returns the value if this [Optional] is [Present] or throws [MissingValueException] else.
*/
fun getOrThrow(): V {
if (this is Present) {
return value
}
throw MissingValueException()
}
data class Present(val value: V) : Optional()
object Absent : Optional()
companion object {
@JvmStatic
fun absent(): Absent = Absent
@JvmStatic
fun present(value: V): Present = Present(value)
@JvmStatic
fun presentIfNotNull(value: V?): Optional = if (value == null) Absent else Present(value)
}
}
/**
* Returns the value if this [Optional] is [Present] or fallback else.
*/
fun Optional.getOrElse(fallback: V): V = if (this is Present) this.value else fallback
@ApolloExperimental
fun Optional.map(mapper: (V) -> R): Optional {
return when(this) {
is Absent -> Absent
is Present -> Optional.present(mapper(value))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy