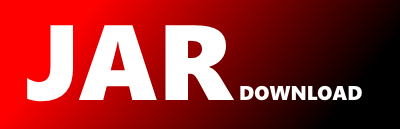
commonMain.com.apollographql.apollo.api.internal.json.ResponseJsonStreamReader.kt Maven / Gradle / Ivy
package com.apollographql.apollo.api.internal.json
import com.apollographql.apollo.api.BigDecimal
import com.apollographql.apollo.api.internal.Throws
import okio.IOException
open class ResponseJsonStreamReader(
private val jsonReader: JsonReader
) {
@Throws(IOException::class)
operator fun hasNext(): Boolean = jsonReader.hasNext()
@Throws(IOException::class)
fun nextName(): String = jsonReader.nextName()
@Throws(IOException::class)
fun skipNext() {
jsonReader.skipValue()
}
@Throws(IOException::class)
fun nextLong(optional: Boolean): Long? {
checkNextValue(optional)
return if (jsonReader.peek() === JsonReader.Token.NULL) {
jsonReader.nextNull()
} else {
jsonReader.nextLong()
}
}
@Throws(IOException::class)
fun nextString(optional: Boolean): String? {
checkNextValue(optional)
return if (jsonReader.peek() === JsonReader.Token.NULL) {
jsonReader.nextNull()
} else {
jsonReader.nextString()
}
}
@Throws(IOException::class)
fun nextBoolean(optional: Boolean): Boolean? {
checkNextValue(optional)
return if (jsonReader.peek() === JsonReader.Token.NULL) {
jsonReader.nextNull()
} else {
jsonReader.nextBoolean()
}
}
@Throws(IOException::class)
fun nextObject(optional: Boolean, objectReader: ObjectReader): T? {
checkNextValue(optional)
return if (jsonReader.peek() === JsonReader.Token.NULL) {
jsonReader.nextNull()
} else {
jsonReader.beginObject()
val result = objectReader.read(this)
jsonReader.endObject()
result
}
}
@Throws(IOException::class)
fun nextList(optional: Boolean, listReader: ListReader): List? {
checkNextValue(optional)
return if (jsonReader.peek() === JsonReader.Token.NULL) {
jsonReader.nextNull()
} else {
jsonReader.beginArray()
val result = ArrayList()
while (jsonReader.hasNext()) {
result.add(listReader.read(this))
}
jsonReader.endArray()
result
}
}
@Throws(IOException::class)
open fun nextScalar(optional: Boolean): Any? {
checkNextValue(optional)
return when {
isNextNull -> skipNext().let { null }
isNextBoolean -> nextBoolean(false)
isNextLong -> BigDecimal(nextLong(false)!!)
isNextNumber -> BigDecimal(nextString(false)!!)
else -> nextString(false)
}
}
@Throws(IOException::class)
fun readObject(): Map? {
return nextObject(false, object : ObjectReader
© 2015 - 2025 Weber Informatics LLC | Privacy Policy